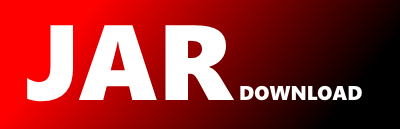
com.pulumi.kubernetes.resource.v1alpha2.kotlin.inputs.ResourceHandleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.resource.v1alpha2.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.kubernetes.resource.v1alpha2.inputs.ResourceHandleArgs.builder
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* ResourceHandle holds opaque resource data for processing by a specific kubelet plugin.
* @property data Data contains the opaque data associated with this ResourceHandle. It is set by the controller component of the resource driver whose name matches the DriverName set in the ResourceClaimStatus this ResourceHandle is embedded in. It is set at allocation time and is intended for processing by the kubelet plugin whose name matches the DriverName set in this ResourceHandle.
* The maximum size of this field is 16KiB. This may get increased in the future, but not reduced.
* @property driverName DriverName specifies the name of the resource driver whose kubelet plugin should be invoked to process this ResourceHandle's data once it lands on a node. This may differ from the DriverName set in ResourceClaimStatus this ResourceHandle is embedded in.
* @property structuredData If StructuredData is set, then it needs to be used instead of Data.
*/
public data class ResourceHandleArgs(
public val `data`: Output? = null,
public val driverName: Output? = null,
public val structuredData: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.resource.v1alpha2.inputs.ResourceHandleArgs =
com.pulumi.kubernetes.resource.v1alpha2.inputs.ResourceHandleArgs.builder()
.`data`(`data`?.applyValue({ args0 -> args0 }))
.driverName(driverName?.applyValue({ args0 -> args0 }))
.structuredData(
structuredData?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [ResourceHandleArgs].
*/
@PulumiTagMarker
public class ResourceHandleArgsBuilder internal constructor() {
private var `data`: Output? = null
private var driverName: Output? = null
private var structuredData: Output? = null
/**
* @param value Data contains the opaque data associated with this ResourceHandle. It is set by the controller component of the resource driver whose name matches the DriverName set in the ResourceClaimStatus this ResourceHandle is embedded in. It is set at allocation time and is intended for processing by the kubelet plugin whose name matches the DriverName set in this ResourceHandle.
* The maximum size of this field is 16KiB. This may get increased in the future, but not reduced.
*/
@JvmName("tmgryutgniubteci")
public suspend fun `data`(`value`: Output) {
this.`data` = value
}
/**
* @param value DriverName specifies the name of the resource driver whose kubelet plugin should be invoked to process this ResourceHandle's data once it lands on a node. This may differ from the DriverName set in ResourceClaimStatus this ResourceHandle is embedded in.
*/
@JvmName("klttcquvcrtoejky")
public suspend fun driverName(`value`: Output) {
this.driverName = value
}
/**
* @param value If StructuredData is set, then it needs to be used instead of Data.
*/
@JvmName("ronueqbirpgpdyjx")
public suspend fun structuredData(`value`: Output) {
this.structuredData = value
}
/**
* @param value Data contains the opaque data associated with this ResourceHandle. It is set by the controller component of the resource driver whose name matches the DriverName set in the ResourceClaimStatus this ResourceHandle is embedded in. It is set at allocation time and is intended for processing by the kubelet plugin whose name matches the DriverName set in this ResourceHandle.
* The maximum size of this field is 16KiB. This may get increased in the future, but not reduced.
*/
@JvmName("txyelalthxywyjed")
public suspend fun `data`(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.`data` = mapped
}
/**
* @param value DriverName specifies the name of the resource driver whose kubelet plugin should be invoked to process this ResourceHandle's data once it lands on a node. This may differ from the DriverName set in ResourceClaimStatus this ResourceHandle is embedded in.
*/
@JvmName("cuaxrylgskissruj")
public suspend fun driverName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.driverName = mapped
}
/**
* @param value If StructuredData is set, then it needs to be used instead of Data.
*/
@JvmName("xrwpfgdbspcbefil")
public suspend fun structuredData(`value`: StructuredResourceHandleArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.structuredData = mapped
}
/**
* @param argument If StructuredData is set, then it needs to be used instead of Data.
*/
@JvmName("etqxxlsbnixjjnqo")
public suspend fun structuredData(argument: suspend StructuredResourceHandleArgsBuilder.() -> Unit) {
val toBeMapped = StructuredResourceHandleArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.structuredData = mapped
}
internal fun build(): ResourceHandleArgs = ResourceHandleArgs(
`data` = `data`,
driverName = driverName,
structuredData = structuredData,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy