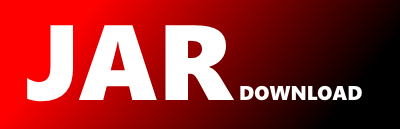
com.pulumi.kubernetes.storage.v1.kotlin.CSINodePatchArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
The newest version!
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.storage.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.ObjectMetaPatchArgs
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.ObjectMetaPatchArgsBuilder
import com.pulumi.kubernetes.storage.v1.CSINodePatchArgs.builder
import com.pulumi.kubernetes.storage.v1.kotlin.inputs.CSINodeSpecPatchArgs
import com.pulumi.kubernetes.storage.v1.kotlin.inputs.CSINodeSpecPatchArgsBuilder
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Patch resources are used to modify existing Kubernetes resources by using
* Server-Side Apply updates. The name of the resource must be specified, but all other properties are optional. More than
* one patch may be applied to the same resource, and a random FieldManager name will be used for each Patch resource.
* Conflicts will result in an error by default, but can be forced using the "pulumi.com/patchForce" annotation. See the
* [Server-Side Apply Docs](https://www.pulumi.com/registry/packages/kubernetes/how-to-guides/managing-resources-with-server-side-apply/) for
* additional information about using Server-Side Apply to manage Kubernetes resources with Pulumi.
* CSINode holds information about all CSI drivers installed on a node. CSI drivers do not need to create the CSINode object directly. As long as they use the node-driver-registrar sidecar container, the kubelet will automatically populate the CSINode object for the CSI driver as part of kubelet plugin registration. CSINode has the same name as a node. If the object is missing, it means either there are no CSI Drivers available on the node, or the Kubelet version is low enough that it doesn't create this object. CSINode has an OwnerReference that points to the corresponding node object.
* @property apiVersion APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
* @property kind Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
* @property metadata Standard object's metadata. metadata.name must be the Kubernetes node name.
* @property spec spec is the specification of CSINode
*/
public data class CSINodePatchArgs(
public val apiVersion: Output? = null,
public val kind: Output? = null,
public val metadata: Output? = null,
public val spec: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.storage.v1.CSINodePatchArgs =
com.pulumi.kubernetes.storage.v1.CSINodePatchArgs.builder()
.apiVersion(apiVersion?.applyValue({ args0 -> args0 }))
.kind(kind?.applyValue({ args0 -> args0 }))
.metadata(metadata?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.spec(spec?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [CSINodePatchArgs].
*/
@PulumiTagMarker
public class CSINodePatchArgsBuilder internal constructor() {
private var apiVersion: Output? = null
private var kind: Output? = null
private var metadata: Output? = null
private var spec: Output? = null
/**
* @param value APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*/
@JvmName("onrunrhnrpgevcpr")
public suspend fun apiVersion(`value`: Output) {
this.apiVersion = value
}
/**
* @param value Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
@JvmName("psulkmojfddhdqpa")
public suspend fun kind(`value`: Output) {
this.kind = value
}
/**
* @param value Standard object's metadata. metadata.name must be the Kubernetes node name.
*/
@JvmName("ffxngvgjfgkkruie")
public suspend fun metadata(`value`: Output) {
this.metadata = value
}
/**
* @param value spec is the specification of CSINode
*/
@JvmName("aheukjpdgcesvxuv")
public suspend fun spec(`value`: Output) {
this.spec = value
}
/**
* @param value APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*/
@JvmName("ujlagugsqmenvqlo")
public suspend fun apiVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiVersion = mapped
}
/**
* @param value Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
@JvmName("fiywcirbrjxewjiu")
public suspend fun kind(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kind = mapped
}
/**
* @param value Standard object's metadata. metadata.name must be the Kubernetes node name.
*/
@JvmName("kedxvhsvhikundim")
public suspend fun metadata(`value`: ObjectMetaPatchArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.metadata = mapped
}
/**
* @param argument Standard object's metadata. metadata.name must be the Kubernetes node name.
*/
@JvmName("kvigfjqerlyulxnx")
public suspend fun metadata(argument: suspend ObjectMetaPatchArgsBuilder.() -> Unit) {
val toBeMapped = ObjectMetaPatchArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.metadata = mapped
}
/**
* @param value spec is the specification of CSINode
*/
@JvmName("cfudydnqvmclclre")
public suspend fun spec(`value`: CSINodeSpecPatchArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.spec = mapped
}
/**
* @param argument spec is the specification of CSINode
*/
@JvmName("csggcivfvrobajtg")
public suspend fun spec(argument: suspend CSINodeSpecPatchArgsBuilder.() -> Unit) {
val toBeMapped = CSINodeSpecPatchArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.spec = mapped
}
internal fun build(): CSINodePatchArgs = CSINodePatchArgs(
apiVersion = apiVersion,
kind = kind,
metadata = metadata,
spec = spec,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy