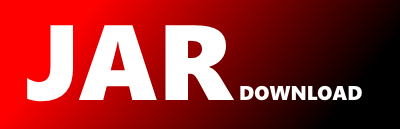
com.pulumi.kubernetes.meta.v1.kotlin.StatusArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.meta.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.kubernetes.meta.v1.StatusArgs.builder
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.ListMetaArgs
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.ListMetaArgsBuilder
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.StatusDetailsArgs
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.StatusDetailsArgsBuilder
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Status is a return value for calls that don't return other objects.
* @property apiVersion APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
* @property code Suggested HTTP return code for this status, 0 if not set.
* @property details Extended data associated with the reason. Each reason may define its own extended details. This field is optional and the data returned is not guaranteed to conform to any schema except that defined by the reason type.
* @property kind Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
* @property message A human-readable description of the status of this operation.
* @property metadata Standard list metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
* @property reason A machine-readable description of why this operation is in the "Failure" status. If this value is empty there is no information available. A Reason clarifies an HTTP status code but does not override it.
*/
public data class StatusArgs(
public val apiVersion: Output? = null,
public val code: Output? = null,
public val details: Output? = null,
public val kind: Output? = null,
public val message: Output? = null,
public val metadata: Output? = null,
public val reason: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.meta.v1.StatusArgs =
com.pulumi.kubernetes.meta.v1.StatusArgs.builder()
.apiVersion(apiVersion?.applyValue({ args0 -> args0 }))
.code(code?.applyValue({ args0 -> args0 }))
.details(details?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.kind(kind?.applyValue({ args0 -> args0 }))
.message(message?.applyValue({ args0 -> args0 }))
.metadata(metadata?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.reason(reason?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [StatusArgs].
*/
@PulumiTagMarker
public class StatusArgsBuilder internal constructor() {
private var apiVersion: Output? = null
private var code: Output? = null
private var details: Output? = null
private var kind: Output? = null
private var message: Output? = null
private var metadata: Output? = null
private var reason: Output? = null
/**
* @param value APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*/
@JvmName("khrnkufdvqkmpuqr")
public suspend fun apiVersion(`value`: Output) {
this.apiVersion = value
}
/**
* @param value Suggested HTTP return code for this status, 0 if not set.
*/
@JvmName("mkqnqnhaammxphdo")
public suspend fun code(`value`: Output) {
this.code = value
}
/**
* @param value Extended data associated with the reason. Each reason may define its own extended details. This field is optional and the data returned is not guaranteed to conform to any schema except that defined by the reason type.
*/
@JvmName("gqoxebxmfommtgri")
public suspend fun details(`value`: Output) {
this.details = value
}
/**
* @param value Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
@JvmName("vnrtbijqbauvakdl")
public suspend fun kind(`value`: Output) {
this.kind = value
}
/**
* @param value A human-readable description of the status of this operation.
*/
@JvmName("fjushvnpehvaapde")
public suspend fun message(`value`: Output) {
this.message = value
}
/**
* @param value Standard list metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
@JvmName("jgiixqsixxjwkijo")
public suspend fun metadata(`value`: Output) {
this.metadata = value
}
/**
* @param value A machine-readable description of why this operation is in the "Failure" status. If this value is empty there is no information available. A Reason clarifies an HTTP status code but does not override it.
*/
@JvmName("ljbamnkautqjinhr")
public suspend fun reason(`value`: Output) {
this.reason = value
}
/**
* @param value APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*/
@JvmName("kuqwomxkffuaekhw")
public suspend fun apiVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiVersion = mapped
}
/**
* @param value Suggested HTTP return code for this status, 0 if not set.
*/
@JvmName("kuugmbvsnxjrjinh")
public suspend fun code(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.code = mapped
}
/**
* @param value Extended data associated with the reason. Each reason may define its own extended details. This field is optional and the data returned is not guaranteed to conform to any schema except that defined by the reason type.
*/
@JvmName("djxrmabbebuggbbq")
public suspend fun details(`value`: StatusDetailsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.details = mapped
}
/**
* @param argument Extended data associated with the reason. Each reason may define its own extended details. This field is optional and the data returned is not guaranteed to conform to any schema except that defined by the reason type.
*/
@JvmName("jrihnwkaedsqrbwl")
public suspend fun details(argument: suspend StatusDetailsArgsBuilder.() -> Unit) {
val toBeMapped = StatusDetailsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.details = mapped
}
/**
* @param value Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
@JvmName("eaapkvpxcdgfqolg")
public suspend fun kind(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kind = mapped
}
/**
* @param value A human-readable description of the status of this operation.
*/
@JvmName("myojcllbdbpssisp")
public suspend fun message(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.message = mapped
}
/**
* @param value Standard list metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
@JvmName("skjuymssdmvrocwt")
public suspend fun metadata(`value`: ListMetaArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.metadata = mapped
}
/**
* @param argument Standard list metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
@JvmName("tbjwhtmnhrtihqmw")
public suspend fun metadata(argument: suspend ListMetaArgsBuilder.() -> Unit) {
val toBeMapped = ListMetaArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.metadata = mapped
}
/**
* @param value A machine-readable description of why this operation is in the "Failure" status. If this value is empty there is no information available. A Reason clarifies an HTTP status code but does not override it.
*/
@JvmName("qoopeicrridpsmiw")
public suspend fun reason(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.reason = mapped
}
internal fun build(): StatusArgs = StatusArgs(
apiVersion = apiVersion,
code = code,
details = details,
kind = kind,
message = message,
metadata = metadata,
reason = reason,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy