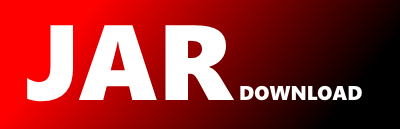
com.pulumi.kubernetes.events.v1.kotlin.EventArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.events.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.kubernetes.core.v1.kotlin.inputs.EventSourceArgs
import com.pulumi.kubernetes.core.v1.kotlin.inputs.EventSourceArgsBuilder
import com.pulumi.kubernetes.core.v1.kotlin.inputs.ObjectReferenceArgs
import com.pulumi.kubernetes.core.v1.kotlin.inputs.ObjectReferenceArgsBuilder
import com.pulumi.kubernetes.events.v1.EventArgs.builder
import com.pulumi.kubernetes.events.v1.kotlin.inputs.EventSeriesArgs
import com.pulumi.kubernetes.events.v1.kotlin.inputs.EventSeriesArgsBuilder
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.ObjectMetaArgs
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.ObjectMetaArgsBuilder
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Event is a report of an event somewhere in the cluster. It generally denotes some state change in the system. Events have a limited retention time and triggers and messages may evolve with time. Event consumers should not rely on the timing of an event with a given Reason reflecting a consistent underlying trigger, or the continued existence of events with that Reason. Events should be treated as informative, best-effort, supplemental data.
* @property action action is what action was taken/failed regarding to the regarding object. It is machine-readable. This field cannot be empty for new Events and it can have at most 128 characters.
* @property apiVersion APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
* @property deprecatedCount deprecatedCount is the deprecated field assuring backward compatibility with core.v1 Event type.
* @property deprecatedFirstTimestamp deprecatedFirstTimestamp is the deprecated field assuring backward compatibility with core.v1 Event type.
* @property deprecatedLastTimestamp deprecatedLastTimestamp is the deprecated field assuring backward compatibility with core.v1 Event type.
* @property deprecatedSource deprecatedSource is the deprecated field assuring backward compatibility with core.v1 Event type.
* @property eventTime eventTime is the time when this Event was first observed. It is required.
* @property kind Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
* @property metadata Standard object's metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
* @property note note is a human-readable description of the status of this operation. Maximal length of the note is 1kB, but libraries should be prepared to handle values up to 64kB.
* @property reason reason is why the action was taken. It is human-readable. This field cannot be empty for new Events and it can have at most 128 characters.
* @property regarding regarding contains the object this Event is about. In most cases it's an Object reporting controller implements, e.g. ReplicaSetController implements ReplicaSets and this event is emitted because it acts on some changes in a ReplicaSet object.
* @property related related is the optional secondary object for more complex actions. E.g. when regarding object triggers a creation or deletion of related object.
* @property reportingController reportingController is the name of the controller that emitted this Event, e.g. `kubernetes.io/kubelet`. This field cannot be empty for new Events.
* @property reportingInstance reportingInstance is the ID of the controller instance, e.g. `kubelet-xyzf`. This field cannot be empty for new Events and it can have at most 128 characters.
* @property series series is data about the Event series this event represents or nil if it's a singleton Event.
* @property type type is the type of this event (Normal, Warning), new types could be added in the future. It is machine-readable. This field cannot be empty for new Events.
*/
public data class EventArgs(
public val action: Output? = null,
public val apiVersion: Output? = null,
public val deprecatedCount: Output? = null,
public val deprecatedFirstTimestamp: Output? = null,
public val deprecatedLastTimestamp: Output? = null,
public val deprecatedSource: Output? = null,
public val eventTime: Output? = null,
public val kind: Output? = null,
public val metadata: Output? = null,
public val note: Output? = null,
public val reason: Output? = null,
public val regarding: Output? = null,
public val related: Output? = null,
public val reportingController: Output? = null,
public val reportingInstance: Output? = null,
public val series: Output? = null,
public val type: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.events.v1.EventArgs =
com.pulumi.kubernetes.events.v1.EventArgs.builder()
.action(action?.applyValue({ args0 -> args0 }))
.apiVersion(apiVersion?.applyValue({ args0 -> args0 }))
.deprecatedCount(deprecatedCount?.applyValue({ args0 -> args0 }))
.deprecatedFirstTimestamp(deprecatedFirstTimestamp?.applyValue({ args0 -> args0 }))
.deprecatedLastTimestamp(deprecatedLastTimestamp?.applyValue({ args0 -> args0 }))
.deprecatedSource(deprecatedSource?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.eventTime(eventTime?.applyValue({ args0 -> args0 }))
.kind(kind?.applyValue({ args0 -> args0 }))
.metadata(metadata?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.note(note?.applyValue({ args0 -> args0 }))
.reason(reason?.applyValue({ args0 -> args0 }))
.regarding(regarding?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.related(related?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.reportingController(reportingController?.applyValue({ args0 -> args0 }))
.reportingInstance(reportingInstance?.applyValue({ args0 -> args0 }))
.series(series?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.type(type?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [EventArgs].
*/
@PulumiTagMarker
public class EventArgsBuilder internal constructor() {
private var action: Output? = null
private var apiVersion: Output? = null
private var deprecatedCount: Output? = null
private var deprecatedFirstTimestamp: Output? = null
private var deprecatedLastTimestamp: Output? = null
private var deprecatedSource: Output? = null
private var eventTime: Output? = null
private var kind: Output? = null
private var metadata: Output? = null
private var note: Output? = null
private var reason: Output? = null
private var regarding: Output? = null
private var related: Output? = null
private var reportingController: Output? = null
private var reportingInstance: Output? = null
private var series: Output? = null
private var type: Output? = null
/**
* @param value action is what action was taken/failed regarding to the regarding object. It is machine-readable. This field cannot be empty for new Events and it can have at most 128 characters.
*/
@JvmName("dsnxxxqbogixowin")
public suspend fun action(`value`: Output) {
this.action = value
}
/**
* @param value APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*/
@JvmName("ryraydcnkgtnbvqd")
public suspend fun apiVersion(`value`: Output) {
this.apiVersion = value
}
/**
* @param value deprecatedCount is the deprecated field assuring backward compatibility with core.v1 Event type.
*/
@JvmName("rrpvbikhgmqemrgs")
public suspend fun deprecatedCount(`value`: Output) {
this.deprecatedCount = value
}
/**
* @param value deprecatedFirstTimestamp is the deprecated field assuring backward compatibility with core.v1 Event type.
*/
@JvmName("kkbgmcjyuxgvrvpu")
public suspend fun deprecatedFirstTimestamp(`value`: Output) {
this.deprecatedFirstTimestamp = value
}
/**
* @param value deprecatedLastTimestamp is the deprecated field assuring backward compatibility with core.v1 Event type.
*/
@JvmName("bphblmidorxrtdrd")
public suspend fun deprecatedLastTimestamp(`value`: Output) {
this.deprecatedLastTimestamp = value
}
/**
* @param value deprecatedSource is the deprecated field assuring backward compatibility with core.v1 Event type.
*/
@JvmName("xgndqgxkkevynits")
public suspend fun deprecatedSource(`value`: Output) {
this.deprecatedSource = value
}
/**
* @param value eventTime is the time when this Event was first observed. It is required.
*/
@JvmName("bayxxfnhiuivsxuh")
public suspend fun eventTime(`value`: Output) {
this.eventTime = value
}
/**
* @param value Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
@JvmName("cxherdreqgakwang")
public suspend fun kind(`value`: Output) {
this.kind = value
}
/**
* @param value Standard object's metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
*/
@JvmName("xrmfnwjmeksptgod")
public suspend fun metadata(`value`: Output) {
this.metadata = value
}
/**
* @param value note is a human-readable description of the status of this operation. Maximal length of the note is 1kB, but libraries should be prepared to handle values up to 64kB.
*/
@JvmName("rtjsqeghfvvvhmmy")
public suspend fun note(`value`: Output) {
this.note = value
}
/**
* @param value reason is why the action was taken. It is human-readable. This field cannot be empty for new Events and it can have at most 128 characters.
*/
@JvmName("maoseewptatdbsow")
public suspend fun reason(`value`: Output) {
this.reason = value
}
/**
* @param value regarding contains the object this Event is about. In most cases it's an Object reporting controller implements, e.g. ReplicaSetController implements ReplicaSets and this event is emitted because it acts on some changes in a ReplicaSet object.
*/
@JvmName("lgymnytdctrswtsa")
public suspend fun regarding(`value`: Output) {
this.regarding = value
}
/**
* @param value related is the optional secondary object for more complex actions. E.g. when regarding object triggers a creation or deletion of related object.
*/
@JvmName("iksdtbctporsksgj")
public suspend fun related(`value`: Output) {
this.related = value
}
/**
* @param value reportingController is the name of the controller that emitted this Event, e.g. `kubernetes.io/kubelet`. This field cannot be empty for new Events.
*/
@JvmName("sntdebcvmjgwbant")
public suspend fun reportingController(`value`: Output) {
this.reportingController = value
}
/**
* @param value reportingInstance is the ID of the controller instance, e.g. `kubelet-xyzf`. This field cannot be empty for new Events and it can have at most 128 characters.
*/
@JvmName("mnmysvksynjssqlt")
public suspend fun reportingInstance(`value`: Output) {
this.reportingInstance = value
}
/**
* @param value series is data about the Event series this event represents or nil if it's a singleton Event.
*/
@JvmName("inhgpmwmyfheujta")
public suspend fun series(`value`: Output) {
this.series = value
}
/**
* @param value type is the type of this event (Normal, Warning), new types could be added in the future. It is machine-readable. This field cannot be empty for new Events.
*/
@JvmName("onqwujjsuqtdmjlw")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value action is what action was taken/failed regarding to the regarding object. It is machine-readable. This field cannot be empty for new Events and it can have at most 128 characters.
*/
@JvmName("vuocxpwsyexmsdvf")
public suspend fun action(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.action = mapped
}
/**
* @param value APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*/
@JvmName("jxyavyfhsocfgnxp")
public suspend fun apiVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiVersion = mapped
}
/**
* @param value deprecatedCount is the deprecated field assuring backward compatibility with core.v1 Event type.
*/
@JvmName("wniergxfkpkqbpqp")
public suspend fun deprecatedCount(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deprecatedCount = mapped
}
/**
* @param value deprecatedFirstTimestamp is the deprecated field assuring backward compatibility with core.v1 Event type.
*/
@JvmName("tgyyihmxutrevoby")
public suspend fun deprecatedFirstTimestamp(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deprecatedFirstTimestamp = mapped
}
/**
* @param value deprecatedLastTimestamp is the deprecated field assuring backward compatibility with core.v1 Event type.
*/
@JvmName("vfrgppbddhntixtb")
public suspend fun deprecatedLastTimestamp(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deprecatedLastTimestamp = mapped
}
/**
* @param value deprecatedSource is the deprecated field assuring backward compatibility with core.v1 Event type.
*/
@JvmName("fmpjxrqybnhgmcxn")
public suspend fun deprecatedSource(`value`: EventSourceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deprecatedSource = mapped
}
/**
* @param argument deprecatedSource is the deprecated field assuring backward compatibility with core.v1 Event type.
*/
@JvmName("hlewpukomnigtiwv")
public suspend fun deprecatedSource(argument: suspend EventSourceArgsBuilder.() -> Unit) {
val toBeMapped = EventSourceArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.deprecatedSource = mapped
}
/**
* @param value eventTime is the time when this Event was first observed. It is required.
*/
@JvmName("upbmeyeeoevlfeyb")
public suspend fun eventTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.eventTime = mapped
}
/**
* @param value Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
@JvmName("gdhhqwdnoxitfyre")
public suspend fun kind(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kind = mapped
}
/**
* @param value Standard object's metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
*/
@JvmName("skvnwtlyayxkiypg")
public suspend fun metadata(`value`: ObjectMetaArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.metadata = mapped
}
/**
* @param argument Standard object's metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
*/
@JvmName("vynswbqlofyrsray")
public suspend fun metadata(argument: suspend ObjectMetaArgsBuilder.() -> Unit) {
val toBeMapped = ObjectMetaArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.metadata = mapped
}
/**
* @param value note is a human-readable description of the status of this operation. Maximal length of the note is 1kB, but libraries should be prepared to handle values up to 64kB.
*/
@JvmName("smvlbyrljgufspec")
public suspend fun note(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.note = mapped
}
/**
* @param value reason is why the action was taken. It is human-readable. This field cannot be empty for new Events and it can have at most 128 characters.
*/
@JvmName("pmkromusepoquhqv")
public suspend fun reason(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.reason = mapped
}
/**
* @param value regarding contains the object this Event is about. In most cases it's an Object reporting controller implements, e.g. ReplicaSetController implements ReplicaSets and this event is emitted because it acts on some changes in a ReplicaSet object.
*/
@JvmName("tpmsiceckdovukel")
public suspend fun regarding(`value`: ObjectReferenceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.regarding = mapped
}
/**
* @param argument regarding contains the object this Event is about. In most cases it's an Object reporting controller implements, e.g. ReplicaSetController implements ReplicaSets and this event is emitted because it acts on some changes in a ReplicaSet object.
*/
@JvmName("lcvkdirleqjbdscj")
public suspend fun regarding(argument: suspend ObjectReferenceArgsBuilder.() -> Unit) {
val toBeMapped = ObjectReferenceArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.regarding = mapped
}
/**
* @param value related is the optional secondary object for more complex actions. E.g. when regarding object triggers a creation or deletion of related object.
*/
@JvmName("yygqmpydiwpckymk")
public suspend fun related(`value`: ObjectReferenceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.related = mapped
}
/**
* @param argument related is the optional secondary object for more complex actions. E.g. when regarding object triggers a creation or deletion of related object.
*/
@JvmName("rprsmcorvlpermia")
public suspend fun related(argument: suspend ObjectReferenceArgsBuilder.() -> Unit) {
val toBeMapped = ObjectReferenceArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.related = mapped
}
/**
* @param value reportingController is the name of the controller that emitted this Event, e.g. `kubernetes.io/kubelet`. This field cannot be empty for new Events.
*/
@JvmName("twffwcgcxgwuvajt")
public suspend fun reportingController(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.reportingController = mapped
}
/**
* @param value reportingInstance is the ID of the controller instance, e.g. `kubelet-xyzf`. This field cannot be empty for new Events and it can have at most 128 characters.
*/
@JvmName("vymoqgxmttdscqvs")
public suspend fun reportingInstance(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.reportingInstance = mapped
}
/**
* @param value series is data about the Event series this event represents or nil if it's a singleton Event.
*/
@JvmName("ukjdjjqqdqeheovp")
public suspend fun series(`value`: EventSeriesArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.series = mapped
}
/**
* @param argument series is data about the Event series this event represents or nil if it's a singleton Event.
*/
@JvmName("ihwelgqnuqmlucht")
public suspend fun series(argument: suspend EventSeriesArgsBuilder.() -> Unit) {
val toBeMapped = EventSeriesArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.series = mapped
}
/**
* @param value type is the type of this event (Normal, Warning), new types could be added in the future. It is machine-readable. This field cannot be empty for new Events.
*/
@JvmName("wrpkruxmvdxnhune")
public suspend fun type(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.type = mapped
}
internal fun build(): EventArgs = EventArgs(
action = action,
apiVersion = apiVersion,
deprecatedCount = deprecatedCount,
deprecatedFirstTimestamp = deprecatedFirstTimestamp,
deprecatedLastTimestamp = deprecatedLastTimestamp,
deprecatedSource = deprecatedSource,
eventTime = eventTime,
kind = kind,
metadata = metadata,
note = note,
reason = reason,
regarding = regarding,
related = related,
reportingController = reportingController,
reportingInstance = reportingInstance,
series = series,
type = type,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy