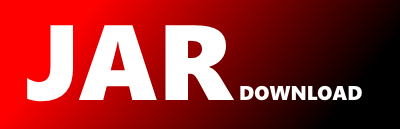
com.pulumi.googlenative.workflowexecutions.v1.kotlin.Execution.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-google-native-kotlin Show documentation
Show all versions of pulumi-google-native-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.googlenative.workflowexecutions.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.googlenative.workflowexecutions.v1.kotlin.outputs.ErrorResponse
import com.pulumi.googlenative.workflowexecutions.v1.kotlin.outputs.StateErrorResponse
import com.pulumi.googlenative.workflowexecutions.v1.kotlin.outputs.StatusResponse
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import com.pulumi.googlenative.workflowexecutions.v1.kotlin.outputs.ErrorResponse.Companion.toKotlin as errorResponseToKotlin
import com.pulumi.googlenative.workflowexecutions.v1.kotlin.outputs.StateErrorResponse.Companion.toKotlin as stateErrorResponseToKotlin
import com.pulumi.googlenative.workflowexecutions.v1.kotlin.outputs.StatusResponse.Companion.toKotlin as statusResponseToKotlin
/**
* Builder for [Execution].
*/
@PulumiTagMarker
public class ExecutionResourceBuilder internal constructor() {
public var name: String? = null
public var args: ExecutionArgs = ExecutionArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ExecutionArgsBuilder.() -> Unit) {
val builder = ExecutionArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Execution {
val builtJavaResource =
com.pulumi.googlenative.workflowexecutions.v1.Execution(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Execution(builtJavaResource)
}
}
/**
* Creates a new execution using the latest revision of the given workflow.
* Auto-naming is currently not supported for this resource.
* Note - this resource's API doesn't support deletion. When deleted, the resource will persist
* on Google Cloud even though it will be deleted from Pulumi state.
*/
public class Execution internal constructor(
override val javaResource: com.pulumi.googlenative.workflowexecutions.v1.Execution,
) : KotlinCustomResource(javaResource, ExecutionMapper) {
/**
* Input parameters of the execution represented as a JSON string. The size limit is 32KB. *Note*: If you are using the REST API directly to run your workflow, you must escape any JSON string value of `argument`. Example: `'{"argument":"{\"firstName\":\"FIRST\",\"lastName\":\"LAST\"}"}'`
*/
public val argument: Output
get() = javaResource.argument().applyValue({ args0 -> args0 })
/**
* The call logging level associated to this execution.
*/
public val callLogLevel: Output
get() = javaResource.callLogLevel().applyValue({ args0 -> args0 })
/**
* Measures the duration of the execution.
*/
public val duration: Output
get() = javaResource.duration().applyValue({ args0 -> args0 })
/**
* Marks the end of execution, successful or not.
*/
public val endTime: Output
get() = javaResource.endTime().applyValue({ args0 -> args0 })
/**
* The error which caused the execution to finish prematurely. The value is only present if the execution's state is `FAILED` or `CANCELLED`.
*/
public val error: Output
get() = javaResource.error().applyValue({ args0 ->
args0.let({ args0 ->
errorResponseToKotlin(args0)
})
})
/**
* Labels associated with this execution. Labels can contain at most 64 entries. Keys and values can be no longer than 63 characters and can only contain lowercase letters, numeric characters, underscores, and dashes. Label keys must start with a letter. International characters are allowed. By default, labels are inherited from the workflow but are overridden by any labels associated with the execution.
*/
public val labels: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy