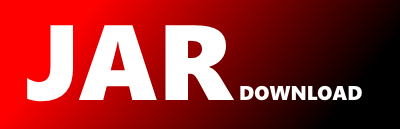
com.pulumi.kubernetes.apps.v1beta1.kotlin.inputs.DeploymentSpecArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.apps.v1beta1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.kubernetes.apps.v1beta1.inputs.DeploymentSpecArgs.builder
import com.pulumi.kubernetes.core.v1.kotlin.inputs.PodTemplateSpecArgs
import com.pulumi.kubernetes.core.v1.kotlin.inputs.PodTemplateSpecArgsBuilder
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.LabelSelectorArgs
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.LabelSelectorArgsBuilder
import kotlin.Boolean
import kotlin.Int
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* DeploymentSpec is the specification of the desired behavior of the Deployment.
* @property minReadySeconds Minimum number of seconds for which a newly created pod should be ready without any of its container crashing, for it to be considered available. Defaults to 0 (pod will be considered available as soon as it is ready)
* @property paused Indicates that the deployment is paused.
* @property progressDeadlineSeconds The maximum time in seconds for a deployment to make progress before it is considered to be failed. The deployment controller will continue to process failed deployments and a condition with a ProgressDeadlineExceeded reason will be surfaced in the deployment status. Note that progress will not be estimated during the time a deployment is paused. Defaults to 600s.
* @property replicas Number of desired pods. This is a pointer to distinguish between explicit zero and not specified. Defaults to 1.
* @property revisionHistoryLimit The number of old ReplicaSets to retain to allow rollback. This is a pointer to distinguish between explicit zero and not specified. Defaults to 2.
* @property rollbackTo DEPRECATED. The config this deployment is rolling back to. Will be cleared after rollback is done.
* @property selector Label selector for pods. Existing ReplicaSets whose pods are selected by this will be the ones affected by this deployment.
* @property strategy The deployment strategy to use to replace existing pods with new ones.
* @property template Template describes the pods that will be created.
*/
public data class DeploymentSpecArgs(
public val minReadySeconds: Output? = null,
public val paused: Output? = null,
public val progressDeadlineSeconds: Output? = null,
public val replicas: Output? = null,
public val revisionHistoryLimit: Output? = null,
public val rollbackTo: Output? = null,
public val selector: Output? = null,
public val strategy: Output? = null,
public val template: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.apps.v1beta1.inputs.DeploymentSpecArgs =
com.pulumi.kubernetes.apps.v1beta1.inputs.DeploymentSpecArgs.builder()
.minReadySeconds(minReadySeconds?.applyValue({ args0 -> args0 }))
.paused(paused?.applyValue({ args0 -> args0 }))
.progressDeadlineSeconds(progressDeadlineSeconds?.applyValue({ args0 -> args0 }))
.replicas(replicas?.applyValue({ args0 -> args0 }))
.revisionHistoryLimit(revisionHistoryLimit?.applyValue({ args0 -> args0 }))
.rollbackTo(rollbackTo?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.selector(selector?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.strategy(strategy?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.template(template.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [DeploymentSpecArgs].
*/
@PulumiTagMarker
public class DeploymentSpecArgsBuilder internal constructor() {
private var minReadySeconds: Output? = null
private var paused: Output? = null
private var progressDeadlineSeconds: Output? = null
private var replicas: Output? = null
private var revisionHistoryLimit: Output? = null
private var rollbackTo: Output? = null
private var selector: Output? = null
private var strategy: Output? = null
private var template: Output? = null
/**
* @param value Minimum number of seconds for which a newly created pod should be ready without any of its container crashing, for it to be considered available. Defaults to 0 (pod will be considered available as soon as it is ready)
*/
@JvmName("cdcicmgkyqarwsmx")
public suspend fun minReadySeconds(`value`: Output) {
this.minReadySeconds = value
}
/**
* @param value Indicates that the deployment is paused.
*/
@JvmName("qrlpfjqtpjlfxwwi")
public suspend fun paused(`value`: Output) {
this.paused = value
}
/**
* @param value The maximum time in seconds for a deployment to make progress before it is considered to be failed. The deployment controller will continue to process failed deployments and a condition with a ProgressDeadlineExceeded reason will be surfaced in the deployment status. Note that progress will not be estimated during the time a deployment is paused. Defaults to 600s.
*/
@JvmName("axdvlmrkffujigqq")
public suspend fun progressDeadlineSeconds(`value`: Output) {
this.progressDeadlineSeconds = value
}
/**
* @param value Number of desired pods. This is a pointer to distinguish between explicit zero and not specified. Defaults to 1.
*/
@JvmName("qrordwfdkqbvvjnp")
public suspend fun replicas(`value`: Output) {
this.replicas = value
}
/**
* @param value The number of old ReplicaSets to retain to allow rollback. This is a pointer to distinguish between explicit zero and not specified. Defaults to 2.
*/
@JvmName("lcufbkvmkfgsevqv")
public suspend fun revisionHistoryLimit(`value`: Output) {
this.revisionHistoryLimit = value
}
/**
* @param value DEPRECATED. The config this deployment is rolling back to. Will be cleared after rollback is done.
*/
@JvmName("cbrditwbxybdbbab")
public suspend fun rollbackTo(`value`: Output) {
this.rollbackTo = value
}
/**
* @param value Label selector for pods. Existing ReplicaSets whose pods are selected by this will be the ones affected by this deployment.
*/
@JvmName("yxbrhegcfqevlnqo")
public suspend fun selector(`value`: Output) {
this.selector = value
}
/**
* @param value The deployment strategy to use to replace existing pods with new ones.
*/
@JvmName("grsonbotxdttmhlv")
public suspend fun strategy(`value`: Output) {
this.strategy = value
}
/**
* @param value Template describes the pods that will be created.
*/
@JvmName("vuimowrfwrcucgjf")
public suspend fun template(`value`: Output) {
this.template = value
}
/**
* @param value Minimum number of seconds for which a newly created pod should be ready without any of its container crashing, for it to be considered available. Defaults to 0 (pod will be considered available as soon as it is ready)
*/
@JvmName("aewqaormrsoqqeok")
public suspend fun minReadySeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.minReadySeconds = mapped
}
/**
* @param value Indicates that the deployment is paused.
*/
@JvmName("yxjstefyyopfropp")
public suspend fun paused(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.paused = mapped
}
/**
* @param value The maximum time in seconds for a deployment to make progress before it is considered to be failed. The deployment controller will continue to process failed deployments and a condition with a ProgressDeadlineExceeded reason will be surfaced in the deployment status. Note that progress will not be estimated during the time a deployment is paused. Defaults to 600s.
*/
@JvmName("umeyeccarfshugbf")
public suspend fun progressDeadlineSeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.progressDeadlineSeconds = mapped
}
/**
* @param value Number of desired pods. This is a pointer to distinguish between explicit zero and not specified. Defaults to 1.
*/
@JvmName("jjbxkdyiilpnshrl")
public suspend fun replicas(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.replicas = mapped
}
/**
* @param value The number of old ReplicaSets to retain to allow rollback. This is a pointer to distinguish between explicit zero and not specified. Defaults to 2.
*/
@JvmName("qyvfiaaullixpryj")
public suspend fun revisionHistoryLimit(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.revisionHistoryLimit = mapped
}
/**
* @param value DEPRECATED. The config this deployment is rolling back to. Will be cleared after rollback is done.
*/
@JvmName("swqvhoagcdillulw")
public suspend fun rollbackTo(`value`: RollbackConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rollbackTo = mapped
}
/**
* @param argument DEPRECATED. The config this deployment is rolling back to. Will be cleared after rollback is done.
*/
@JvmName("renfohbdxlsgeofn")
public suspend fun rollbackTo(argument: suspend RollbackConfigArgsBuilder.() -> Unit) {
val toBeMapped = RollbackConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.rollbackTo = mapped
}
/**
* @param value Label selector for pods. Existing ReplicaSets whose pods are selected by this will be the ones affected by this deployment.
*/
@JvmName("coaqqernldwrolan")
public suspend fun selector(`value`: LabelSelectorArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.selector = mapped
}
/**
* @param argument Label selector for pods. Existing ReplicaSets whose pods are selected by this will be the ones affected by this deployment.
*/
@JvmName("baftrxudplyljkpe")
public suspend fun selector(argument: suspend LabelSelectorArgsBuilder.() -> Unit) {
val toBeMapped = LabelSelectorArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.selector = mapped
}
/**
* @param value The deployment strategy to use to replace existing pods with new ones.
*/
@JvmName("ighnctdvhpkfsfsb")
public suspend fun strategy(`value`: DeploymentStrategyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.strategy = mapped
}
/**
* @param argument The deployment strategy to use to replace existing pods with new ones.
*/
@JvmName("hgsaunaulvbauptg")
public suspend fun strategy(argument: suspend DeploymentStrategyArgsBuilder.() -> Unit) {
val toBeMapped = DeploymentStrategyArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.strategy = mapped
}
/**
* @param value Template describes the pods that will be created.
*/
@JvmName("yntxtoldxsupnxvx")
public suspend fun template(`value`: PodTemplateSpecArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.template = mapped
}
/**
* @param argument Template describes the pods that will be created.
*/
@JvmName("cuuayepfrophrhsa")
public suspend fun template(argument: suspend PodTemplateSpecArgsBuilder.() -> Unit) {
val toBeMapped = PodTemplateSpecArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.template = mapped
}
internal fun build(): DeploymentSpecArgs = DeploymentSpecArgs(
minReadySeconds = minReadySeconds,
paused = paused,
progressDeadlineSeconds = progressDeadlineSeconds,
replicas = replicas,
revisionHistoryLimit = revisionHistoryLimit,
rollbackTo = rollbackTo,
selector = selector,
strategy = strategy,
template = template ?: throw PulumiNullFieldException("template"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy