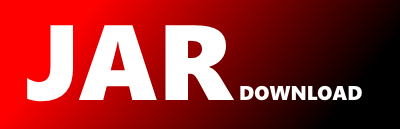
com.pulumi.kubernetes.autoscaling.v2.kotlin.inputs.MetricTargetArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.autoscaling.v2.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kubernetes.autoscaling.v2.inputs.MetricTargetArgs.builder
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* MetricTarget defines the target value, average value, or average utilization of a specific metric
* @property averageUtilization averageUtilization is the target value of the average of the resource metric across all relevant pods, represented as a percentage of the requested value of the resource for the pods. Currently only valid for Resource metric source type
* @property averageValue averageValue is the target value of the average of the metric across all relevant pods (as a quantity)
* @property type type represents whether the metric type is Utilization, Value, or AverageValue
* @property value value is the target value of the metric (as a quantity).
*/
public data class MetricTargetArgs(
public val averageUtilization: Output? = null,
public val averageValue: Output? = null,
public val type: Output,
public val `value`: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.autoscaling.v2.inputs.MetricTargetArgs =
com.pulumi.kubernetes.autoscaling.v2.inputs.MetricTargetArgs.builder()
.averageUtilization(averageUtilization?.applyValue({ args0 -> args0 }))
.averageValue(averageValue?.applyValue({ args0 -> args0 }))
.type(type.applyValue({ args0 -> args0 }))
.`value`(`value`?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [MetricTargetArgs].
*/
@PulumiTagMarker
public class MetricTargetArgsBuilder internal constructor() {
private var averageUtilization: Output? = null
private var averageValue: Output? = null
private var type: Output? = null
private var `value`: Output? = null
/**
* @param value averageUtilization is the target value of the average of the resource metric across all relevant pods, represented as a percentage of the requested value of the resource for the pods. Currently only valid for Resource metric source type
*/
@JvmName("xbcrekpunkqicugu")
public suspend fun averageUtilization(`value`: Output) {
this.averageUtilization = value
}
/**
* @param value averageValue is the target value of the average of the metric across all relevant pods (as a quantity)
*/
@JvmName("mtbrndqaridvefyj")
public suspend fun averageValue(`value`: Output) {
this.averageValue = value
}
/**
* @param value type represents whether the metric type is Utilization, Value, or AverageValue
*/
@JvmName("owxavxpelldhlsix")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value value is the target value of the metric (as a quantity).
*/
@JvmName("rwbohxgcrhdwmspj")
public suspend fun `value`(`value`: Output) {
this.`value` = value
}
/**
* @param value averageUtilization is the target value of the average of the resource metric across all relevant pods, represented as a percentage of the requested value of the resource for the pods. Currently only valid for Resource metric source type
*/
@JvmName("lqhhjrpluidjmqrg")
public suspend fun averageUtilization(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.averageUtilization = mapped
}
/**
* @param value averageValue is the target value of the average of the metric across all relevant pods (as a quantity)
*/
@JvmName("mnrsaliywoaliahc")
public suspend fun averageValue(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.averageValue = mapped
}
/**
* @param value type represents whether the metric type is Utilization, Value, or AverageValue
*/
@JvmName("ujnajybqjhgmlhau")
public suspend fun type(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.type = mapped
}
/**
* @param value value is the target value of the metric (as a quantity).
*/
@JvmName("bhwspkcwphuyqlyi")
public suspend fun `value`(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.`value` = mapped
}
internal fun build(): MetricTargetArgs = MetricTargetArgs(
averageUtilization = averageUtilization,
averageValue = averageValue,
type = type ?: throw PulumiNullFieldException("type"),
`value` = `value`,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy