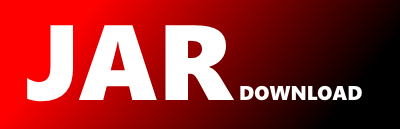
com.pulumi.kubernetes.node.v1beta1.kotlin.RuntimeClassArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.node.v1beta1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.ObjectMetaArgs
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.ObjectMetaArgsBuilder
import com.pulumi.kubernetes.node.v1beta1.RuntimeClassArgs.builder
import com.pulumi.kubernetes.node.v1beta1.kotlin.inputs.OverheadArgs
import com.pulumi.kubernetes.node.v1beta1.kotlin.inputs.OverheadArgsBuilder
import com.pulumi.kubernetes.node.v1beta1.kotlin.inputs.SchedulingArgs
import com.pulumi.kubernetes.node.v1beta1.kotlin.inputs.SchedulingArgsBuilder
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* RuntimeClass defines a class of container runtime supported in the cluster. The RuntimeClass is used to determine which container runtime is used to run all containers in a pod. RuntimeClasses are (currently) manually defined by a user or cluster provisioner, and referenced in the PodSpec. The Kubelet is responsible for resolving the RuntimeClassName reference before running the pod. For more details, see https://git.k8s.io/enhancements/keps/sig-node/runtime-class.md
* @property apiVersion APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
* @property handler Handler specifies the underlying runtime and configuration that the CRI implementation will use to handle pods of this class. The possible values are specific to the node & CRI configuration. It is assumed that all handlers are available on every node, and handlers of the same name are equivalent on every node. For example, a handler called "runc" might specify that the runc OCI runtime (using native Linux containers) will be used to run the containers in a pod. The Handler must conform to the DNS Label (RFC 1123) requirements, and is immutable.
* @property kind Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
* @property metadata More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
* @property overhead Overhead represents the resource overhead associated with running a pod for a given RuntimeClass. For more details, see https://git.k8s.io/enhancements/keps/sig-node/20190226-pod-overhead.md This field is alpha-level as of Kubernetes v1.15, and is only honored by servers that enable the PodOverhead feature.
* @property scheduling Scheduling holds the scheduling constraints to ensure that pods running with this RuntimeClass are scheduled to nodes that support it. If scheduling is nil, this RuntimeClass is assumed to be supported by all nodes.
*/
public data class RuntimeClassArgs(
public val apiVersion: Output? = null,
public val handler: Output? = null,
public val kind: Output? = null,
public val metadata: Output? = null,
public val overhead: Output? = null,
public val scheduling: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.node.v1beta1.RuntimeClassArgs =
com.pulumi.kubernetes.node.v1beta1.RuntimeClassArgs.builder()
.apiVersion(apiVersion?.applyValue({ args0 -> args0 }))
.handler(handler?.applyValue({ args0 -> args0 }))
.kind(kind?.applyValue({ args0 -> args0 }))
.metadata(metadata?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.overhead(overhead?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.scheduling(scheduling?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [RuntimeClassArgs].
*/
@PulumiTagMarker
public class RuntimeClassArgsBuilder internal constructor() {
private var apiVersion: Output? = null
private var handler: Output? = null
private var kind: Output? = null
private var metadata: Output? = null
private var overhead: Output? = null
private var scheduling: Output? = null
/**
* @param value APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*/
@JvmName("ftjbrxiakawsqyul")
public suspend fun apiVersion(`value`: Output) {
this.apiVersion = value
}
/**
* @param value Handler specifies the underlying runtime and configuration that the CRI implementation will use to handle pods of this class. The possible values are specific to the node & CRI configuration. It is assumed that all handlers are available on every node, and handlers of the same name are equivalent on every node. For example, a handler called "runc" might specify that the runc OCI runtime (using native Linux containers) will be used to run the containers in a pod. The Handler must conform to the DNS Label (RFC 1123) requirements, and is immutable.
*/
@JvmName("flmqbsqodfqpqguq")
public suspend fun handler(`value`: Output) {
this.handler = value
}
/**
* @param value Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
@JvmName("kodfuxtqwfqympjn")
public suspend fun kind(`value`: Output) {
this.kind = value
}
/**
* @param value More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
*/
@JvmName("wrtsocphepcspnys")
public suspend fun metadata(`value`: Output) {
this.metadata = value
}
/**
* @param value Overhead represents the resource overhead associated with running a pod for a given RuntimeClass. For more details, see https://git.k8s.io/enhancements/keps/sig-node/20190226-pod-overhead.md This field is alpha-level as of Kubernetes v1.15, and is only honored by servers that enable the PodOverhead feature.
*/
@JvmName("rkabnbujeqcnrckf")
public suspend fun overhead(`value`: Output) {
this.overhead = value
}
/**
* @param value Scheduling holds the scheduling constraints to ensure that pods running with this RuntimeClass are scheduled to nodes that support it. If scheduling is nil, this RuntimeClass is assumed to be supported by all nodes.
*/
@JvmName("ywlntrlpuerlwkpn")
public suspend fun scheduling(`value`: Output) {
this.scheduling = value
}
/**
* @param value APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*/
@JvmName("qphrfonxlgemvqal")
public suspend fun apiVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiVersion = mapped
}
/**
* @param value Handler specifies the underlying runtime and configuration that the CRI implementation will use to handle pods of this class. The possible values are specific to the node & CRI configuration. It is assumed that all handlers are available on every node, and handlers of the same name are equivalent on every node. For example, a handler called "runc" might specify that the runc OCI runtime (using native Linux containers) will be used to run the containers in a pod. The Handler must conform to the DNS Label (RFC 1123) requirements, and is immutable.
*/
@JvmName("grpdvvkengepvxks")
public suspend fun handler(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.handler = mapped
}
/**
* @param value Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
@JvmName("onvvcanweplaaghn")
public suspend fun kind(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kind = mapped
}
/**
* @param value More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
*/
@JvmName("rcbuslivmrmtibtt")
public suspend fun metadata(`value`: ObjectMetaArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.metadata = mapped
}
/**
* @param argument More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
*/
@JvmName("opsayovquptsfutq")
public suspend fun metadata(argument: suspend ObjectMetaArgsBuilder.() -> Unit) {
val toBeMapped = ObjectMetaArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.metadata = mapped
}
/**
* @param value Overhead represents the resource overhead associated with running a pod for a given RuntimeClass. For more details, see https://git.k8s.io/enhancements/keps/sig-node/20190226-pod-overhead.md This field is alpha-level as of Kubernetes v1.15, and is only honored by servers that enable the PodOverhead feature.
*/
@JvmName("bxttobbgjeeinfkn")
public suspend fun overhead(`value`: OverheadArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.overhead = mapped
}
/**
* @param argument Overhead represents the resource overhead associated with running a pod for a given RuntimeClass. For more details, see https://git.k8s.io/enhancements/keps/sig-node/20190226-pod-overhead.md This field is alpha-level as of Kubernetes v1.15, and is only honored by servers that enable the PodOverhead feature.
*/
@JvmName("hupfllsbegblhpla")
public suspend fun overhead(argument: suspend OverheadArgsBuilder.() -> Unit) {
val toBeMapped = OverheadArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.overhead = mapped
}
/**
* @param value Scheduling holds the scheduling constraints to ensure that pods running with this RuntimeClass are scheduled to nodes that support it. If scheduling is nil, this RuntimeClass is assumed to be supported by all nodes.
*/
@JvmName("dshkbuwpbvkfbjlf")
public suspend fun scheduling(`value`: SchedulingArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.scheduling = mapped
}
/**
* @param argument Scheduling holds the scheduling constraints to ensure that pods running with this RuntimeClass are scheduled to nodes that support it. If scheduling is nil, this RuntimeClass is assumed to be supported by all nodes.
*/
@JvmName("xxghadcokttnornv")
public suspend fun scheduling(argument: suspend SchedulingArgsBuilder.() -> Unit) {
val toBeMapped = SchedulingArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.scheduling = mapped
}
internal fun build(): RuntimeClassArgs = RuntimeClassArgs(
apiVersion = apiVersion,
handler = handler,
kind = kind,
metadata = metadata,
overhead = overhead,
scheduling = scheduling,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy