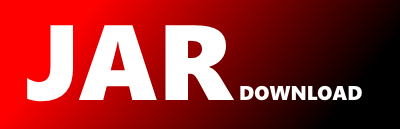
com.pulumi.kubernetes.apps.v1beta2.kotlin.inputs.DaemonSetStatusArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.apps.v1beta2.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.kubernetes.apps.v1beta2.inputs.DaemonSetStatusArgs.builder
import kotlin.Int
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* DaemonSetStatus represents the current status of a daemon set.
* @property collisionCount Count of hash collisions for the DaemonSet. The DaemonSet controller uses this field as a collision avoidance mechanism when it needs to create the name for the newest ControllerRevision.
* @property conditions Represents the latest available observations of a DaemonSet's current state.
* @property currentNumberScheduled The number of nodes that are running at least 1 daemon pod and are supposed to run the daemon pod. More info: https://kubernetes.io/docs/concepts/workloads/controllers/daemonset/
* @property desiredNumberScheduled The total number of nodes that should be running the daemon pod (including nodes correctly running the daemon pod). More info: https://kubernetes.io/docs/concepts/workloads/controllers/daemonset/
* @property numberAvailable The number of nodes that should be running the daemon pod and have one or more of the daemon pod running and available (ready for at least spec.minReadySeconds)
* @property numberMisscheduled The number of nodes that are running the daemon pod, but are not supposed to run the daemon pod. More info: https://kubernetes.io/docs/concepts/workloads/controllers/daemonset/
* @property numberReady The number of nodes that should be running the daemon pod and have one or more of the daemon pod running and ready.
* @property numberUnavailable The number of nodes that should be running the daemon pod and have none of the daemon pod running and available (ready for at least spec.minReadySeconds)
* @property observedGeneration The most recent generation observed by the daemon set controller.
* @property updatedNumberScheduled The total number of nodes that are running updated daemon pod
*/
public data class DaemonSetStatusArgs(
public val collisionCount: Output? = null,
public val conditions: Output>? = null,
public val currentNumberScheduled: Output,
public val desiredNumberScheduled: Output,
public val numberAvailable: Output? = null,
public val numberMisscheduled: Output,
public val numberReady: Output,
public val numberUnavailable: Output? = null,
public val observedGeneration: Output? = null,
public val updatedNumberScheduled: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.apps.v1beta2.inputs.DaemonSetStatusArgs =
com.pulumi.kubernetes.apps.v1beta2.inputs.DaemonSetStatusArgs.builder()
.collisionCount(collisionCount?.applyValue({ args0 -> args0 }))
.conditions(
conditions?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.currentNumberScheduled(currentNumberScheduled.applyValue({ args0 -> args0 }))
.desiredNumberScheduled(desiredNumberScheduled.applyValue({ args0 -> args0 }))
.numberAvailable(numberAvailable?.applyValue({ args0 -> args0 }))
.numberMisscheduled(numberMisscheduled.applyValue({ args0 -> args0 }))
.numberReady(numberReady.applyValue({ args0 -> args0 }))
.numberUnavailable(numberUnavailable?.applyValue({ args0 -> args0 }))
.observedGeneration(observedGeneration?.applyValue({ args0 -> args0 }))
.updatedNumberScheduled(updatedNumberScheduled?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DaemonSetStatusArgs].
*/
@PulumiTagMarker
public class DaemonSetStatusArgsBuilder internal constructor() {
private var collisionCount: Output? = null
private var conditions: Output>? = null
private var currentNumberScheduled: Output? = null
private var desiredNumberScheduled: Output? = null
private var numberAvailable: Output? = null
private var numberMisscheduled: Output? = null
private var numberReady: Output? = null
private var numberUnavailable: Output? = null
private var observedGeneration: Output? = null
private var updatedNumberScheduled: Output? = null
/**
* @param value Count of hash collisions for the DaemonSet. The DaemonSet controller uses this field as a collision avoidance mechanism when it needs to create the name for the newest ControllerRevision.
*/
@JvmName("ctgbrvpdbvqxohht")
public suspend fun collisionCount(`value`: Output) {
this.collisionCount = value
}
/**
* @param value Represents the latest available observations of a DaemonSet's current state.
*/
@JvmName("vjhpukaqlbcmlgqr")
public suspend fun conditions(`value`: Output>) {
this.conditions = value
}
@JvmName("qphpdufgqcdgbebm")
public suspend fun conditions(vararg values: Output) {
this.conditions = Output.all(values.asList())
}
/**
* @param values Represents the latest available observations of a DaemonSet's current state.
*/
@JvmName("wwhxgsjyxxubstvu")
public suspend fun conditions(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy