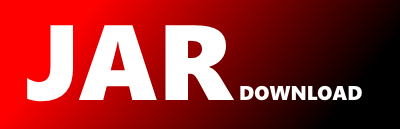
com.pulumi.kubernetes.discovery.v1.kotlin.inputs.EndpointSliceArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.discovery.v1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.kubernetes.discovery.v1.inputs.EndpointSliceArgs.builder
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.ObjectMetaArgs
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.ObjectMetaArgsBuilder
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* EndpointSlice represents a subset of the endpoints that implement a service. For a given service there may be multiple EndpointSlice objects, selected by labels, which must be joined to produce the full set of endpoints.
* @property addressType addressType specifies the type of address carried by this EndpointSlice. All addresses in this slice must be the same type. This field is immutable after creation. The following address types are currently supported: * IPv4: Represents an IPv4 Address. * IPv6: Represents an IPv6 Address. * FQDN: Represents a Fully Qualified Domain Name.
* @property apiVersion APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
* @property endpoints endpoints is a list of unique endpoints in this slice. Each slice may include a maximum of 1000 endpoints.
* @property kind Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
* @property metadata Standard object's metadata.
* @property ports ports specifies the list of network ports exposed by each endpoint in this slice. Each port must have a unique name. When ports is empty, it indicates that there are no defined ports. When a port is defined with a nil port value, it indicates "all ports". Each slice may include a maximum of 100 ports.
*/
public data class EndpointSliceArgs(
public val addressType: Output,
public val apiVersion: Output? = null,
public val endpoints: Output>,
public val kind: Output? = null,
public val metadata: Output? = null,
public val ports: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.discovery.v1.inputs.EndpointSliceArgs =
com.pulumi.kubernetes.discovery.v1.inputs.EndpointSliceArgs.builder()
.addressType(addressType.applyValue({ args0 -> args0 }))
.apiVersion(apiVersion?.applyValue({ args0 -> args0 }))
.endpoints(
endpoints.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.kind(kind?.applyValue({ args0 -> args0 }))
.metadata(metadata?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.ports(
ports?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [EndpointSliceArgs].
*/
@PulumiTagMarker
public class EndpointSliceArgsBuilder internal constructor() {
private var addressType: Output? = null
private var apiVersion: Output? = null
private var endpoints: Output>? = null
private var kind: Output? = null
private var metadata: Output? = null
private var ports: Output>? = null
/**
* @param value addressType specifies the type of address carried by this EndpointSlice. All addresses in this slice must be the same type. This field is immutable after creation. The following address types are currently supported: * IPv4: Represents an IPv4 Address. * IPv6: Represents an IPv6 Address. * FQDN: Represents a Fully Qualified Domain Name.
*/
@JvmName("rrosptdmqmfnpchf")
public suspend fun addressType(`value`: Output) {
this.addressType = value
}
/**
* @param value APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*/
@JvmName("mjeqevptyedtguxp")
public suspend fun apiVersion(`value`: Output) {
this.apiVersion = value
}
/**
* @param value endpoints is a list of unique endpoints in this slice. Each slice may include a maximum of 1000 endpoints.
*/
@JvmName("qfuacjnngwcyqrvh")
public suspend fun endpoints(`value`: Output>) {
this.endpoints = value
}
@JvmName("wjqxooybpkbctytb")
public suspend fun endpoints(vararg values: Output) {
this.endpoints = Output.all(values.asList())
}
/**
* @param values endpoints is a list of unique endpoints in this slice. Each slice may include a maximum of 1000 endpoints.
*/
@JvmName("bibevidtgicdoqlt")
public suspend fun endpoints(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy