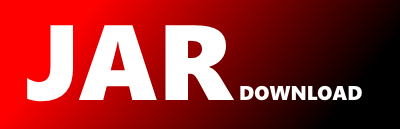
com.pulumi.kubernetes.resource.v1alpha3.kotlin.inputs.DeviceAttributeArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.resource.v1alpha3.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kubernetes.resource.v1alpha3.inputs.DeviceAttributeArgs.builder
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* DeviceAttribute must have exactly one field set.
* @property bool BoolValue is a true/false value.
* @property int IntValue is a number.
* @property string StringValue is a string. Must not be longer than 64 characters.
* @property version VersionValue is a semantic version according to semver.org spec 2.0.0. Must not be longer than 64 characters.
*/
public data class DeviceAttributeArgs(
public val bool: Output? = null,
public val int: Output? = null,
public val string: Output? = null,
public val version: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.resource.v1alpha3.inputs.DeviceAttributeArgs =
com.pulumi.kubernetes.resource.v1alpha3.inputs.DeviceAttributeArgs.builder()
.bool(bool?.applyValue({ args0 -> args0 }))
.int_(int?.applyValue({ args0 -> args0 }))
.string(string?.applyValue({ args0 -> args0 }))
.version(version?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DeviceAttributeArgs].
*/
@PulumiTagMarker
public class DeviceAttributeArgsBuilder internal constructor() {
private var bool: Output? = null
private var int: Output? = null
private var string: Output? = null
private var version: Output? = null
/**
* @param value BoolValue is a true/false value.
*/
@JvmName("kthgknqayqbiblaq")
public suspend fun bool(`value`: Output) {
this.bool = value
}
/**
* @param value IntValue is a number.
*/
@JvmName("raeexjysowmbbsfm")
public suspend fun int(`value`: Output) {
this.int = value
}
/**
* @param value StringValue is a string. Must not be longer than 64 characters.
*/
@JvmName("dykjpgbiiuphayqv")
public suspend fun string(`value`: Output) {
this.string = value
}
/**
* @param value VersionValue is a semantic version according to semver.org spec 2.0.0. Must not be longer than 64 characters.
*/
@JvmName("efyoeitdrohlskef")
public suspend fun version(`value`: Output) {
this.version = value
}
/**
* @param value BoolValue is a true/false value.
*/
@JvmName("nvnpfjiklbudjght")
public suspend fun bool(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bool = mapped
}
/**
* @param value IntValue is a number.
*/
@JvmName("gxcwuutarxkbvtno")
public suspend fun int(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.int = mapped
}
/**
* @param value StringValue is a string. Must not be longer than 64 characters.
*/
@JvmName("xbtmdpcmdsdtjyuk")
public suspend fun string(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.string = mapped
}
/**
* @param value VersionValue is a semantic version according to semver.org spec 2.0.0. Must not be longer than 64 characters.
*/
@JvmName("qhmmrxqkigmkkqvq")
public suspend fun version(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.version = mapped
}
internal fun build(): DeviceAttributeArgs = DeviceAttributeArgs(
bool = bool,
int = int,
string = string,
version = version,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy