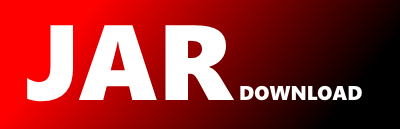
com.pulumi.kubernetes.certificates.v1alpha1.kotlin.ClusterTrustBundlePatch.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.certificates.v1alpha1.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.kubernetes.certificates.v1alpha1.kotlin.outputs.ClusterTrustBundleSpecPatch
import com.pulumi.kubernetes.meta.v1.kotlin.outputs.ObjectMetaPatch
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.kubernetes.certificates.v1alpha1.kotlin.outputs.ClusterTrustBundleSpecPatch.Companion.toKotlin as clusterTrustBundleSpecPatchToKotlin
import com.pulumi.kubernetes.meta.v1.kotlin.outputs.ObjectMetaPatch.Companion.toKotlin as objectMetaPatchToKotlin
/**
* Builder for [ClusterTrustBundlePatch].
*/
@PulumiTagMarker
public class ClusterTrustBundlePatchResourceBuilder internal constructor() {
public var name: String? = null
public var args: ClusterTrustBundlePatchArgs = ClusterTrustBundlePatchArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ClusterTrustBundlePatchArgsBuilder.() -> Unit) {
val builder = ClusterTrustBundlePatchArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): ClusterTrustBundlePatch {
val builtJavaResource =
com.pulumi.kubernetes.certificates.v1alpha1.ClusterTrustBundlePatch(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return ClusterTrustBundlePatch(builtJavaResource)
}
}
/**
* Patch resources are used to modify existing Kubernetes resources by using
* Server-Side Apply updates. The name of the resource must be specified, but all other properties are optional. More than
* one patch may be applied to the same resource, and a random FieldManager name will be used for each Patch resource.
* Conflicts will result in an error by default, but can be forced using the "pulumi.com/patchForce" annotation. See the
* [Server-Side Apply Docs](https://www.pulumi.com/registry/packages/kubernetes/how-to-guides/managing-resources-with-server-side-apply/) for
* additional information about using Server-Side Apply to manage Kubernetes resources with Pulumi.
* ClusterTrustBundle is a cluster-scoped container for X.509 trust anchors (root certificates).
* ClusterTrustBundle objects are considered to be readable by any authenticated user in the cluster, because they can be mounted by pods using the `clusterTrustBundle` projection. All service accounts have read access to ClusterTrustBundles by default. Users who only have namespace-level access to a cluster can read ClusterTrustBundles by impersonating a serviceaccount that they have access to.
* It can be optionally associated with a particular assigner, in which case it contains one valid set of trust anchors for that signer. Signers may have multiple associated ClusterTrustBundles; each is an independent set of trust anchors for that signer. Admission control is used to enforce that only users with permissions on the signer can create or modify the corresponding bundle.
*/
public class ClusterTrustBundlePatch internal constructor(
override val javaResource: com.pulumi.kubernetes.certificates.v1alpha1.ClusterTrustBundlePatch,
) : KotlinCustomResource(javaResource, ClusterTrustBundlePatchMapper) {
/**
* APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*/
public val apiVersion: Output?
get() = javaResource.apiVersion().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
public val kind: Output?
get() = javaResource.kind().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* metadata contains the object metadata.
*/
public val metadata: Output?
get() = javaResource.metadata().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
objectMetaPatchToKotlin(args0)
})
}).orElse(null)
})
/**
* spec contains the signer (if any) and trust anchors.
*/
public val spec: Output?
get() = javaResource.spec().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
clusterTrustBundleSpecPatchToKotlin(args0)
})
}).orElse(null)
})
}
public object ClusterTrustBundlePatchMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.kubernetes.certificates.v1alpha1.ClusterTrustBundlePatch::class == javaResource::class
override fun map(javaResource: Resource): ClusterTrustBundlePatch =
ClusterTrustBundlePatch(
javaResource as
com.pulumi.kubernetes.certificates.v1alpha1.ClusterTrustBundlePatch,
)
}
/**
* @see [ClusterTrustBundlePatch].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [ClusterTrustBundlePatch].
*/
public suspend fun clusterTrustBundlePatch(
name: String,
block: suspend ClusterTrustBundlePatchResourceBuilder.() -> Unit,
): ClusterTrustBundlePatch {
val builder = ClusterTrustBundlePatchResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [ClusterTrustBundlePatch].
* @param name The _unique_ name of the resulting resource.
*/
public fun clusterTrustBundlePatch(name: String): ClusterTrustBundlePatch {
val builder = ClusterTrustBundlePatchResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy