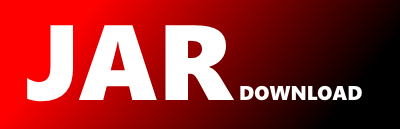
com.pulumi.kubernetes.apps.v1beta1.kotlin.inputs.StatefulSetArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.apps.v1beta1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.kubernetes.apps.v1beta1.inputs.StatefulSetArgs.builder
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.ObjectMetaArgs
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.ObjectMetaArgsBuilder
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* StatefulSet represents a set of pods with consistent identities. Identities are defined as:
* - Network: A single stable DNS and hostname.
* - Storage: As many VolumeClaims as requested.
* The StatefulSet guarantees that a given network identity will always map to the same storage identity.
* This resource waits until its status is ready before registering success
* for create/update, and populating output properties from the current state of the resource.
* The following conditions are used to determine whether the resource creation has
* succeeded or failed:
* 1. The value of 'spec.replicas' matches '.status.replicas', '.status.currentReplicas',
* and '.status.readyReplicas'.
* 2. The value of '.status.updateRevision' matches '.status.currentRevision'.
* If the StatefulSet has not reached a Ready state after 10 minutes, it will
* time out and mark the resource update as Failed. You can override the default timeout value
* by setting the 'customTimeouts' option on the resource.
* @property apiVersion APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
* @property kind Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
* @property metadata
* @property spec Spec defines the desired identities of pods in this set.
* @property status Status is the current status of Pods in this StatefulSet. This data may be out of date by some window of time.
*/
public data class StatefulSetArgs(
public val apiVersion: Output? = null,
public val kind: Output? = null,
public val metadata: Output? = null,
public val spec: Output? = null,
public val status: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.apps.v1beta1.inputs.StatefulSetArgs =
com.pulumi.kubernetes.apps.v1beta1.inputs.StatefulSetArgs.builder()
.apiVersion(apiVersion?.applyValue({ args0 -> args0 }))
.kind(kind?.applyValue({ args0 -> args0 }))
.metadata(metadata?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.spec(spec?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.status(status?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [StatefulSetArgs].
*/
@PulumiTagMarker
public class StatefulSetArgsBuilder internal constructor() {
private var apiVersion: Output? = null
private var kind: Output? = null
private var metadata: Output? = null
private var spec: Output? = null
private var status: Output? = null
/**
* @param value APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*/
@JvmName("djfrwurhqifibnre")
public suspend fun apiVersion(`value`: Output) {
this.apiVersion = value
}
/**
* @param value Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
@JvmName("ctsbrkdewafxiado")
public suspend fun kind(`value`: Output) {
this.kind = value
}
/**
* @param value
*/
@JvmName("uycbbvrcpsxtyenn")
public suspend fun metadata(`value`: Output) {
this.metadata = value
}
/**
* @param value Spec defines the desired identities of pods in this set.
*/
@JvmName("naefuftptkraladv")
public suspend fun spec(`value`: Output) {
this.spec = value
}
/**
* @param value Status is the current status of Pods in this StatefulSet. This data may be out of date by some window of time.
*/
@JvmName("ngvirljarmgbayvp")
public suspend fun status(`value`: Output) {
this.status = value
}
/**
* @param value APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*/
@JvmName("jhlpbemuqmourkwt")
public suspend fun apiVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiVersion = mapped
}
/**
* @param value Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
@JvmName("pltjetjginoxjcqv")
public suspend fun kind(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kind = mapped
}
/**
* @param value
*/
@JvmName("glrmykvnwuvisvfe")
public suspend fun metadata(`value`: ObjectMetaArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.metadata = mapped
}
/**
* @param argument
*/
@JvmName("vhyainrrirfjhdic")
public suspend fun metadata(argument: suspend ObjectMetaArgsBuilder.() -> Unit) {
val toBeMapped = ObjectMetaArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.metadata = mapped
}
/**
* @param value Spec defines the desired identities of pods in this set.
*/
@JvmName("ntbitninjfelaxid")
public suspend fun spec(`value`: StatefulSetSpecArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.spec = mapped
}
/**
* @param argument Spec defines the desired identities of pods in this set.
*/
@JvmName("ujevybjjwmadtinp")
public suspend fun spec(argument: suspend StatefulSetSpecArgsBuilder.() -> Unit) {
val toBeMapped = StatefulSetSpecArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.spec = mapped
}
/**
* @param value Status is the current status of Pods in this StatefulSet. This data may be out of date by some window of time.
*/
@JvmName("dcjdgmhicvbyafdq")
public suspend fun status(`value`: StatefulSetStatusArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.status = mapped
}
/**
* @param argument Status is the current status of Pods in this StatefulSet. This data may be out of date by some window of time.
*/
@JvmName("xjpwxahnpmtuftxb")
public suspend fun status(argument: suspend StatefulSetStatusArgsBuilder.() -> Unit) {
val toBeMapped = StatefulSetStatusArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.status = mapped
}
internal fun build(): StatefulSetArgs = StatefulSetArgs(
apiVersion = apiVersion,
kind = kind,
metadata = metadata,
spec = spec,
status = status,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy