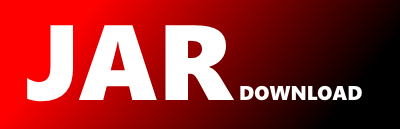
com.pulumi.kubernetes.scheduling.v1.kotlin.PriorityClassArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.scheduling.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.ObjectMetaArgs
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.ObjectMetaArgsBuilder
import com.pulumi.kubernetes.scheduling.v1.PriorityClassArgs.builder
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* PriorityClass defines mapping from a priority class name to the priority integer value. The value can be any valid integer.
* @property apiVersion APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
* @property description description is an arbitrary string that usually provides guidelines on when this priority class should be used.
* @property globalDefault globalDefault specifies whether this PriorityClass should be considered as the default priority for pods that do not have any priority class. Only one PriorityClass can be marked as `globalDefault`. However, if more than one PriorityClasses exists with their `globalDefault` field set to true, the smallest value of such global default PriorityClasses will be used as the default priority.
* @property kind Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
* @property metadata Standard object's metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
* @property preemptionPolicy preemptionPolicy is the Policy for preempting pods with lower priority. One of Never, PreemptLowerPriority. Defaults to PreemptLowerPriority if unset.
* @property value value represents the integer value of this priority class. This is the actual priority that pods receive when they have the name of this class in their pod spec.
*/
public data class PriorityClassArgs(
public val apiVersion: Output? = null,
public val description: Output? = null,
public val globalDefault: Output? = null,
public val kind: Output? = null,
public val metadata: Output? = null,
public val preemptionPolicy: Output? = null,
public val `value`: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.scheduling.v1.PriorityClassArgs =
com.pulumi.kubernetes.scheduling.v1.PriorityClassArgs.builder()
.apiVersion(apiVersion?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.globalDefault(globalDefault?.applyValue({ args0 -> args0 }))
.kind(kind?.applyValue({ args0 -> args0 }))
.metadata(metadata?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.preemptionPolicy(preemptionPolicy?.applyValue({ args0 -> args0 }))
.`value`(`value`?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [PriorityClassArgs].
*/
@PulumiTagMarker
public class PriorityClassArgsBuilder internal constructor() {
private var apiVersion: Output? = null
private var description: Output? = null
private var globalDefault: Output? = null
private var kind: Output? = null
private var metadata: Output? = null
private var preemptionPolicy: Output? = null
private var `value`: Output? = null
/**
* @param value APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*/
@JvmName("aubtrloviacrlibj")
public suspend fun apiVersion(`value`: Output) {
this.apiVersion = value
}
/**
* @param value description is an arbitrary string that usually provides guidelines on when this priority class should be used.
*/
@JvmName("snagbetwdxmevphu")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value globalDefault specifies whether this PriorityClass should be considered as the default priority for pods that do not have any priority class. Only one PriorityClass can be marked as `globalDefault`. However, if more than one PriorityClasses exists with their `globalDefault` field set to true, the smallest value of such global default PriorityClasses will be used as the default priority.
*/
@JvmName("rttgnddapkvfhvrx")
public suspend fun globalDefault(`value`: Output) {
this.globalDefault = value
}
/**
* @param value Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
@JvmName("xbllkvdrcacsytsy")
public suspend fun kind(`value`: Output) {
this.kind = value
}
/**
* @param value Standard object's metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
*/
@JvmName("oqgsbncbfswrpcry")
public suspend fun metadata(`value`: Output) {
this.metadata = value
}
/**
* @param value preemptionPolicy is the Policy for preempting pods with lower priority. One of Never, PreemptLowerPriority. Defaults to PreemptLowerPriority if unset.
*/
@JvmName("hepyyfbfqgwfvean")
public suspend fun preemptionPolicy(`value`: Output) {
this.preemptionPolicy = value
}
/**
* @param value value represents the integer value of this priority class. This is the actual priority that pods receive when they have the name of this class in their pod spec.
*/
@JvmName("phdqbgyinytkrhln")
public suspend fun `value`(`value`: Output) {
this.`value` = value
}
/**
* @param value APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*/
@JvmName("sbwvrtwglwnaalqm")
public suspend fun apiVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiVersion = mapped
}
/**
* @param value description is an arbitrary string that usually provides guidelines on when this priority class should be used.
*/
@JvmName("mdmdcjptgibnycet")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value globalDefault specifies whether this PriorityClass should be considered as the default priority for pods that do not have any priority class. Only one PriorityClass can be marked as `globalDefault`. However, if more than one PriorityClasses exists with their `globalDefault` field set to true, the smallest value of such global default PriorityClasses will be used as the default priority.
*/
@JvmName("jnblgrswiesdtnjr")
public suspend fun globalDefault(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.globalDefault = mapped
}
/**
* @param value Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
@JvmName("hxgllcaghkbpfbxu")
public suspend fun kind(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kind = mapped
}
/**
* @param value Standard object's metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
*/
@JvmName("nbdxgbvgphpgbigk")
public suspend fun metadata(`value`: ObjectMetaArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.metadata = mapped
}
/**
* @param argument Standard object's metadata. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
*/
@JvmName("vvytrckwusvixosw")
public suspend fun metadata(argument: suspend ObjectMetaArgsBuilder.() -> Unit) {
val toBeMapped = ObjectMetaArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.metadata = mapped
}
/**
* @param value preemptionPolicy is the Policy for preempting pods with lower priority. One of Never, PreemptLowerPriority. Defaults to PreemptLowerPriority if unset.
*/
@JvmName("lgfhaamspwkaknnd")
public suspend fun preemptionPolicy(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.preemptionPolicy = mapped
}
/**
* @param value value represents the integer value of this priority class. This is the actual priority that pods receive when they have the name of this class in their pod spec.
*/
@JvmName("cpjmgdjxwqfqbixl")
public suspend fun `value`(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.`value` = mapped
}
internal fun build(): PriorityClassArgs = PriorityClassArgs(
apiVersion = apiVersion,
description = description,
globalDefault = globalDefault,
kind = kind,
metadata = metadata,
preemptionPolicy = preemptionPolicy,
`value` = `value`,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy