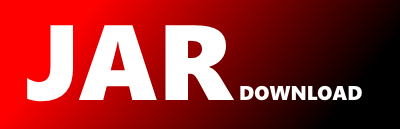
com.pulumi.googlenative.toolresults.v1beta3.kotlin.inputs.ToolExecutionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.toolresults.v1beta3.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.toolresults.v1beta3.inputs.ToolExecutionArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* An execution of an arbitrary tool. It could be a test runner or a tool copying artifacts or deploying code.
* @property commandLineArguments The full tokenized command line including the program name (equivalent to argv in a C program). - In response: present if set by create request - In create request: optional - In update request: never set
* @property exitCode Tool execution exit code. This field will be set once the tool has exited. - In response: present if set by create/update request - In create request: optional - In update request: optional, a FAILED_PRECONDITION error will be returned if an exit_code is already set.
* @property toolLogs References to any plain text logs output the tool execution. This field can be set before the tool has exited in order to be able to have access to a live view of the logs while the tool is running. The maximum allowed number of tool logs per step is 1000. - In response: present if set by create/update request - In create request: optional - In update request: optional, any value provided will be appended to the existing list
* @property toolOutputs References to opaque files of any format output by the tool execution. The maximum allowed number of tool outputs per step is 1000. - In response: present if set by create/update request - In create request: optional - In update request: optional, any value provided will be appended to the existing list
*/
public data class ToolExecutionArgs(
public val commandLineArguments: Output>? = null,
public val exitCode: Output? = null,
public val toolLogs: Output>? = null,
public val toolOutputs: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.toolresults.v1beta3.inputs.ToolExecutionArgs =
com.pulumi.googlenative.toolresults.v1beta3.inputs.ToolExecutionArgs.builder()
.commandLineArguments(commandLineArguments?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.exitCode(exitCode?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.toolLogs(
toolLogs?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.toolOutputs(
toolOutputs?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [ToolExecutionArgs].
*/
@PulumiTagMarker
public class ToolExecutionArgsBuilder internal constructor() {
private var commandLineArguments: Output>? = null
private var exitCode: Output? = null
private var toolLogs: Output>? = null
private var toolOutputs: Output>? = null
/**
* @param value The full tokenized command line including the program name (equivalent to argv in a C program). - In response: present if set by create request - In create request: optional - In update request: never set
*/
@JvmName("opeerkehpgvsyjpy")
public suspend fun commandLineArguments(`value`: Output>) {
this.commandLineArguments = value
}
@JvmName("kbvqauatjoitvvnj")
public suspend fun commandLineArguments(vararg values: Output) {
this.commandLineArguments = Output.all(values.asList())
}
/**
* @param values The full tokenized command line including the program name (equivalent to argv in a C program). - In response: present if set by create request - In create request: optional - In update request: never set
*/
@JvmName("ccutmkdcmtwuepdu")
public suspend fun commandLineArguments(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy