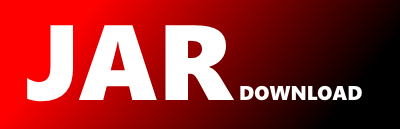
com.pulumi.kubernetes.resource.v1alpha2.kotlin.inputs.ResourceClaimConsumerReferenceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.resource.v1alpha2.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kubernetes.resource.v1alpha2.inputs.ResourceClaimConsumerReferenceArgs.builder
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* ResourceClaimConsumerReference contains enough information to let you locate the consumer of a ResourceClaim. The user must be a resource in the same namespace as the ResourceClaim.
* @property apiGroup APIGroup is the group for the resource being referenced. It is empty for the core API. This matches the group in the APIVersion that is used when creating the resources.
* @property name Name is the name of resource being referenced.
* @property resource Resource is the type of resource being referenced, for example "pods".
* @property uid UID identifies exactly one incarnation of the resource.
*/
public data class ResourceClaimConsumerReferenceArgs(
public val apiGroup: Output? = null,
public val name: Output,
public val resource: Output,
public val uid: Output,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.resource.v1alpha2.inputs.ResourceClaimConsumerReferenceArgs =
com.pulumi.kubernetes.resource.v1alpha2.inputs.ResourceClaimConsumerReferenceArgs.builder()
.apiGroup(apiGroup?.applyValue({ args0 -> args0 }))
.name(name.applyValue({ args0 -> args0 }))
.resource(resource.applyValue({ args0 -> args0 }))
.uid(uid.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ResourceClaimConsumerReferenceArgs].
*/
@PulumiTagMarker
public class ResourceClaimConsumerReferenceArgsBuilder internal constructor() {
private var apiGroup: Output? = null
private var name: Output? = null
private var resource: Output? = null
private var uid: Output? = null
/**
* @param value APIGroup is the group for the resource being referenced. It is empty for the core API. This matches the group in the APIVersion that is used when creating the resources.
*/
@JvmName("yreujjpwwmwyjhkf")
public suspend fun apiGroup(`value`: Output) {
this.apiGroup = value
}
/**
* @param value Name is the name of resource being referenced.
*/
@JvmName("vqedqwxvjjdnehnd")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Resource is the type of resource being referenced, for example "pods".
*/
@JvmName("prbscdpircrygylr")
public suspend fun resource(`value`: Output) {
this.resource = value
}
/**
* @param value UID identifies exactly one incarnation of the resource.
*/
@JvmName("avkbsdhumakymvid")
public suspend fun uid(`value`: Output) {
this.uid = value
}
/**
* @param value APIGroup is the group for the resource being referenced. It is empty for the core API. This matches the group in the APIVersion that is used when creating the resources.
*/
@JvmName("lewvyyotcmuxvjww")
public suspend fun apiGroup(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiGroup = mapped
}
/**
* @param value Name is the name of resource being referenced.
*/
@JvmName("hdgrulxxaxatoken")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value Resource is the type of resource being referenced, for example "pods".
*/
@JvmName("gcirkjtbblgbqxwc")
public suspend fun resource(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.resource = mapped
}
/**
* @param value UID identifies exactly one incarnation of the resource.
*/
@JvmName("ipfypshyjtokaato")
public suspend fun uid(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.uid = mapped
}
internal fun build(): ResourceClaimConsumerReferenceArgs = ResourceClaimConsumerReferenceArgs(
apiGroup = apiGroup,
name = name ?: throw PulumiNullFieldException("name"),
resource = resource ?: throw PulumiNullFieldException("resource"),
uid = uid ?: throw PulumiNullFieldException("uid"),
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy