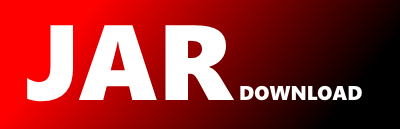
com.pulumi.kubernetes.autoscaling.v2beta1.kotlin.inputs.ExternalMetricStatusArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.autoscaling.v2beta1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.kubernetes.autoscaling.v2beta1.inputs.ExternalMetricStatusArgs.builder
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.LabelSelectorArgs
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.LabelSelectorArgsBuilder
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* ExternalMetricStatus indicates the current value of a global metric not associated with any Kubernetes object.
* @property currentAverageValue currentAverageValue is the current value of metric averaged over autoscaled pods.
* @property currentValue currentValue is the current value of the metric (as a quantity)
* @property metricName metricName is the name of a metric used for autoscaling in metric system.
* @property metricSelector metricSelector is used to identify a specific time series within a given metric.
*/
public data class ExternalMetricStatusArgs(
public val currentAverageValue: Output? = null,
public val currentValue: Output,
public val metricName: Output,
public val metricSelector: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.autoscaling.v2beta1.inputs.ExternalMetricStatusArgs =
com.pulumi.kubernetes.autoscaling.v2beta1.inputs.ExternalMetricStatusArgs.builder()
.currentAverageValue(currentAverageValue?.applyValue({ args0 -> args0 }))
.currentValue(currentValue.applyValue({ args0 -> args0 }))
.metricName(metricName.applyValue({ args0 -> args0 }))
.metricSelector(
metricSelector?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [ExternalMetricStatusArgs].
*/
@PulumiTagMarker
public class ExternalMetricStatusArgsBuilder internal constructor() {
private var currentAverageValue: Output? = null
private var currentValue: Output? = null
private var metricName: Output? = null
private var metricSelector: Output? = null
/**
* @param value currentAverageValue is the current value of metric averaged over autoscaled pods.
*/
@JvmName("tddxfyvlbtsxspju")
public suspend fun currentAverageValue(`value`: Output) {
this.currentAverageValue = value
}
/**
* @param value currentValue is the current value of the metric (as a quantity)
*/
@JvmName("rsefseddsssulyrq")
public suspend fun currentValue(`value`: Output) {
this.currentValue = value
}
/**
* @param value metricName is the name of a metric used for autoscaling in metric system.
*/
@JvmName("qrnnvgnrurlcfrpj")
public suspend fun metricName(`value`: Output) {
this.metricName = value
}
/**
* @param value metricSelector is used to identify a specific time series within a given metric.
*/
@JvmName("kluvkdccatbfqvpi")
public suspend fun metricSelector(`value`: Output) {
this.metricSelector = value
}
/**
* @param value currentAverageValue is the current value of metric averaged over autoscaled pods.
*/
@JvmName("wmaqbihrohayslse")
public suspend fun currentAverageValue(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.currentAverageValue = mapped
}
/**
* @param value currentValue is the current value of the metric (as a quantity)
*/
@JvmName("xobjnaaijptkweht")
public suspend fun currentValue(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.currentValue = mapped
}
/**
* @param value metricName is the name of a metric used for autoscaling in metric system.
*/
@JvmName("gbngywktuudfmmmh")
public suspend fun metricName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.metricName = mapped
}
/**
* @param value metricSelector is used to identify a specific time series within a given metric.
*/
@JvmName("fhiyfajdvrkuhwfy")
public suspend fun metricSelector(`value`: LabelSelectorArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.metricSelector = mapped
}
/**
* @param argument metricSelector is used to identify a specific time series within a given metric.
*/
@JvmName("syqkxmjqukdvnnfy")
public suspend fun metricSelector(argument: suspend LabelSelectorArgsBuilder.() -> Unit) {
val toBeMapped = LabelSelectorArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.metricSelector = mapped
}
internal fun build(): ExternalMetricStatusArgs = ExternalMetricStatusArgs(
currentAverageValue = currentAverageValue,
currentValue = currentValue ?: throw PulumiNullFieldException("currentValue"),
metricName = metricName ?: throw PulumiNullFieldException("metricName"),
metricSelector = metricSelector,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy