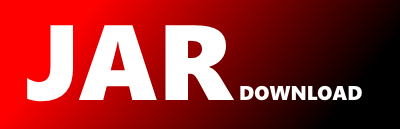
com.pulumi.googlenative.tpu.v2alpha1.kotlin.inputs.QueueingPolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.tpu.v2alpha1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.tpu.v2alpha1.inputs.QueueingPolicyArgs.builder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Defines the policy of the QueuedRequest.
* @property validAfterDuration A relative time after which resources may be created.
* @property validAfterTime An absolute time at which resources may be created.
* @property validInterval An absolute time interval within which resources may be created.
* @property validUntilDuration A relative time after which resources should not be created. If the request cannot be fulfilled by this time the request will be failed.
* @property validUntilTime An absolute time after which resources should not be created. If the request cannot be fulfilled by this time the request will be failed.
*/
public data class QueueingPolicyArgs(
public val validAfterDuration: Output? = null,
public val validAfterTime: Output? = null,
public val validInterval: Output? = null,
public val validUntilDuration: Output? = null,
public val validUntilTime: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.googlenative.tpu.v2alpha1.inputs.QueueingPolicyArgs =
com.pulumi.googlenative.tpu.v2alpha1.inputs.QueueingPolicyArgs.builder()
.validAfterDuration(validAfterDuration?.applyValue({ args0 -> args0 }))
.validAfterTime(validAfterTime?.applyValue({ args0 -> args0 }))
.validInterval(validInterval?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.validUntilDuration(validUntilDuration?.applyValue({ args0 -> args0 }))
.validUntilTime(validUntilTime?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [QueueingPolicyArgs].
*/
@PulumiTagMarker
public class QueueingPolicyArgsBuilder internal constructor() {
private var validAfterDuration: Output? = null
private var validAfterTime: Output? = null
private var validInterval: Output? = null
private var validUntilDuration: Output? = null
private var validUntilTime: Output? = null
/**
* @param value A relative time after which resources may be created.
*/
@JvmName("lhgutcybivkcvuls")
public suspend fun validAfterDuration(`value`: Output) {
this.validAfterDuration = value
}
/**
* @param value An absolute time at which resources may be created.
*/
@JvmName("wtttkewittsgfbxf")
public suspend fun validAfterTime(`value`: Output) {
this.validAfterTime = value
}
/**
* @param value An absolute time interval within which resources may be created.
*/
@JvmName("fupkirmxgorawihd")
public suspend fun validInterval(`value`: Output) {
this.validInterval = value
}
/**
* @param value A relative time after which resources should not be created. If the request cannot be fulfilled by this time the request will be failed.
*/
@JvmName("wcmmmqrppmopihjp")
public suspend fun validUntilDuration(`value`: Output) {
this.validUntilDuration = value
}
/**
* @param value An absolute time after which resources should not be created. If the request cannot be fulfilled by this time the request will be failed.
*/
@JvmName("hnbnmbdnjpixdrbg")
public suspend fun validUntilTime(`value`: Output) {
this.validUntilTime = value
}
/**
* @param value A relative time after which resources may be created.
*/
@JvmName("ovqfjyvftdukusbw")
public suspend fun validAfterDuration(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.validAfterDuration = mapped
}
/**
* @param value An absolute time at which resources may be created.
*/
@JvmName("augmyifvujainhep")
public suspend fun validAfterTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.validAfterTime = mapped
}
/**
* @param value An absolute time interval within which resources may be created.
*/
@JvmName("bjdnsqeewexgutrp")
public suspend fun validInterval(`value`: IntervalArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.validInterval = mapped
}
/**
* @param argument An absolute time interval within which resources may be created.
*/
@JvmName("lsbcengbcxhvcjdl")
public suspend fun validInterval(argument: suspend IntervalArgsBuilder.() -> Unit) {
val toBeMapped = IntervalArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.validInterval = mapped
}
/**
* @param value A relative time after which resources should not be created. If the request cannot be fulfilled by this time the request will be failed.
*/
@JvmName("jsvnmvsjxpjpmfot")
public suspend fun validUntilDuration(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.validUntilDuration = mapped
}
/**
* @param value An absolute time after which resources should not be created. If the request cannot be fulfilled by this time the request will be failed.
*/
@JvmName("turpnlpgleqroyss")
public suspend fun validUntilTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.validUntilTime = mapped
}
internal fun build(): QueueingPolicyArgs = QueueingPolicyArgs(
validAfterDuration = validAfterDuration,
validAfterTime = validAfterTime,
validInterval = validInterval,
validUntilDuration = validUntilDuration,
validUntilTime = validUntilTime,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy