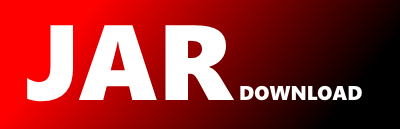
com.pulumi.kubernetes.discovery.v1beta1.kotlin.inputs.EndpointConditionsArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.discovery.v1beta1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kubernetes.discovery.v1beta1.inputs.EndpointConditionsArgs.builder
import kotlin.Boolean
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* EndpointConditions represents the current condition of an endpoint.
* @property ready ready indicates that this endpoint is prepared to receive traffic, according to whatever system is managing the endpoint. A nil value indicates an unknown state. In most cases consumers should interpret this unknown state as ready.
* @property serving serving is identical to ready except that it is set regardless of the terminating state of endpoints. This condition should be set to true for a ready endpoint that is terminating. If nil, consumers should defer to the ready condition. This field can be enabled with the EndpointSliceTerminatingCondition feature gate.
* @property terminating terminating indicates that this endpoint is terminating. A nil value indicates an unknown state. Consumers should interpret this unknown state to mean that the endpoint is not terminating. This field can be enabled with the EndpointSliceTerminatingCondition feature gate.
*/
public data class EndpointConditionsArgs(
public val ready: Output? = null,
public val serving: Output? = null,
public val terminating: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.discovery.v1beta1.inputs.EndpointConditionsArgs =
com.pulumi.kubernetes.discovery.v1beta1.inputs.EndpointConditionsArgs.builder()
.ready(ready?.applyValue({ args0 -> args0 }))
.serving(serving?.applyValue({ args0 -> args0 }))
.terminating(terminating?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [EndpointConditionsArgs].
*/
@PulumiTagMarker
public class EndpointConditionsArgsBuilder internal constructor() {
private var ready: Output? = null
private var serving: Output? = null
private var terminating: Output? = null
/**
* @param value ready indicates that this endpoint is prepared to receive traffic, according to whatever system is managing the endpoint. A nil value indicates an unknown state. In most cases consumers should interpret this unknown state as ready.
*/
@JvmName("wjjllvvrgdoljund")
public suspend fun ready(`value`: Output) {
this.ready = value
}
/**
* @param value serving is identical to ready except that it is set regardless of the terminating state of endpoints. This condition should be set to true for a ready endpoint that is terminating. If nil, consumers should defer to the ready condition. This field can be enabled with the EndpointSliceTerminatingCondition feature gate.
*/
@JvmName("bfmqxbnrqvnudgyn")
public suspend fun serving(`value`: Output) {
this.serving = value
}
/**
* @param value terminating indicates that this endpoint is terminating. A nil value indicates an unknown state. Consumers should interpret this unknown state to mean that the endpoint is not terminating. This field can be enabled with the EndpointSliceTerminatingCondition feature gate.
*/
@JvmName("dafschihixaxmiqw")
public suspend fun terminating(`value`: Output) {
this.terminating = value
}
/**
* @param value ready indicates that this endpoint is prepared to receive traffic, according to whatever system is managing the endpoint. A nil value indicates an unknown state. In most cases consumers should interpret this unknown state as ready.
*/
@JvmName("xdjmspjpfyuldkat")
public suspend fun ready(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ready = mapped
}
/**
* @param value serving is identical to ready except that it is set regardless of the terminating state of endpoints. This condition should be set to true for a ready endpoint that is terminating. If nil, consumers should defer to the ready condition. This field can be enabled with the EndpointSliceTerminatingCondition feature gate.
*/
@JvmName("nblchdhgolieyuuq")
public suspend fun serving(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serving = mapped
}
/**
* @param value terminating indicates that this endpoint is terminating. A nil value indicates an unknown state. Consumers should interpret this unknown state to mean that the endpoint is not terminating. This field can be enabled with the EndpointSliceTerminatingCondition feature gate.
*/
@JvmName("vnlyxivdmulmabgq")
public suspend fun terminating(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.terminating = mapped
}
internal fun build(): EndpointConditionsArgs = EndpointConditionsArgs(
ready = ready,
serving = serving,
terminating = terminating,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy