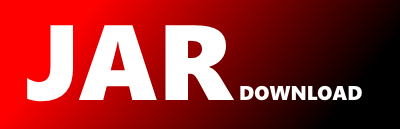
com.pulumi.kubernetes.apps.v1.kotlin.Deployment.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.apps.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.kubernetes.apps.v1.kotlin.outputs.DeploymentSpec
import com.pulumi.kubernetes.apps.v1.kotlin.outputs.DeploymentStatus
import com.pulumi.kubernetes.meta.v1.kotlin.outputs.ObjectMeta
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.kubernetes.apps.v1.kotlin.outputs.DeploymentSpec.Companion.toKotlin as deploymentSpecToKotlin
import com.pulumi.kubernetes.apps.v1.kotlin.outputs.DeploymentStatus.Companion.toKotlin as deploymentStatusToKotlin
import com.pulumi.kubernetes.meta.v1.kotlin.outputs.ObjectMeta.Companion.toKotlin as objectMetaToKotlin
/**
* Builder for [Deployment].
*/
@PulumiTagMarker
public class DeploymentResourceBuilder internal constructor() {
public var name: String? = null
public var args: DeploymentArgs = DeploymentArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend DeploymentArgsBuilder.() -> Unit) {
val builder = DeploymentArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Deployment {
val builtJavaResource = com.pulumi.kubernetes.apps.v1.Deployment(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Deployment(builtJavaResource)
}
}
/**
* Deployment enables declarative updates for Pods and ReplicaSets.
* This resource waits until its status is ready before registering success
* for create/update, and populating output properties from the current state of the resource.
* The following conditions are used to determine whether the resource creation has
* succeeded or failed:
* 1. The Deployment has begun to be updated by the Deployment controller. If the current
* generation of the Deployment is > 1, then this means that the current generation must
* be different from the generation reported by the last outputs.
* 2. There exists a ReplicaSet whose revision is equal to the current revision of the
* Deployment.
* 3. The Deployment's '.status.conditions' has a status of type 'Available' whose 'status'
* member is set to 'True'.
* 4. If the Deployment has generation > 1, then '.status.conditions' has a status of type
* 'Progressing', whose 'status' member is set to 'True', and whose 'reason' is
* 'NewReplicaSetAvailable'. For generation <= 1, this status field does not exist,
* because it doesn't do a rollout (i.e., it simply creates the Deployment and
* corresponding ReplicaSet), and therefore there is no rollout to mark as 'Progressing'.
* If the Deployment has not reached a Ready state after 10 minutes, it will
* time out and mark the resource update as Failed. You can override the default timeout value
* by setting the 'customTimeouts' option on the resource.
* ## Example Usage
* ### Create a Deployment with auto-naming
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.kubernetes.apps_v1.Deployment;
* import com.pulumi.kubernetes.apps_v1.DeploymentArgs;
* import com.pulumi.kubernetes.meta_v1.inputs.ObjectMetaArgs;
* import com.pulumi.kubernetes.apps_v1.inputs.DeploymentSpecArgs;
* import com.pulumi.kubernetes.meta_v1.inputs.LabelSelectorArgs;
* import com.pulumi.kubernetes.core_v1.inputs.PodTemplateSpecArgs;
* import com.pulumi.kubernetes.core_v1.inputs.PodSpecArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var deployment = new Deployment("deployment", DeploymentArgs.builder()
* .metadata(ObjectMetaArgs.builder()
* .labels(Map.of("app", "nginx"))
* .build())
* .spec(DeploymentSpecArgs.builder()
* .replicas(3)
* .selector(LabelSelectorArgs.builder()
* .matchLabels(Map.of("app", "nginx"))
* .build())
* .template(PodTemplateSpecArgs.builder()
* .metadata(ObjectMetaArgs.builder()
* .labels(Map.of("app", "nginx"))
* .build())
* .spec(PodSpecArgs.builder()
* .containers(ContainerArgs.builder()
* .image("nginx:1.14.2")
* .name("nginx")
* .ports(ContainerPortArgs.builder()
* .containerPort(80)
* .build())
* .build())
* .build())
* .build())
* .build())
* .build());
* }
* }
* ```
* ### Create a Deployment with a user-specified name
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.kubernetes.apps_v1.Deployment;
* import com.pulumi.kubernetes.apps_v1.DeploymentArgs;
* import com.pulumi.kubernetes.meta_v1.inputs.ObjectMetaArgs;
* import com.pulumi.kubernetes.apps_v1.inputs.DeploymentSpecArgs;
* import com.pulumi.kubernetes.meta_v1.inputs.LabelSelectorArgs;
* import com.pulumi.kubernetes.core_v1.inputs.PodTemplateSpecArgs;
* import com.pulumi.kubernetes.core_v1.inputs.PodSpecArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var deployment = new Deployment("deployment", DeploymentArgs.builder()
* .metadata(ObjectMetaArgs.builder()
* .labels(Map.of("app", "nginx"))
* .name("nginx-deployment")
* .build())
* .spec(DeploymentSpecArgs.builder()
* .replicas(3)
* .selector(LabelSelectorArgs.builder()
* .matchLabels(Map.of("app", "nginx"))
* .build())
* .template(PodTemplateSpecArgs.builder()
* .metadata(ObjectMetaArgs.builder()
* .labels(Map.of("app", "nginx"))
* .build())
* .spec(PodSpecArgs.builder()
* .containers(ContainerArgs.builder()
* .image("nginx:1.14.2")
* .name("nginx")
* .ports(ContainerPortArgs.builder()
* .containerPort(80)
* .build())
* .build())
* .build())
* .build())
* .build())
* .build());
* }
* }
* ```
*/
public class Deployment internal constructor(
override val javaResource: com.pulumi.kubernetes.apps.v1.Deployment,
) : KotlinCustomResource(javaResource, DeploymentMapper) {
/**
* APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*/
public val apiVersion: Output
get() = javaResource.apiVersion().applyValue({ args0 -> args0 })
/**
* Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
public val kind: Output
get() = javaResource.kind().applyValue({ args0 -> args0 })
/**
* Standard object's metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
*/
public val metadata: Output
get() = javaResource.metadata().applyValue({ args0 ->
args0.let({ args0 ->
objectMetaToKotlin(args0)
})
})
/**
* Specification of the desired behavior of the Deployment.
*/
public val spec: Output
get() = javaResource.spec().applyValue({ args0 ->
args0.let({ args0 ->
deploymentSpecToKotlin(args0)
})
})
/**
* Most recently observed status of the Deployment.
*/
public val status: Output?
get() = javaResource.status().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
deploymentStatusToKotlin(args0)
})
}).orElse(null)
})
}
public object DeploymentMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.kubernetes.apps.v1.Deployment::class == javaResource::class
override fun map(javaResource: Resource): Deployment = Deployment(
javaResource as
com.pulumi.kubernetes.apps.v1.Deployment,
)
}
/**
* @see [Deployment].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Deployment].
*/
public suspend fun deployment(name: String, block: suspend DeploymentResourceBuilder.() -> Unit): Deployment {
val builder = DeploymentResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Deployment].
* @param name The _unique_ name of the resulting resource.
*/
public fun deployment(name: String): Deployment {
val builder = DeploymentResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy