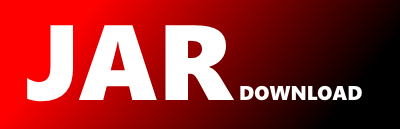
com.pulumi.kubernetes.networking.v1alpha1.kotlin.IPAddressArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.networking.v1alpha1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.ObjectMetaArgs
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.ObjectMetaArgsBuilder
import com.pulumi.kubernetes.networking.v1alpha1.IPAddressArgs.builder
import com.pulumi.kubernetes.networking.v1alpha1.kotlin.inputs.IPAddressSpecArgs
import com.pulumi.kubernetes.networking.v1alpha1.kotlin.inputs.IPAddressSpecArgsBuilder
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* IPAddress represents a single IP of a single IP Family. The object is designed to be used by APIs that operate on IP addresses. The object is used by the Service core API for allocation of IP addresses. An IP address can be represented in different formats, to guarantee the uniqueness of the IP, the name of the object is the IP address in canonical format, four decimal digits separated by dots suppressing leading zeros for IPv4 and the representation defined by RFC 5952 for IPv6. Valid: 192.168.1.5 or 2001:db8::1 or 2001:db8:aaaa:bbbb:cccc:dddd:eeee:1 Invalid: 10.01.2.3 or 2001:db8:0:0:0::1
* @property apiVersion APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
* @property kind Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
* @property metadata Standard object's metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
* @property spec spec is the desired state of the IPAddress. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#spec-and-status
*/
public data class IPAddressArgs(
public val apiVersion: Output? = null,
public val kind: Output? = null,
public val metadata: Output? = null,
public val spec: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.networking.v1alpha1.IPAddressArgs =
com.pulumi.kubernetes.networking.v1alpha1.IPAddressArgs.builder()
.apiVersion(apiVersion?.applyValue({ args0 -> args0 }))
.kind(kind?.applyValue({ args0 -> args0 }))
.metadata(metadata?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.spec(spec?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [IPAddressArgs].
*/
@PulumiTagMarker
public class IPAddressArgsBuilder internal constructor() {
private var apiVersion: Output? = null
private var kind: Output? = null
private var metadata: Output? = null
private var spec: Output? = null
/**
* @param value APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*/
@JvmName("kkhibnwrbixqdjfw")
public suspend fun apiVersion(`value`: Output) {
this.apiVersion = value
}
/**
* @param value Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
@JvmName("prxkmwjcsqikykxb")
public suspend fun kind(`value`: Output) {
this.kind = value
}
/**
* @param value Standard object's metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
*/
@JvmName("bqfsgqujoyfxnevy")
public suspend fun metadata(`value`: Output) {
this.metadata = value
}
/**
* @param value spec is the desired state of the IPAddress. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#spec-and-status
*/
@JvmName("wfcuqeberlnatuio")
public suspend fun spec(`value`: Output) {
this.spec = value
}
/**
* @param value APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*/
@JvmName("mqhckupaqsjnslll")
public suspend fun apiVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiVersion = mapped
}
/**
* @param value Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
@JvmName("nlokkkqdrmicjude")
public suspend fun kind(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kind = mapped
}
/**
* @param value Standard object's metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
*/
@JvmName("fgyuxifsrbthhqjp")
public suspend fun metadata(`value`: ObjectMetaArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.metadata = mapped
}
/**
* @param argument Standard object's metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
*/
@JvmName("pdsxkduyxulfivjs")
public suspend fun metadata(argument: suspend ObjectMetaArgsBuilder.() -> Unit) {
val toBeMapped = ObjectMetaArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.metadata = mapped
}
/**
* @param value spec is the desired state of the IPAddress. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#spec-and-status
*/
@JvmName("csyubjubicaummxm")
public suspend fun spec(`value`: IPAddressSpecArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.spec = mapped
}
/**
* @param argument spec is the desired state of the IPAddress. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#spec-and-status
*/
@JvmName("qlnppdjtjiufcnve")
public suspend fun spec(argument: suspend IPAddressSpecArgsBuilder.() -> Unit) {
val toBeMapped = IPAddressSpecArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.spec = mapped
}
internal fun build(): IPAddressArgs = IPAddressArgs(
apiVersion = apiVersion,
kind = kind,
metadata = metadata,
spec = spec,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy