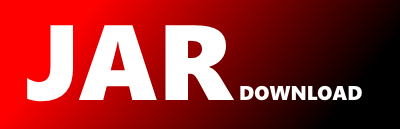
com.pulumi.kubernetes.kotlin.ProviderArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.kubernetes.ProviderArgs.builder
import com.pulumi.kubernetes.kotlin.inputs.HelmReleaseSettingsArgs
import com.pulumi.kubernetes.kotlin.inputs.HelmReleaseSettingsArgsBuilder
import com.pulumi.kubernetes.kotlin.inputs.KubeClientSettingsArgs
import com.pulumi.kubernetes.kotlin.inputs.KubeClientSettingsArgsBuilder
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* The provider type for the kubernetes package.
* @property cluster If present, the name of the kubeconfig cluster to use.
* @property clusterIdentifier If present, this value will control the provider's replacement behavior. In particular, the provider will _only_ be replaced when `clusterIdentifier` changes; all other changes to provider configuration will be treated as updates.
* Kubernetes does not yet offer an API for cluster identification, so Pulumi uses heuristics to decide when a provider resource should be replaced or updated. These heuristics can sometimes lead to destructive replace operations when an update would be more appropriate, or vice versa.
* Use `clusterIdentifier` for more fine-grained control of the provider resource's lifecycle.
* @property context If present, the name of the kubeconfig context to use.
* @property deleteUnreachable If present and set to true, the provider will delete resources associated with an unreachable Kubernetes cluster from Pulumi state
* @property enableConfigMapMutable BETA FEATURE - If present and set to true, allow ConfigMaps to be mutated.
* This feature is in developer preview, and is disabled by default.
* This config can be specified in the following ways using this precedence:
* 1. This `enableConfigMapMutable` parameter.
* 2. The `PULUMI_K8S_ENABLE_CONFIGMAP_MUTABLE` environment variable.
* @property enableSecretMutable BETA FEATURE - If present and set to true, allow Secrets to be mutated.
* This feature is in developer preview, and is disabled by default.
* This config can be specified in the following ways using this precedence:
* 1. This `enableSecretMutable` parameter.
* 2. The `PULUMI_K8S_ENABLE_SECRET_MUTABLE` environment variable.
* @property enableServerSideApply If present and set to false, disable Server-Side Apply mode.
* See https://github.com/pulumi/pulumi-kubernetes/issues/2011 for additional details.
* @property helmReleaseSettings Options to configure the Helm Release resource.
* @property kubeClientSettings Options for tuning the Kubernetes client used by a Provider.
* @property kubeconfig The contents of a kubeconfig file or the path to a kubeconfig file.
* @property namespace If present, the default namespace to use. This flag is ignored for cluster-scoped resources.
* A namespace can be specified in multiple places, and the precedence is as follows:
* 1. `.metadata.namespace` set on the resource.
* 2. This `namespace` parameter.
* 3. `namespace` set for the active context in the kubeconfig.
* @property renderYamlToDirectory BETA FEATURE - If present, render resource manifests to this directory. In this mode, resources will not
* be created on a Kubernetes cluster, but the rendered manifests will be kept in sync with changes
* to the Pulumi program. This feature is in developer preview, and is disabled by default.
* Note that some computed Outputs such as status fields will not be populated
* since the resources are not created on a Kubernetes cluster. These Output values will remain undefined,
* and may result in an error if they are referenced by other resources. Also note that any secret values
* used in these resources will be rendered in plaintext to the resulting YAML.
* @property skipUpdateUnreachable If present and set to true, the provider will skip resources update associated with an unreachable Kubernetes cluster from Pulumi state
* @property suppressDeprecationWarnings If present and set to true, suppress apiVersion deprecation warnings from the CLI.
* @property suppressHelmHookWarnings If present and set to true, suppress unsupported Helm hook warnings from the CLI.
*/
public data class ProviderArgs(
public val cluster: Output? = null,
public val clusterIdentifier: Output? = null,
public val context: Output? = null,
public val deleteUnreachable: Output? = null,
public val enableConfigMapMutable: Output? = null,
public val enableSecretMutable: Output? = null,
public val enableServerSideApply: Output? = null,
public val helmReleaseSettings: Output? = null,
public val kubeClientSettings: Output? = null,
public val kubeconfig: Output? = null,
public val namespace: Output? = null,
public val renderYamlToDirectory: Output? = null,
public val skipUpdateUnreachable: Output? = null,
public val suppressDeprecationWarnings: Output? = null,
public val suppressHelmHookWarnings: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.ProviderArgs =
com.pulumi.kubernetes.ProviderArgs.builder()
.cluster(cluster?.applyValue({ args0 -> args0 }))
.clusterIdentifier(clusterIdentifier?.applyValue({ args0 -> args0 }))
.context(context?.applyValue({ args0 -> args0 }))
.deleteUnreachable(deleteUnreachable?.applyValue({ args0 -> args0 }))
.enableConfigMapMutable(enableConfigMapMutable?.applyValue({ args0 -> args0 }))
.enableSecretMutable(enableSecretMutable?.applyValue({ args0 -> args0 }))
.enableServerSideApply(enableServerSideApply?.applyValue({ args0 -> args0 }))
.helmReleaseSettings(
helmReleaseSettings?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.kubeClientSettings(
kubeClientSettings?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.kubeconfig(kubeconfig?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.renderYamlToDirectory(renderYamlToDirectory?.applyValue({ args0 -> args0 }))
.skipUpdateUnreachable(skipUpdateUnreachable?.applyValue({ args0 -> args0 }))
.suppressDeprecationWarnings(suppressDeprecationWarnings?.applyValue({ args0 -> args0 }))
.suppressHelmHookWarnings(suppressHelmHookWarnings?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ProviderArgs].
*/
@PulumiTagMarker
public class ProviderArgsBuilder internal constructor() {
private var cluster: Output? = null
private var clusterIdentifier: Output? = null
private var context: Output? = null
private var deleteUnreachable: Output? = null
private var enableConfigMapMutable: Output? = null
private var enableSecretMutable: Output? = null
private var enableServerSideApply: Output? = null
private var helmReleaseSettings: Output? = null
private var kubeClientSettings: Output? = null
private var kubeconfig: Output? = null
private var namespace: Output? = null
private var renderYamlToDirectory: Output? = null
private var skipUpdateUnreachable: Output? = null
private var suppressDeprecationWarnings: Output? = null
private var suppressHelmHookWarnings: Output? = null
/**
* @param value If present, the name of the kubeconfig cluster to use.
*/
@JvmName("civdvuwppstspjso")
public suspend fun cluster(`value`: Output) {
this.cluster = value
}
/**
* @param value If present, this value will control the provider's replacement behavior. In particular, the provider will _only_ be replaced when `clusterIdentifier` changes; all other changes to provider configuration will be treated as updates.
* Kubernetes does not yet offer an API for cluster identification, so Pulumi uses heuristics to decide when a provider resource should be replaced or updated. These heuristics can sometimes lead to destructive replace operations when an update would be more appropriate, or vice versa.
* Use `clusterIdentifier` for more fine-grained control of the provider resource's lifecycle.
*/
@JvmName("jbouwmrgqpuleugc")
public suspend fun clusterIdentifier(`value`: Output) {
this.clusterIdentifier = value
}
/**
* @param value If present, the name of the kubeconfig context to use.
*/
@JvmName("kmuetaulnrpfiwma")
public suspend fun context(`value`: Output) {
this.context = value
}
/**
* @param value If present and set to true, the provider will delete resources associated with an unreachable Kubernetes cluster from Pulumi state
*/
@JvmName("wemolfhvqbnllrlv")
public suspend fun deleteUnreachable(`value`: Output) {
this.deleteUnreachable = value
}
/**
* @param value BETA FEATURE - If present and set to true, allow ConfigMaps to be mutated.
* This feature is in developer preview, and is disabled by default.
* This config can be specified in the following ways using this precedence:
* 1. This `enableConfigMapMutable` parameter.
* 2. The `PULUMI_K8S_ENABLE_CONFIGMAP_MUTABLE` environment variable.
*/
@JvmName("feuepvtqxtllwppf")
public suspend fun enableConfigMapMutable(`value`: Output) {
this.enableConfigMapMutable = value
}
/**
* @param value BETA FEATURE - If present and set to true, allow Secrets to be mutated.
* This feature is in developer preview, and is disabled by default.
* This config can be specified in the following ways using this precedence:
* 1. This `enableSecretMutable` parameter.
* 2. The `PULUMI_K8S_ENABLE_SECRET_MUTABLE` environment variable.
*/
@JvmName("xmeryqdwpkjwtqxi")
public suspend fun enableSecretMutable(`value`: Output) {
this.enableSecretMutable = value
}
/**
* @param value If present and set to false, disable Server-Side Apply mode.
* See https://github.com/pulumi/pulumi-kubernetes/issues/2011 for additional details.
*/
@JvmName("okfsobcaqbmbkqys")
public suspend fun enableServerSideApply(`value`: Output) {
this.enableServerSideApply = value
}
/**
* @param value Options to configure the Helm Release resource.
*/
@JvmName("qgwvibctommuwolx")
public suspend fun helmReleaseSettings(`value`: Output) {
this.helmReleaseSettings = value
}
/**
* @param value Options for tuning the Kubernetes client used by a Provider.
*/
@JvmName("sxnsopvmqdessape")
public suspend fun kubeClientSettings(`value`: Output) {
this.kubeClientSettings = value
}
/**
* @param value The contents of a kubeconfig file or the path to a kubeconfig file.
*/
@JvmName("rgojgdbirhupqapl")
public suspend fun kubeconfig(`value`: Output) {
this.kubeconfig = value
}
/**
* @param value If present, the default namespace to use. This flag is ignored for cluster-scoped resources.
* A namespace can be specified in multiple places, and the precedence is as follows:
* 1. `.metadata.namespace` set on the resource.
* 2. This `namespace` parameter.
* 3. `namespace` set for the active context in the kubeconfig.
*/
@JvmName("edhuckplnpmteico")
public suspend fun namespace(`value`: Output) {
this.namespace = value
}
/**
* @param value BETA FEATURE - If present, render resource manifests to this directory. In this mode, resources will not
* be created on a Kubernetes cluster, but the rendered manifests will be kept in sync with changes
* to the Pulumi program. This feature is in developer preview, and is disabled by default.
* Note that some computed Outputs such as status fields will not be populated
* since the resources are not created on a Kubernetes cluster. These Output values will remain undefined,
* and may result in an error if they are referenced by other resources. Also note that any secret values
* used in these resources will be rendered in plaintext to the resulting YAML.
*/
@JvmName("lrneyrxdydmglums")
public suspend fun renderYamlToDirectory(`value`: Output) {
this.renderYamlToDirectory = value
}
/**
* @param value If present and set to true, the provider will skip resources update associated with an unreachable Kubernetes cluster from Pulumi state
*/
@JvmName("rkssluhikrtgdhhj")
public suspend fun skipUpdateUnreachable(`value`: Output) {
this.skipUpdateUnreachable = value
}
/**
* @param value If present and set to true, suppress apiVersion deprecation warnings from the CLI.
*/
@JvmName("cuqubqvtxocymfjo")
public suspend fun suppressDeprecationWarnings(`value`: Output) {
this.suppressDeprecationWarnings = value
}
/**
* @param value If present and set to true, suppress unsupported Helm hook warnings from the CLI.
*/
@JvmName("iyuupjtwwonpfbqw")
public suspend fun suppressHelmHookWarnings(`value`: Output) {
this.suppressHelmHookWarnings = value
}
/**
* @param value If present, the name of the kubeconfig cluster to use.
*/
@JvmName("idnfnvcnyyhorhir")
public suspend fun cluster(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.cluster = mapped
}
/**
* @param value If present, this value will control the provider's replacement behavior. In particular, the provider will _only_ be replaced when `clusterIdentifier` changes; all other changes to provider configuration will be treated as updates.
* Kubernetes does not yet offer an API for cluster identification, so Pulumi uses heuristics to decide when a provider resource should be replaced or updated. These heuristics can sometimes lead to destructive replace operations when an update would be more appropriate, or vice versa.
* Use `clusterIdentifier` for more fine-grained control of the provider resource's lifecycle.
*/
@JvmName("uduouvsdhekmgtmc")
public suspend fun clusterIdentifier(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.clusterIdentifier = mapped
}
/**
* @param value If present, the name of the kubeconfig context to use.
*/
@JvmName("wpiffwkpioidesqq")
public suspend fun context(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.context = mapped
}
/**
* @param value If present and set to true, the provider will delete resources associated with an unreachable Kubernetes cluster from Pulumi state
*/
@JvmName("oiiebdftwcpcpnee")
public suspend fun deleteUnreachable(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deleteUnreachable = mapped
}
/**
* @param value BETA FEATURE - If present and set to true, allow ConfigMaps to be mutated.
* This feature is in developer preview, and is disabled by default.
* This config can be specified in the following ways using this precedence:
* 1. This `enableConfigMapMutable` parameter.
* 2. The `PULUMI_K8S_ENABLE_CONFIGMAP_MUTABLE` environment variable.
*/
@JvmName("mmtjcwefpynprwgj")
public suspend fun enableConfigMapMutable(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableConfigMapMutable = mapped
}
/**
* @param value BETA FEATURE - If present and set to true, allow Secrets to be mutated.
* This feature is in developer preview, and is disabled by default.
* This config can be specified in the following ways using this precedence:
* 1. This `enableSecretMutable` parameter.
* 2. The `PULUMI_K8S_ENABLE_SECRET_MUTABLE` environment variable.
*/
@JvmName("jvcsehyqsjhfeibq")
public suspend fun enableSecretMutable(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableSecretMutable = mapped
}
/**
* @param value If present and set to false, disable Server-Side Apply mode.
* See https://github.com/pulumi/pulumi-kubernetes/issues/2011 for additional details.
*/
@JvmName("ypoiauqksaptgisg")
public suspend fun enableServerSideApply(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.enableServerSideApply = mapped
}
/**
* @param value Options to configure the Helm Release resource.
*/
@JvmName("bfuxtvjeobepsrfp")
public suspend fun helmReleaseSettings(`value`: HelmReleaseSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.helmReleaseSettings = mapped
}
/**
* @param argument Options to configure the Helm Release resource.
*/
@JvmName("awqilkdgjufofpqq")
public suspend fun helmReleaseSettings(argument: suspend HelmReleaseSettingsArgsBuilder.() -> Unit) {
val toBeMapped = HelmReleaseSettingsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.helmReleaseSettings = mapped
}
/**
* @param value Options for tuning the Kubernetes client used by a Provider.
*/
@JvmName("fivhdajugjfugwrr")
public suspend fun kubeClientSettings(`value`: KubeClientSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kubeClientSettings = mapped
}
/**
* @param argument Options for tuning the Kubernetes client used by a Provider.
*/
@JvmName("axynahyrckonaing")
public suspend fun kubeClientSettings(argument: suspend KubeClientSettingsArgsBuilder.() -> Unit) {
val toBeMapped = KubeClientSettingsArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.kubeClientSettings = mapped
}
/**
* @param value The contents of a kubeconfig file or the path to a kubeconfig file.
*/
@JvmName("jdqlgxtellsusbtq")
public suspend fun kubeconfig(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kubeconfig = mapped
}
/**
* @param value If present, the default namespace to use. This flag is ignored for cluster-scoped resources.
* A namespace can be specified in multiple places, and the precedence is as follows:
* 1. `.metadata.namespace` set on the resource.
* 2. This `namespace` parameter.
* 3. `namespace` set for the active context in the kubeconfig.
*/
@JvmName("lvosdrxknorywjnk")
public suspend fun namespace(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.namespace = mapped
}
/**
* @param value BETA FEATURE - If present, render resource manifests to this directory. In this mode, resources will not
* be created on a Kubernetes cluster, but the rendered manifests will be kept in sync with changes
* to the Pulumi program. This feature is in developer preview, and is disabled by default.
* Note that some computed Outputs such as status fields will not be populated
* since the resources are not created on a Kubernetes cluster. These Output values will remain undefined,
* and may result in an error if they are referenced by other resources. Also note that any secret values
* used in these resources will be rendered in plaintext to the resulting YAML.
*/
@JvmName("rtuuvlruxcvldqgr")
public suspend fun renderYamlToDirectory(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.renderYamlToDirectory = mapped
}
/**
* @param value If present and set to true, the provider will skip resources update associated with an unreachable Kubernetes cluster from Pulumi state
*/
@JvmName("annoyjfffhndvbsd")
public suspend fun skipUpdateUnreachable(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.skipUpdateUnreachable = mapped
}
/**
* @param value If present and set to true, suppress apiVersion deprecation warnings from the CLI.
*/
@JvmName("eedhokwjlbynyhdv")
public suspend fun suppressDeprecationWarnings(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.suppressDeprecationWarnings = mapped
}
/**
* @param value If present and set to true, suppress unsupported Helm hook warnings from the CLI.
*/
@JvmName("rygpocnkmuepsjpl")
public suspend fun suppressHelmHookWarnings(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.suppressHelmHookWarnings = mapped
}
internal fun build(): ProviderArgs = ProviderArgs(
cluster = cluster,
clusterIdentifier = clusterIdentifier,
context = context,
deleteUnreachable = deleteUnreachable,
enableConfigMapMutable = enableConfigMapMutable,
enableSecretMutable = enableSecretMutable,
enableServerSideApply = enableServerSideApply,
helmReleaseSettings = helmReleaseSettings,
kubeClientSettings = kubeClientSettings,
kubeconfig = kubeconfig,
namespace = namespace,
renderYamlToDirectory = renderYamlToDirectory,
skipUpdateUnreachable = skipUpdateUnreachable,
suppressDeprecationWarnings = suppressDeprecationWarnings,
suppressHelmHookWarnings = suppressHelmHookWarnings,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy