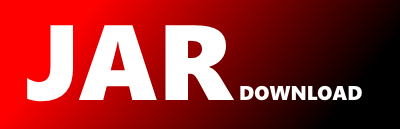
com.pulumi.kubernetes.apps.v1beta1.kotlin.inputs.ControllerRevisionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.apps.v1beta1.kotlin.inputs
import com.google.gson.JsonParser
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.kubernetes.apps.v1beta1.inputs.ControllerRevisionArgs.builder
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.ObjectMetaArgs
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.ObjectMetaArgsBuilder
import kotlinx.serialization.json.Json
import kotlinx.serialization.json.JsonElement
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* ControllerRevision implements an immutable snapshot of state data. Clients are responsible for serializing and deserializing the objects that contain their internal state. Once a ControllerRevision has been successfully created, it can not be updated. The API Server will fail validation of all requests that attempt to mutate the Data field. ControllerRevisions may, however, be deleted. Note that, due to its use by both the DaemonSet and StatefulSet controllers for update and rollback, this object is beta. However, it may be subject to name and representation changes in future releases, and clients should not depend on its stability. It is primarily for internal use by controllers.
* @property apiVersion APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
* @property data Data is the serialized representation of the state.
* @property kind Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
* @property metadata Standard object's metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
* @property revision Revision indicates the revision of the state represented by Data.
*/
public data class ControllerRevisionArgs(
public val apiVersion: Output? = null,
public val `data`: Output? = null,
public val kind: Output? = null,
public val metadata: Output? = null,
public val revision: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.apps.v1beta1.inputs.ControllerRevisionArgs =
com.pulumi.kubernetes.apps.v1beta1.inputs.ControllerRevisionArgs.builder()
.apiVersion(apiVersion?.applyValue({ args0 -> args0 }))
.`data`(
`data`?.applyValue({ args0 ->
JsonParser.parseString(Json.encodeToString(JsonElement.serializer(), args0))
}),
)
.kind(kind?.applyValue({ args0 -> args0 }))
.metadata(metadata?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.revision(revision.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ControllerRevisionArgs].
*/
@PulumiTagMarker
public class ControllerRevisionArgsBuilder internal constructor() {
private var apiVersion: Output? = null
private var `data`: Output? = null
private var kind: Output? = null
private var metadata: Output? = null
private var revision: Output? = null
/**
* @param value APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*/
@JvmName("ankhawfbijoldorv")
public suspend fun apiVersion(`value`: Output) {
this.apiVersion = value
}
/**
* @param value Data is the serialized representation of the state.
*/
@JvmName("apqnxixajyjgsvae")
public suspend fun `data`(`value`: Output) {
this.`data` = value
}
/**
* @param value Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
@JvmName("wfpyoancqwbhwgyr")
public suspend fun kind(`value`: Output) {
this.kind = value
}
/**
* @param value Standard object's metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
*/
@JvmName("yiqxqylaeqnemddv")
public suspend fun metadata(`value`: Output) {
this.metadata = value
}
/**
* @param value Revision indicates the revision of the state represented by Data.
*/
@JvmName("eomiyvscbcppovfo")
public suspend fun revision(`value`: Output) {
this.revision = value
}
/**
* @param value APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*/
@JvmName("ioyqtdmqmfkccatn")
public suspend fun apiVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiVersion = mapped
}
/**
* @param value Data is the serialized representation of the state.
*/
@JvmName("hplcnofbnaxhnrbu")
public suspend fun `data`(`value`: JsonElement?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.`data` = mapped
}
/**
* @param value Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
@JvmName("eokiwbkfijcxnjse")
public suspend fun kind(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kind = mapped
}
/**
* @param value Standard object's metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
*/
@JvmName("kjdpltowplskurkw")
public suspend fun metadata(`value`: ObjectMetaArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.metadata = mapped
}
/**
* @param argument Standard object's metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
*/
@JvmName("lmyymkptrfwfbbvr")
public suspend fun metadata(argument: suspend ObjectMetaArgsBuilder.() -> Unit) {
val toBeMapped = ObjectMetaArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.metadata = mapped
}
/**
* @param value Revision indicates the revision of the state represented by Data.
*/
@JvmName("ajpelxolbnurftas")
public suspend fun revision(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.revision = mapped
}
internal fun build(): ControllerRevisionArgs = ControllerRevisionArgs(
apiVersion = apiVersion,
`data` = `data`,
kind = kind,
metadata = metadata,
revision = revision ?: throw PulumiNullFieldException("revision"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy