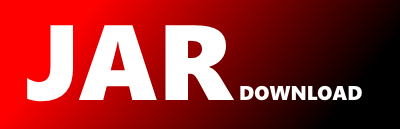
com.pulumi.kubernetes.discovery.v1.kotlin.inputs.EndpointArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.discovery.v1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.kubernetes.core.v1.kotlin.inputs.ObjectReferenceArgs
import com.pulumi.kubernetes.core.v1.kotlin.inputs.ObjectReferenceArgsBuilder
import com.pulumi.kubernetes.discovery.v1.inputs.EndpointArgs.builder
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Endpoint represents a single logical "backend" implementing a service.
* @property addresses addresses of this endpoint. The contents of this field are interpreted according to the corresponding EndpointSlice addressType field. Consumers must handle different types of addresses in the context of their own capabilities. This must contain at least one address but no more than 100. These are all assumed to be fungible and clients may choose to only use the first element. Refer to: https://issue.k8s.io/106267
* @property conditions conditions contains information about the current status of the endpoint.
* @property deprecatedTopology deprecatedTopology contains topology information part of the v1beta1 API. This field is deprecated, and will be removed when the v1beta1 API is removed (no sooner than kubernetes v1.24). While this field can hold values, it is not writable through the v1 API, and any attempts to write to it will be silently ignored. Topology information can be found in the zone and nodeName fields instead.
* @property hints hints contains information associated with how an endpoint should be consumed.
* @property hostname hostname of this endpoint. This field may be used by consumers of endpoints to distinguish endpoints from each other (e.g. in DNS names). Multiple endpoints which use the same hostname should be considered fungible (e.g. multiple A values in DNS). Must be lowercase and pass DNS Label (RFC 1123) validation.
* @property nodeName nodeName represents the name of the Node hosting this endpoint. This can be used to determine endpoints local to a Node.
* @property targetRef targetRef is a reference to a Kubernetes object that represents this endpoint.
* @property zone zone is the name of the Zone this endpoint exists in.
*/
public data class EndpointArgs(
public val addresses: Output>,
public val conditions: Output? = null,
public val deprecatedTopology: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy