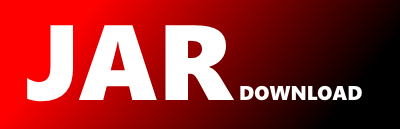
com.pulumi.kubernetes.events.v1beta1.kotlin.Event.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.events.v1beta1.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.kubernetes.core.v1.kotlin.outputs.EventSource
import com.pulumi.kubernetes.core.v1.kotlin.outputs.ObjectReference
import com.pulumi.kubernetes.events.v1beta1.kotlin.outputs.EventSeries
import com.pulumi.kubernetes.meta.v1.kotlin.outputs.ObjectMeta
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.kubernetes.core.v1.kotlin.outputs.EventSource.Companion.toKotlin as eventSourceToKotlin
import com.pulumi.kubernetes.core.v1.kotlin.outputs.ObjectReference.Companion.toKotlin as objectReferenceToKotlin
import com.pulumi.kubernetes.events.v1beta1.kotlin.outputs.EventSeries.Companion.toKotlin as eventSeriesToKotlin
import com.pulumi.kubernetes.meta.v1.kotlin.outputs.ObjectMeta.Companion.toKotlin as objectMetaToKotlin
/**
* Builder for [Event].
*/
@PulumiTagMarker
public class EventResourceBuilder internal constructor() {
public var name: String? = null
public var args: EventArgs = EventArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend EventArgsBuilder.() -> Unit) {
val builder = EventArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Event {
val builtJavaResource = com.pulumi.kubernetes.events.v1beta1.Event(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Event(builtJavaResource)
}
}
/**
* Event is a report of an event somewhere in the cluster. It generally denotes some state change in the system.
*/
public class Event internal constructor(
override val javaResource: com.pulumi.kubernetes.events.v1beta1.Event,
) : KotlinCustomResource(javaResource, EventMapper) {
/**
* What action was taken/failed regarding to the regarding object.
*/
public val action: Output
get() = javaResource.action().applyValue({ args0 -> args0 })
/**
* APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*/
public val apiVersion: Output
get() = javaResource.apiVersion().applyValue({ args0 -> args0 })
/**
* Deprecated field assuring backward compatibility with core.v1 Event type
*/
public val deprecatedCount: Output
get() = javaResource.deprecatedCount().applyValue({ args0 -> args0 })
/**
* Deprecated field assuring backward compatibility with core.v1 Event type
*/
public val deprecatedFirstTimestamp: Output
get() = javaResource.deprecatedFirstTimestamp().applyValue({ args0 -> args0 })
/**
* Deprecated field assuring backward compatibility with core.v1 Event type
*/
public val deprecatedLastTimestamp: Output
get() = javaResource.deprecatedLastTimestamp().applyValue({ args0 -> args0 })
/**
* Deprecated field assuring backward compatibility with core.v1 Event type
*/
public val deprecatedSource: Output
get() = javaResource.deprecatedSource().applyValue({ args0 ->
args0.let({ args0 ->
eventSourceToKotlin(args0)
})
})
/**
* Required. Time when this Event was first observed.
*/
public val eventTime: Output
get() = javaResource.eventTime().applyValue({ args0 -> args0 })
/**
* Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
public val kind: Output
get() = javaResource.kind().applyValue({ args0 -> args0 })
public val metadata: Output
get() = javaResource.metadata().applyValue({ args0 ->
args0.let({ args0 ->
objectMetaToKotlin(args0)
})
})
/**
* Optional. A human-readable description of the status of this operation. Maximal length of the note is 1kB, but libraries should be prepared to handle values up to 64kB.
*/
public val note: Output
get() = javaResource.note().applyValue({ args0 -> args0 })
/**
* Why the action was taken.
*/
public val reason: Output
get() = javaResource.reason().applyValue({ args0 -> args0 })
/**
* The object this Event is about. In most cases it's an Object reporting controller implements. E.g. ReplicaSetController implements ReplicaSets and this event is emitted because it acts on some changes in a ReplicaSet object.
*/
public val regarding: Output
get() = javaResource.regarding().applyValue({ args0 ->
args0.let({ args0 ->
objectReferenceToKotlin(args0)
})
})
/**
* Optional secondary object for more complex actions. E.g. when regarding object triggers a creation or deletion of related object.
*/
public val related: Output
get() = javaResource.related().applyValue({ args0 ->
args0.let({ args0 ->
objectReferenceToKotlin(args0)
})
})
/**
* Name of the controller that emitted this Event, e.g. `kubernetes.io/kubelet`.
*/
public val reportingController: Output
get() = javaResource.reportingController().applyValue({ args0 -> args0 })
/**
* ID of the controller instance, e.g. `kubelet-xyzf`.
*/
public val reportingInstance: Output
get() = javaResource.reportingInstance().applyValue({ args0 -> args0 })
/**
* Data about the Event series this event represents or nil if it's a singleton Event.
*/
public val series: Output
get() = javaResource.series().applyValue({ args0 ->
args0.let({ args0 ->
eventSeriesToKotlin(args0)
})
})
/**
* Type of this event (Normal, Warning), new types could be added in the future.
*/
public val type: Output
get() = javaResource.type().applyValue({ args0 -> args0 })
}
public object EventMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.kubernetes.events.v1beta1.Event::class == javaResource::class
override fun map(javaResource: Resource): Event = Event(
javaResource as
com.pulumi.kubernetes.events.v1beta1.Event,
)
}
/**
* @see [Event].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Event].
*/
public suspend fun event(name: String, block: suspend EventResourceBuilder.() -> Unit): Event {
val builder = EventResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Event].
* @param name The _unique_ name of the resulting resource.
*/
public fun event(name: String): Event {
val builder = EventResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy