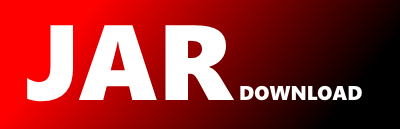
com.pulumi.kubernetes.flowcontrol.v1.kotlin.FlowSchemaArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.flowcontrol.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.kubernetes.flowcontrol.v1.FlowSchemaArgs.builder
import com.pulumi.kubernetes.flowcontrol.v1.kotlin.inputs.FlowSchemaSpecArgs
import com.pulumi.kubernetes.flowcontrol.v1.kotlin.inputs.FlowSchemaSpecArgsBuilder
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.ObjectMetaArgs
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.ObjectMetaArgsBuilder
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* FlowSchema defines the schema of a group of flows. Note that a flow is made up of a set of inbound API requests with similar attributes and is identified by a pair of strings: the name of the FlowSchema and a "flow distinguisher".
* @property apiVersion APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
* @property kind Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
* @property metadata `metadata` is the standard object's metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
* @property spec `spec` is the specification of the desired behavior of a FlowSchema. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#spec-and-status
*/
public data class FlowSchemaArgs(
public val apiVersion: Output? = null,
public val kind: Output? = null,
public val metadata: Output? = null,
public val spec: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.flowcontrol.v1.FlowSchemaArgs =
com.pulumi.kubernetes.flowcontrol.v1.FlowSchemaArgs.builder()
.apiVersion(apiVersion?.applyValue({ args0 -> args0 }))
.kind(kind?.applyValue({ args0 -> args0 }))
.metadata(metadata?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.spec(spec?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [FlowSchemaArgs].
*/
@PulumiTagMarker
public class FlowSchemaArgsBuilder internal constructor() {
private var apiVersion: Output? = null
private var kind: Output? = null
private var metadata: Output? = null
private var spec: Output? = null
/**
* @param value APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*/
@JvmName("kimawpqdgfvfhdul")
public suspend fun apiVersion(`value`: Output) {
this.apiVersion = value
}
/**
* @param value Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
@JvmName("cakcqkyldjfpoixx")
public suspend fun kind(`value`: Output) {
this.kind = value
}
/**
* @param value `metadata` is the standard object's metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
*/
@JvmName("gjqwgotsjaemixgg")
public suspend fun metadata(`value`: Output) {
this.metadata = value
}
/**
* @param value `spec` is the specification of the desired behavior of a FlowSchema. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#spec-and-status
*/
@JvmName("mrgmmmlxrweclyku")
public suspend fun spec(`value`: Output) {
this.spec = value
}
/**
* @param value APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*/
@JvmName("amkisfypgdxmijhw")
public suspend fun apiVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiVersion = mapped
}
/**
* @param value Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
@JvmName("cydoqcsfqvxucxju")
public suspend fun kind(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kind = mapped
}
/**
* @param value `metadata` is the standard object's metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
*/
@JvmName("ptrbkldconybiiwr")
public suspend fun metadata(`value`: ObjectMetaArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.metadata = mapped
}
/**
* @param argument `metadata` is the standard object's metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
*/
@JvmName("jylxkhfveicpmryv")
public suspend fun metadata(argument: suspend ObjectMetaArgsBuilder.() -> Unit) {
val toBeMapped = ObjectMetaArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.metadata = mapped
}
/**
* @param value `spec` is the specification of the desired behavior of a FlowSchema. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#spec-and-status
*/
@JvmName("fwfgjobkpljnvocr")
public suspend fun spec(`value`: FlowSchemaSpecArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.spec = mapped
}
/**
* @param argument `spec` is the specification of the desired behavior of a FlowSchema. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#spec-and-status
*/
@JvmName("bedoiaebcqbcpdhr")
public suspend fun spec(argument: suspend FlowSchemaSpecArgsBuilder.() -> Unit) {
val toBeMapped = FlowSchemaSpecArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.spec = mapped
}
internal fun build(): FlowSchemaArgs = FlowSchemaArgs(
apiVersion = apiVersion,
kind = kind,
metadata = metadata,
spec = spec,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy