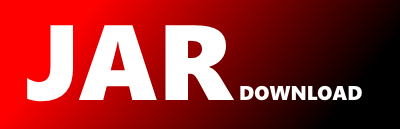
com.pulumi.kubernetes.apps.v1.kotlin.inputs.RollingUpdateStatefulSetStrategyPatchArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.apps.v1.kotlin.inputs
import com.pulumi.core.Either
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kubernetes.apps.v1.inputs.RollingUpdateStatefulSetStrategyPatchArgs.builder
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* RollingUpdateStatefulSetStrategy is used to communicate parameter for RollingUpdateStatefulSetStrategyType.
* @property maxUnavailable The maximum number of pods that can be unavailable during the update. Value can be an absolute number (ex: 5) or a percentage of desired pods (ex: 10%). Absolute number is calculated from percentage by rounding up. This can not be 0. Defaults to 1. This field is alpha-level and is only honored by servers that enable the MaxUnavailableStatefulSet feature. The field applies to all pods in the range 0 to Replicas-1. That means if there is any unavailable pod in the range 0 to Replicas-1, it will be counted towards MaxUnavailable.
* @property partition Partition indicates the ordinal at which the StatefulSet should be partitioned for updates. During a rolling update, all pods from ordinal Replicas-1 to Partition are updated. All pods from ordinal Partition-1 to 0 remain untouched. This is helpful in being able to do a canary based deployment. The default value is 0.
*/
public data class RollingUpdateStatefulSetStrategyPatchArgs(
public val maxUnavailable: Output>? = null,
public val partition: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.apps.v1.inputs.RollingUpdateStatefulSetStrategyPatchArgs =
com.pulumi.kubernetes.apps.v1.inputs.RollingUpdateStatefulSetStrategyPatchArgs.builder()
.maxUnavailable(
maxUnavailable?.applyValue({ args0 ->
args0.transform({ args0 -> args0 }, { args0 ->
args0
})
}),
)
.partition(partition?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [RollingUpdateStatefulSetStrategyPatchArgs].
*/
@PulumiTagMarker
public class RollingUpdateStatefulSetStrategyPatchArgsBuilder internal constructor() {
private var maxUnavailable: Output>? = null
private var partition: Output? = null
/**
* @param value The maximum number of pods that can be unavailable during the update. Value can be an absolute number (ex: 5) or a percentage of desired pods (ex: 10%). Absolute number is calculated from percentage by rounding up. This can not be 0. Defaults to 1. This field is alpha-level and is only honored by servers that enable the MaxUnavailableStatefulSet feature. The field applies to all pods in the range 0 to Replicas-1. That means if there is any unavailable pod in the range 0 to Replicas-1, it will be counted towards MaxUnavailable.
*/
@JvmName("nbqcpeckawifsost")
public suspend fun maxUnavailable(`value`: Output>) {
this.maxUnavailable = value
}
/**
* @param value Partition indicates the ordinal at which the StatefulSet should be partitioned for updates. During a rolling update, all pods from ordinal Replicas-1 to Partition are updated. All pods from ordinal Partition-1 to 0 remain untouched. This is helpful in being able to do a canary based deployment. The default value is 0.
*/
@JvmName("xeovsoofdwydkobq")
public suspend fun partition(`value`: Output) {
this.partition = value
}
/**
* @param value The maximum number of pods that can be unavailable during the update. Value can be an absolute number (ex: 5) or a percentage of desired pods (ex: 10%). Absolute number is calculated from percentage by rounding up. This can not be 0. Defaults to 1. This field is alpha-level and is only honored by servers that enable the MaxUnavailableStatefulSet feature. The field applies to all pods in the range 0 to Replicas-1. That means if there is any unavailable pod in the range 0 to Replicas-1, it will be counted towards MaxUnavailable.
*/
@JvmName("orhfakorayynengo")
public suspend fun maxUnavailable(`value`: Either?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.maxUnavailable = mapped
}
/**
* @param value The maximum number of pods that can be unavailable during the update. Value can be an absolute number (ex: 5) or a percentage of desired pods (ex: 10%). Absolute number is calculated from percentage by rounding up. This can not be 0. Defaults to 1. This field is alpha-level and is only honored by servers that enable the MaxUnavailableStatefulSet feature. The field applies to all pods in the range 0 to Replicas-1. That means if there is any unavailable pod in the range 0 to Replicas-1, it will be counted towards MaxUnavailable.
*/
@JvmName("ogpsjlndhbaawmvk")
public fun maxUnavailable(`value`: Int) {
val toBeMapped = Either.ofLeft(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.maxUnavailable = mapped
}
/**
* @param value The maximum number of pods that can be unavailable during the update. Value can be an absolute number (ex: 5) or a percentage of desired pods (ex: 10%). Absolute number is calculated from percentage by rounding up. This can not be 0. Defaults to 1. This field is alpha-level and is only honored by servers that enable the MaxUnavailableStatefulSet feature. The field applies to all pods in the range 0 to Replicas-1. That means if there is any unavailable pod in the range 0 to Replicas-1, it will be counted towards MaxUnavailable.
*/
@JvmName("feuqfvbpddwrsmkt")
public fun maxUnavailable(`value`: String) {
val toBeMapped = Either.ofRight(value)
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.maxUnavailable = mapped
}
/**
* @param value Partition indicates the ordinal at which the StatefulSet should be partitioned for updates. During a rolling update, all pods from ordinal Replicas-1 to Partition are updated. All pods from ordinal Partition-1 to 0 remain untouched. This is helpful in being able to do a canary based deployment. The default value is 0.
*/
@JvmName("lohujifmdwvtpuir")
public suspend fun partition(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.partition = mapped
}
internal fun build(): RollingUpdateStatefulSetStrategyPatchArgs =
RollingUpdateStatefulSetStrategyPatchArgs(
maxUnavailable = maxUnavailable,
partition = partition,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy