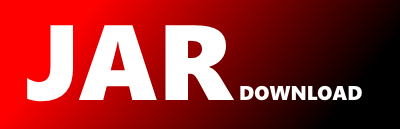
com.pulumi.kubernetes.extensions.v1beta1.kotlin.inputs.HTTPIngressPathArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.extensions.v1beta1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.kubernetes.extensions.v1beta1.inputs.HTTPIngressPathArgs.builder
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* HTTPIngressPath associates a path regex with a backend. Incoming urls matching the path are forwarded to the backend.
* @property backend Backend defines the referenced service endpoint to which the traffic will be forwarded to.
* @property path Path is an extended POSIX regex as defined by IEEE Std 1003.1, (i.e this follows the egrep/unix syntax, not the perl syntax) matched against the path of an incoming request. Currently it can contain characters disallowed from the conventional "path" part of a URL as defined by RFC 3986. Paths must begin with a '/'. If unspecified, the path defaults to a catch all sending traffic to the backend.
* @property pathType PathType determines the interpretation of the Path matching. PathType can be one of the following values: * Exact: Matches the URL path exactly. * Prefix: Matches based on a URL path prefix split by '/'. Matching is
* done on a path element by element basis. A path element refers is the
* list of labels in the path split by the '/' separator. A request is a
* match for path p if every p is an element-wise prefix of p of the
* request path. Note that if the last element of the path is a substring
* of the last element in request path, it is not a match (e.g. /foo/bar
* matches /foo/bar/baz, but does not match /foo/barbaz).
* * ImplementationSpecific: Interpretation of the Path matching is up to
* the IngressClass. Implementations can treat this as a separate PathType
* or treat it identically to Prefix or Exact path types.
* Implementations are required to support all path types. Defaults to ImplementationSpecific.
*/
public data class HTTPIngressPathArgs(
public val backend: Output,
public val path: Output? = null,
public val pathType: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.extensions.v1beta1.inputs.HTTPIngressPathArgs =
com.pulumi.kubernetes.extensions.v1beta1.inputs.HTTPIngressPathArgs.builder()
.backend(backend.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.path(path?.applyValue({ args0 -> args0 }))
.pathType(pathType?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [HTTPIngressPathArgs].
*/
@PulumiTagMarker
public class HTTPIngressPathArgsBuilder internal constructor() {
private var backend: Output? = null
private var path: Output? = null
private var pathType: Output? = null
/**
* @param value Backend defines the referenced service endpoint to which the traffic will be forwarded to.
*/
@JvmName("yjydrhtsinpnjqtc")
public suspend fun backend(`value`: Output) {
this.backend = value
}
/**
* @param value Path is an extended POSIX regex as defined by IEEE Std 1003.1, (i.e this follows the egrep/unix syntax, not the perl syntax) matched against the path of an incoming request. Currently it can contain characters disallowed from the conventional "path" part of a URL as defined by RFC 3986. Paths must begin with a '/'. If unspecified, the path defaults to a catch all sending traffic to the backend.
*/
@JvmName("uqhshepgvagiywkg")
public suspend fun path(`value`: Output) {
this.path = value
}
/**
* @param value PathType determines the interpretation of the Path matching. PathType can be one of the following values: * Exact: Matches the URL path exactly. * Prefix: Matches based on a URL path prefix split by '/'. Matching is
* done on a path element by element basis. A path element refers is the
* list of labels in the path split by the '/' separator. A request is a
* match for path p if every p is an element-wise prefix of p of the
* request path. Note that if the last element of the path is a substring
* of the last element in request path, it is not a match (e.g. /foo/bar
* matches /foo/bar/baz, but does not match /foo/barbaz).
* * ImplementationSpecific: Interpretation of the Path matching is up to
* the IngressClass. Implementations can treat this as a separate PathType
* or treat it identically to Prefix or Exact path types.
* Implementations are required to support all path types. Defaults to ImplementationSpecific.
*/
@JvmName("njvpruuugqlormmn")
public suspend fun pathType(`value`: Output) {
this.pathType = value
}
/**
* @param value Backend defines the referenced service endpoint to which the traffic will be forwarded to.
*/
@JvmName("selvrojpupmdqktb")
public suspend fun backend(`value`: IngressBackendArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.backend = mapped
}
/**
* @param argument Backend defines the referenced service endpoint to which the traffic will be forwarded to.
*/
@JvmName("xndkkeyfmruonwwc")
public suspend fun backend(argument: suspend IngressBackendArgsBuilder.() -> Unit) {
val toBeMapped = IngressBackendArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.backend = mapped
}
/**
* @param value Path is an extended POSIX regex as defined by IEEE Std 1003.1, (i.e this follows the egrep/unix syntax, not the perl syntax) matched against the path of an incoming request. Currently it can contain characters disallowed from the conventional "path" part of a URL as defined by RFC 3986. Paths must begin with a '/'. If unspecified, the path defaults to a catch all sending traffic to the backend.
*/
@JvmName("vhnkyykhpslkheww")
public suspend fun path(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.path = mapped
}
/**
* @param value PathType determines the interpretation of the Path matching. PathType can be one of the following values: * Exact: Matches the URL path exactly. * Prefix: Matches based on a URL path prefix split by '/'. Matching is
* done on a path element by element basis. A path element refers is the
* list of labels in the path split by the '/' separator. A request is a
* match for path p if every p is an element-wise prefix of p of the
* request path. Note that if the last element of the path is a substring
* of the last element in request path, it is not a match (e.g. /foo/bar
* matches /foo/bar/baz, but does not match /foo/barbaz).
* * ImplementationSpecific: Interpretation of the Path matching is up to
* the IngressClass. Implementations can treat this as a separate PathType
* or treat it identically to Prefix or Exact path types.
* Implementations are required to support all path types. Defaults to ImplementationSpecific.
*/
@JvmName("hmodqosxoymtwlxa")
public suspend fun pathType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.pathType = mapped
}
internal fun build(): HTTPIngressPathArgs = HTTPIngressPathArgs(
backend = backend ?: throw PulumiNullFieldException("backend"),
path = path,
pathType = pathType,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy