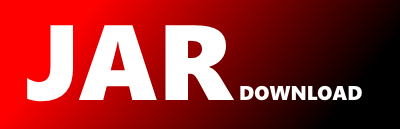
com.pulumi.googlenative.vmmigration.v1.kotlin.SourceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.vmmigration.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.googlenative.vmmigration.v1.SourceArgs.builder
import com.pulumi.googlenative.vmmigration.v1.kotlin.inputs.AwsSourceDetailsArgs
import com.pulumi.googlenative.vmmigration.v1.kotlin.inputs.AwsSourceDetailsArgsBuilder
import com.pulumi.googlenative.vmmigration.v1.kotlin.inputs.VmwareSourceDetailsArgs
import com.pulumi.googlenative.vmmigration.v1.kotlin.inputs.VmwareSourceDetailsArgsBuilder
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Creates a new Source in a given project and location.
* Auto-naming is currently not supported for this resource.
* @property aws AWS type source details.
* @property description User-provided description of the source.
* @property labels The labels of the source.
* @property location
* @property project
* @property requestId A request ID to identify requests. Specify a unique request ID so that if you must retry your request, the server will know to ignore the request if it has already been completed. The server will guarantee that for at least 60 minutes since the first request. For example, consider a situation where you make an initial request and the request times out. If you make the request again with the same request ID, the server can check if original operation with the same request ID was received, and if so, will ignore the second request. This prevents clients from accidentally creating duplicate commitments. The request ID must be a valid UUID with the exception that zero UUID is not supported (00000000-0000-0000-0000-000000000000).
* @property sourceId Required. The source identifier.
* @property vmware Vmware type source details.
*/
public data class SourceArgs(
public val aws: Output? = null,
public val description: Output? = null,
public val labels: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy