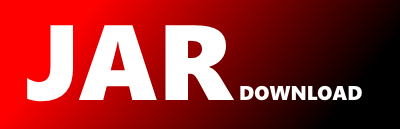
com.pulumi.kubernetes.kustomize.v2.kotlin.DirectoryArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.kustomize.v2.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kubernetes.kustomize.v2.DirectoryArgs.builder
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Directory is a component representing a collection of resources described by a kustomize directory (kustomization).
* ## Example Usage
* ### Local Kustomize Directory
* ```java
* package myproject;
* import com.pulumi.Pulumi;
* import com.pulumi.kubernetes.kustomize.v2.Directory;
* import com.pulumi.kubernetes.kustomize.v2.DirectoryArgs;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(ctx -> {
* var helloWorld = new Directory("helloWorldLocal", DirectoryArgs.builder()
* .directory("./helloWorld")
* .build());
* });
* }
* }
* ```
* ### Kustomize Directory from a Git Repo
* ```java
* package myproject;
* import com.pulumi.Pulumi;
* import com.pulumi.kubernetes.kustomize.v2.Directory;
* import com.pulumi.kubernetes.kustomize.v2.DirectoryArgs;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(ctx -> {
* var helloWorld = new Directory("helloWorldRemote", DirectoryArgs.builder()
* .directory("https://github.com/kubernetes-sigs/kustomize/tree/v3.3.1/examples/helloWorld")
* .build());
* });
* }
* }
* ```
* @property directory The directory containing the kustomization to apply. The value can be a local directory or a folder in a
* git repository.
* Example: ./helloWorld
* Example: https://github.com/kubernetes-sigs/kustomize/tree/master/examples/helloWorld
* @property namespace The default namespace to apply to the resources. Defaults to the provider's namespace.
* @property resourcePrefix A prefix for the auto-generated resource names. Defaults to the name of the Directory resource. Example: A resource created with resourcePrefix="foo" would produce a resource named "foo:resourceName".
* @property skipAwait Indicates that child resources should skip the await logic.
*/
public data class DirectoryArgs(
public val directory: Output? = null,
public val namespace: Output? = null,
public val resourcePrefix: Output? = null,
public val skipAwait: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.kustomize.v2.DirectoryArgs =
com.pulumi.kubernetes.kustomize.v2.DirectoryArgs.builder()
.directory(directory?.applyValue({ args0 -> args0 }))
.namespace(namespace?.applyValue({ args0 -> args0 }))
.resourcePrefix(resourcePrefix?.applyValue({ args0 -> args0 }))
.skipAwait(skipAwait?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DirectoryArgs].
*/
@PulumiTagMarker
public class DirectoryArgsBuilder internal constructor() {
private var directory: Output? = null
private var namespace: Output? = null
private var resourcePrefix: Output? = null
private var skipAwait: Output? = null
/**
* @param value The directory containing the kustomization to apply. The value can be a local directory or a folder in a
* git repository.
* Example: ./helloWorld
* Example: https://github.com/kubernetes-sigs/kustomize/tree/master/examples/helloWorld
*/
@JvmName("phdimdoocsslriiu")
public suspend fun directory(`value`: Output) {
this.directory = value
}
/**
* @param value The default namespace to apply to the resources. Defaults to the provider's namespace.
*/
@JvmName("wwdohyksefbgtdge")
public suspend fun namespace(`value`: Output) {
this.namespace = value
}
/**
* @param value A prefix for the auto-generated resource names. Defaults to the name of the Directory resource. Example: A resource created with resourcePrefix="foo" would produce a resource named "foo:resourceName".
*/
@JvmName("maveickwphgksskb")
public suspend fun resourcePrefix(`value`: Output) {
this.resourcePrefix = value
}
/**
* @param value Indicates that child resources should skip the await logic.
*/
@JvmName("dmcbduuaynpvcgte")
public suspend fun skipAwait(`value`: Output) {
this.skipAwait = value
}
/**
* @param value The directory containing the kustomization to apply. The value can be a local directory or a folder in a
* git repository.
* Example: ./helloWorld
* Example: https://github.com/kubernetes-sigs/kustomize/tree/master/examples/helloWorld
*/
@JvmName("kslquuewfbgahske")
public suspend fun directory(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.directory = mapped
}
/**
* @param value The default namespace to apply to the resources. Defaults to the provider's namespace.
*/
@JvmName("symfhjbrhbstnvfq")
public suspend fun namespace(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.namespace = mapped
}
/**
* @param value A prefix for the auto-generated resource names. Defaults to the name of the Directory resource. Example: A resource created with resourcePrefix="foo" would produce a resource named "foo:resourceName".
*/
@JvmName("ghfqowudiapajyvt")
public suspend fun resourcePrefix(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourcePrefix = mapped
}
/**
* @param value Indicates that child resources should skip the await logic.
*/
@JvmName("hrrykqqrbwrjlmut")
public suspend fun skipAwait(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.skipAwait = mapped
}
internal fun build(): DirectoryArgs = DirectoryArgs(
directory = directory,
namespace = namespace,
resourcePrefix = resourcePrefix,
skipAwait = skipAwait,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy