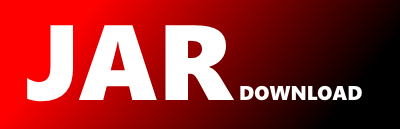
com.pulumi.kubernetes.helm.v3.kotlin.Release.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.helm.v3.kotlin
import com.pulumi.asset.AssetOrArchive
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.kubernetes.helm.v3.kotlin.outputs.ReleaseStatus
import com.pulumi.kubernetes.helm.v3.kotlin.outputs.RepositoryOpts
import com.pulumi.resources.Resource
import kotlin.Any
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import com.pulumi.kubernetes.helm.v3.kotlin.outputs.ReleaseStatus.Companion.toKotlin as releaseStatusToKotlin
import com.pulumi.kubernetes.helm.v3.kotlin.outputs.RepositoryOpts.Companion.toKotlin as repositoryOptsToKotlin
/**
* Builder for [Release].
*/
@PulumiTagMarker
public class ReleaseResourceBuilder internal constructor() {
public var name: String? = null
public var args: ReleaseArgs = ReleaseArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ReleaseArgsBuilder.() -> Unit) {
val builder = ReleaseArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Release {
val builtJavaResource = com.pulumi.kubernetes.helm.v3.Release(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Release(builtJavaResource)
}
}
/**
* A `Release` is an instance of a chart running in a Kubernetes cluster. A `Chart` is a Helm package. It contains all the
* resource definitions necessary to run an application, tool, or service inside a Kubernetes cluster.
* This resource models a Helm Release as if it were created by the Helm CLI. The underlying implementation embeds Helm as
* a library to perform the orchestration of the resources. As a result, the full spectrum of Helm features are supported
* natively.
* You may also want to consider the `Chart` resource as an alternative method for managing helm charts. For more information about the trade-offs between these options see: [Choosing the right Helm resource for your use case](https://www.pulumi.com/registry/packages/kubernetes/how-to-guides/choosing-the-right-helm-resource-for-your-use-case)
* ## Example Usage
* ### Local Chart Directory
* No Java example available.
* ### Remote Chart
* No Java example available.
* ### Set Chart Values
* No Java example available.
* ### Deploy Chart into Namespace
* No Java example available.
* ### Depend on a Chart resource
* No Java example available.
* ### Specify Helm Chart Values in File and Code
* No Java example available.
* ### Query Kubernetes Resource Installed By Helm Chart
* No Java example available.
* ## Import
* An existing Helm Release resource can be imported using its `type token`, `name` and identifier, e.g.
* ```sh
* $ pulumi import kubernetes:helm.sh/v3:Release myRelease /
* ```
*/
public class Release internal constructor(
override val javaResource: com.pulumi.kubernetes.helm.v3.Release,
) : KotlinCustomResource(javaResource, ReleaseMapper) {
/**
* Whether to allow Null values in helm chart configs.
*/
public val allowNullValues: Output?
get() = javaResource.allowNullValues().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* If set, installation process purges chart on fail. `skipAwait` will be disabled automatically if atomic is used.
*/
public val atomic: Output?
get() = javaResource.atomic().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Chart name to be installed. A path may be used.
*/
public val chart: Output
get() = javaResource.chart().applyValue({ args0 -> args0 })
/**
* Allow deletion of new resources created in this upgrade when upgrade fails.
*/
public val cleanupOnFail: Output?
get() = javaResource.cleanupOnFail().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Create the namespace if it does not exist.
*/
public val createNamespace: Output?
get() = javaResource.createNamespace().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Run helm dependency update before installing the chart.
*/
public val dependencyUpdate: Output?
get() = javaResource.dependencyUpdate().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Add a custom description
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Use chart development versions, too. Equivalent to version '>0.0.0-0'. If `version` is set, this is ignored.
*/
public val devel: Output?
get() = javaResource.devel().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Prevent CRD hooks from running, but run other hooks. See helm install --no-crd-hook
*/
public val disableCRDHooks: Output?
get() = javaResource.disableCRDHooks().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* If set, the installation process will not validate rendered templates against the Kubernetes OpenAPI Schema
*/
public val disableOpenapiValidation: Output?
get() = javaResource.disableOpenapiValidation().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Prevent hooks from running.
*/
public val disableWebhooks: Output?
get() = javaResource.disableWebhooks().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Force resource update through delete/recreate if needed.
*/
public val forceUpdate: Output?
get() = javaResource.forceUpdate().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Location of public keys used for verification. Used only if `verify` is true
*/
public val keyring: Output?
get() = javaResource.keyring().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Run helm lint when planning.
*/
public val lint: Output?
get() = javaResource.lint().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The rendered manifests as JSON. Not yet supported.
*/
public val manifest: Output
© 2015 - 2024 Weber Informatics LLC | Privacy Policy