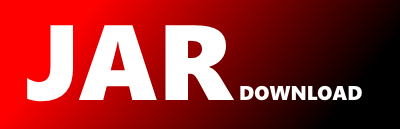
com.pulumi.googlenative.vision.v1.kotlin.ReferenceImage.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.vision.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.googlenative.vision.v1.kotlin.outputs.BoundingPolyResponse
import com.pulumi.googlenative.vision.v1.kotlin.outputs.BoundingPolyResponse.Companion.toKotlin
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
/**
* Builder for [ReferenceImage].
*/
@PulumiTagMarker
public class ReferenceImageResourceBuilder internal constructor() {
public var name: String? = null
public var args: ReferenceImageArgs = ReferenceImageArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ReferenceImageArgsBuilder.() -> Unit) {
val builder = ReferenceImageArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): ReferenceImage {
val builtJavaResource =
com.pulumi.googlenative.vision.v1.ReferenceImage(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return ReferenceImage(builtJavaResource)
}
}
/**
* Creates and returns a new ReferenceImage resource. The `bounding_poly` field is optional. If `bounding_poly` is not specified, the system will try to detect regions of interest in the image that are compatible with the product_category on the parent product. If it is specified, detection is ALWAYS skipped. The system converts polygons into non-rotated rectangles. Note that the pipeline will resize the image if the image resolution is too large to process (above 50MP). Possible errors: * Returns INVALID_ARGUMENT if the image_uri is missing or longer than 4096 characters. * Returns INVALID_ARGUMENT if the product does not exist. * Returns INVALID_ARGUMENT if bounding_poly is not provided, and nothing compatible with the parent product's product_category is detected. * Returns INVALID_ARGUMENT if bounding_poly contains more than 10 polygons.
*/
public class ReferenceImage internal constructor(
override val javaResource: com.pulumi.googlenative.vision.v1.ReferenceImage,
) : KotlinCustomResource(javaResource, ReferenceImageMapper) {
/**
* Optional. Bounding polygons around the areas of interest in the reference image. If this field is empty, the system will try to detect regions of interest. At most 10 bounding polygons will be used. The provided shape is converted into a non-rotated rectangle. Once converted, the small edge of the rectangle must be greater than or equal to 300 pixels. The aspect ratio must be 1:4 or less (i.e. 1:3 is ok; 1:5 is not).
*/
public val boundingPolys: Output>
get() = javaResource.boundingPolys().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> toKotlin(args0) })
})
})
public val location: Output
get() = javaResource.location().applyValue({ args0 -> args0 })
/**
* The resource name of the reference image. Format is: `projects/PROJECT_ID/locations/LOC_ID/products/PRODUCT_ID/referenceImages/IMAGE_ID`. This field is ignored when creating a reference image.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
public val productId: Output
get() = javaResource.productId().applyValue({ args0 -> args0 })
public val project: Output
get() = javaResource.project().applyValue({ args0 -> args0 })
/**
* A user-supplied resource id for the ReferenceImage to be added. If set, the server will attempt to use this value as the resource id. If it is already in use, an error is returned with code ALREADY_EXISTS. Must be at most 128 characters long. It cannot contain the character `/`.
*/
public val referenceImageId: Output?
get() = javaResource.referenceImageId().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The Google Cloud Storage URI of the reference image. The URI must start with `gs://`.
*/
public val uri: Output
get() = javaResource.uri().applyValue({ args0 -> args0 })
}
public object ReferenceImageMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.googlenative.vision.v1.ReferenceImage::class == javaResource::class
override fun map(javaResource: Resource): ReferenceImage = ReferenceImage(
javaResource as
com.pulumi.googlenative.vision.v1.ReferenceImage,
)
}
/**
* @see [ReferenceImage].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [ReferenceImage].
*/
public suspend fun referenceImage(
name: String,
block: suspend ReferenceImageResourceBuilder.() -> Unit,
): ReferenceImage {
val builder = ReferenceImageResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [ReferenceImage].
* @param name The _unique_ name of the resulting resource.
*/
public fun referenceImage(name: String): ReferenceImage {
val builder = ReferenceImageResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy