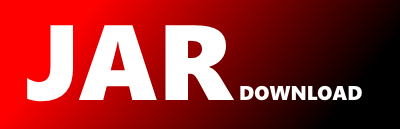
com.pulumi.kubernetes.apiextensions.v1.kotlin.inputs.CustomResourceDefinitionSpecPatchArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.apiextensions.v1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.kubernetes.apiextensions.v1.inputs.CustomResourceDefinitionSpecPatchArgs.builder
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* CustomResourceDefinitionSpec describes how a user wants their resource to appear
* @property conversion conversion defines conversion settings for the CRD.
* @property group group is the API group of the defined custom resource. The custom resources are served under `/apis//...`. Must match the name of the CustomResourceDefinition (in the form `.`).
* @property names names specify the resource and kind names for the custom resource.
* @property preserveUnknownFields preserveUnknownFields indicates that object fields which are not specified in the OpenAPI schema should be preserved when persisting to storage. apiVersion, kind, metadata and known fields inside metadata are always preserved. This field is deprecated in favor of setting `x-preserve-unknown-fields` to true in `spec.versions[*].schema.openAPIV3Schema`. See https://kubernetes.io/docs/tasks/extend-kubernetes/custom-resources/custom-resource-definitions/#field-pruning for details.
* @property scope scope indicates whether the defined custom resource is cluster- or namespace-scoped. Allowed values are `Cluster` and `Namespaced`.
* @property versions versions is the list of all API versions of the defined custom resource. Version names are used to compute the order in which served versions are listed in API discovery. If the version string is "kube-like", it will sort above non "kube-like" version strings, which are ordered lexicographically. "Kube-like" versions start with a "v", then are followed by a number (the major version), then optionally the string "alpha" or "beta" and another number (the minor version). These are sorted first by GA > beta > alpha (where GA is a version with no suffix such as beta or alpha), and then by comparing major version, then minor version. An example sorted list of versions: v10, v2, v1, v11beta2, v10beta3, v3beta1, v12alpha1, v11alpha2, foo1, foo10.
*/
public data class CustomResourceDefinitionSpecPatchArgs(
public val conversion: Output? = null,
public val group: Output? = null,
public val names: Output? = null,
public val preserveUnknownFields: Output? = null,
public val scope: Output? = null,
public val versions: Output>? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.apiextensions.v1.inputs.CustomResourceDefinitionSpecPatchArgs =
com.pulumi.kubernetes.apiextensions.v1.inputs.CustomResourceDefinitionSpecPatchArgs.builder()
.conversion(conversion?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.group(group?.applyValue({ args0 -> args0 }))
.names(names?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.preserveUnknownFields(preserveUnknownFields?.applyValue({ args0 -> args0 }))
.scope(scope?.applyValue({ args0 -> args0 }))
.versions(
versions?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [CustomResourceDefinitionSpecPatchArgs].
*/
@PulumiTagMarker
public class CustomResourceDefinitionSpecPatchArgsBuilder internal constructor() {
private var conversion: Output? = null
private var group: Output? = null
private var names: Output? = null
private var preserveUnknownFields: Output? = null
private var scope: Output? = null
private var versions: Output>? = null
/**
* @param value conversion defines conversion settings for the CRD.
*/
@JvmName("auexfxudrfrnjref")
public suspend fun conversion(`value`: Output) {
this.conversion = value
}
/**
* @param value group is the API group of the defined custom resource. The custom resources are served under `/apis//...`. Must match the name of the CustomResourceDefinition (in the form `.`).
*/
@JvmName("xgbsvywingrjxyap")
public suspend fun group(`value`: Output) {
this.group = value
}
/**
* @param value names specify the resource and kind names for the custom resource.
*/
@JvmName("xryoguqfypejlevg")
public suspend fun names(`value`: Output) {
this.names = value
}
/**
* @param value preserveUnknownFields indicates that object fields which are not specified in the OpenAPI schema should be preserved when persisting to storage. apiVersion, kind, metadata and known fields inside metadata are always preserved. This field is deprecated in favor of setting `x-preserve-unknown-fields` to true in `spec.versions[*].schema.openAPIV3Schema`. See https://kubernetes.io/docs/tasks/extend-kubernetes/custom-resources/custom-resource-definitions/#field-pruning for details.
*/
@JvmName("fauhjtikfapyvtds")
public suspend fun preserveUnknownFields(`value`: Output) {
this.preserveUnknownFields = value
}
/**
* @param value scope indicates whether the defined custom resource is cluster- or namespace-scoped. Allowed values are `Cluster` and `Namespaced`.
*/
@JvmName("nivfmodsxeaufkca")
public suspend fun scope(`value`: Output) {
this.scope = value
}
/**
* @param value versions is the list of all API versions of the defined custom resource. Version names are used to compute the order in which served versions are listed in API discovery. If the version string is "kube-like", it will sort above non "kube-like" version strings, which are ordered lexicographically. "Kube-like" versions start with a "v", then are followed by a number (the major version), then optionally the string "alpha" or "beta" and another number (the minor version). These are sorted first by GA > beta > alpha (where GA is a version with no suffix such as beta or alpha), and then by comparing major version, then minor version. An example sorted list of versions: v10, v2, v1, v11beta2, v10beta3, v3beta1, v12alpha1, v11alpha2, foo1, foo10.
*/
@JvmName("ulwuqjetbkhlkynn")
public suspend fun versions(`value`: Output>) {
this.versions = value
}
@JvmName("wuwttrhqyvtjiysd")
public suspend fun versions(vararg values: Output) {
this.versions = Output.all(values.asList())
}
/**
* @param values versions is the list of all API versions of the defined custom resource. Version names are used to compute the order in which served versions are listed in API discovery. If the version string is "kube-like", it will sort above non "kube-like" version strings, which are ordered lexicographically. "Kube-like" versions start with a "v", then are followed by a number (the major version), then optionally the string "alpha" or "beta" and another number (the minor version). These are sorted first by GA > beta > alpha (where GA is a version with no suffix such as beta or alpha), and then by comparing major version, then minor version. An example sorted list of versions: v10, v2, v1, v11beta2, v10beta3, v3beta1, v12alpha1, v11alpha2, foo1, foo10.
*/
@JvmName("qtugfxqkskbhjrxm")
public suspend fun versions(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy