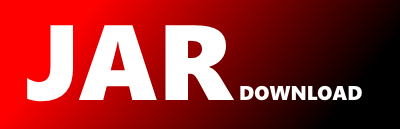
com.pulumi.kubernetes.apiextensions.v1.kotlin.inputs.WebhookConversionPatchArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.apiextensions.v1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.kubernetes.apiextensions.v1.inputs.WebhookConversionPatchArgs.builder
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* WebhookConversion describes how to call a conversion webhook
* @property clientConfig clientConfig is the instructions for how to call the webhook if strategy is `Webhook`.
* @property conversionReviewVersions conversionReviewVersions is an ordered list of preferred `ConversionReview` versions the Webhook expects. The API server will use the first version in the list which it supports. If none of the versions specified in this list are supported by API server, conversion will fail for the custom resource. If a persisted Webhook configuration specifies allowed versions and does not include any versions known to the API Server, calls to the webhook will fail.
*/
public data class WebhookConversionPatchArgs(
public val clientConfig: Output? = null,
public val conversionReviewVersions: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.apiextensions.v1.inputs.WebhookConversionPatchArgs =
com.pulumi.kubernetes.apiextensions.v1.inputs.WebhookConversionPatchArgs.builder()
.clientConfig(clientConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.conversionReviewVersions(
conversionReviewVersions?.applyValue({ args0 ->
args0.map({ args0 ->
args0
})
}),
).build()
}
/**
* Builder for [WebhookConversionPatchArgs].
*/
@PulumiTagMarker
public class WebhookConversionPatchArgsBuilder internal constructor() {
private var clientConfig: Output? = null
private var conversionReviewVersions: Output>? = null
/**
* @param value clientConfig is the instructions for how to call the webhook if strategy is `Webhook`.
*/
@JvmName("dcqxxndkgidhyxku")
public suspend fun clientConfig(`value`: Output) {
this.clientConfig = value
}
/**
* @param value conversionReviewVersions is an ordered list of preferred `ConversionReview` versions the Webhook expects. The API server will use the first version in the list which it supports. If none of the versions specified in this list are supported by API server, conversion will fail for the custom resource. If a persisted Webhook configuration specifies allowed versions and does not include any versions known to the API Server, calls to the webhook will fail.
*/
@JvmName("xrgfgontfneyqvor")
public suspend fun conversionReviewVersions(`value`: Output>) {
this.conversionReviewVersions = value
}
@JvmName("sdpodjycnquprlre")
public suspend fun conversionReviewVersions(vararg values: Output) {
this.conversionReviewVersions = Output.all(values.asList())
}
/**
* @param values conversionReviewVersions is an ordered list of preferred `ConversionReview` versions the Webhook expects. The API server will use the first version in the list which it supports. If none of the versions specified in this list are supported by API server, conversion will fail for the custom resource. If a persisted Webhook configuration specifies allowed versions and does not include any versions known to the API Server, calls to the webhook will fail.
*/
@JvmName("mafokvykosikyhgj")
public suspend fun conversionReviewVersions(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy