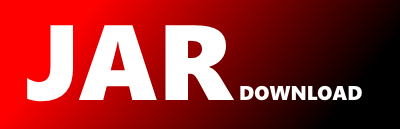
com.pulumi.kubernetes.meta.v1.kotlin.inputs.ManagedFieldsEntryPatchArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.meta.v1.kotlin.inputs
import com.google.gson.JsonParser
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kubernetes.meta.v1.inputs.ManagedFieldsEntryPatchArgs.builder
import kotlinx.serialization.json.Json
import kotlinx.serialization.json.JsonElement
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* ManagedFieldsEntry is a workflow-id, a FieldSet and the group version of the resource that the fieldset applies to.
* @property apiVersion APIVersion defines the version of this resource that this field set applies to. The format is "group/version" just like the top-level APIVersion field. It is necessary to track the version of a field set because it cannot be automatically converted.
* @property fieldsType FieldsType is the discriminator for the different fields format and version. There is currently only one possible value: "FieldsV1"
* @property fieldsV1 FieldsV1 holds the first JSON version format as described in the "FieldsV1" type.
* @property manager Manager is an identifier of the workflow managing these fields.
* @property operation Operation is the type of operation which lead to this ManagedFieldsEntry being created. The only valid values for this field are 'Apply' and 'Update'.
* @property subresource Subresource is the name of the subresource used to update that object, or empty string if the object was updated through the main resource. The value of this field is used to distinguish between managers, even if they share the same name. For example, a status update will be distinct from a regular update using the same manager name. Note that the APIVersion field is not related to the Subresource field and it always corresponds to the version of the main resource.
* @property time Time is the timestamp of when the ManagedFields entry was added. The timestamp will also be updated if a field is added, the manager changes any of the owned fields value or removes a field. The timestamp does not update when a field is removed from the entry because another manager took it over.
*/
public data class ManagedFieldsEntryPatchArgs(
public val apiVersion: Output? = null,
public val fieldsType: Output? = null,
public val fieldsV1: Output? = null,
public val manager: Output? = null,
public val operation: Output? = null,
public val subresource: Output? = null,
public val time: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.meta.v1.inputs.ManagedFieldsEntryPatchArgs =
com.pulumi.kubernetes.meta.v1.inputs.ManagedFieldsEntryPatchArgs.builder()
.apiVersion(apiVersion?.applyValue({ args0 -> args0 }))
.fieldsType(fieldsType?.applyValue({ args0 -> args0 }))
.fieldsV1(
fieldsV1?.applyValue({ args0 ->
JsonParser.parseString(Json.encodeToString(JsonElement.serializer(), args0))
}),
)
.manager(manager?.applyValue({ args0 -> args0 }))
.operation(operation?.applyValue({ args0 -> args0 }))
.subresource(subresource?.applyValue({ args0 -> args0 }))
.time(time?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ManagedFieldsEntryPatchArgs].
*/
@PulumiTagMarker
public class ManagedFieldsEntryPatchArgsBuilder internal constructor() {
private var apiVersion: Output? = null
private var fieldsType: Output? = null
private var fieldsV1: Output? = null
private var manager: Output? = null
private var operation: Output? = null
private var subresource: Output? = null
private var time: Output? = null
/**
* @param value APIVersion defines the version of this resource that this field set applies to. The format is "group/version" just like the top-level APIVersion field. It is necessary to track the version of a field set because it cannot be automatically converted.
*/
@JvmName("bdytoqogbywdynoq")
public suspend fun apiVersion(`value`: Output) {
this.apiVersion = value
}
/**
* @param value FieldsType is the discriminator for the different fields format and version. There is currently only one possible value: "FieldsV1"
*/
@JvmName("hvwvpoxwycqllgnw")
public suspend fun fieldsType(`value`: Output) {
this.fieldsType = value
}
/**
* @param value FieldsV1 holds the first JSON version format as described in the "FieldsV1" type.
*/
@JvmName("fasyvjavinwggeok")
public suspend fun fieldsV1(`value`: Output) {
this.fieldsV1 = value
}
/**
* @param value Manager is an identifier of the workflow managing these fields.
*/
@JvmName("bwcbklvntwbtxycq")
public suspend fun manager(`value`: Output) {
this.manager = value
}
/**
* @param value Operation is the type of operation which lead to this ManagedFieldsEntry being created. The only valid values for this field are 'Apply' and 'Update'.
*/
@JvmName("ofwqwiapefydojdb")
public suspend fun operation(`value`: Output) {
this.operation = value
}
/**
* @param value Subresource is the name of the subresource used to update that object, or empty string if the object was updated through the main resource. The value of this field is used to distinguish between managers, even if they share the same name. For example, a status update will be distinct from a regular update using the same manager name. Note that the APIVersion field is not related to the Subresource field and it always corresponds to the version of the main resource.
*/
@JvmName("fnkflvqkgibnuvio")
public suspend fun subresource(`value`: Output) {
this.subresource = value
}
/**
* @param value Time is the timestamp of when the ManagedFields entry was added. The timestamp will also be updated if a field is added, the manager changes any of the owned fields value or removes a field. The timestamp does not update when a field is removed from the entry because another manager took it over.
*/
@JvmName("urwvsgatdsudclfn")
public suspend fun time(`value`: Output) {
this.time = value
}
/**
* @param value APIVersion defines the version of this resource that this field set applies to. The format is "group/version" just like the top-level APIVersion field. It is necessary to track the version of a field set because it cannot be automatically converted.
*/
@JvmName("lcdianksemmdfxyg")
public suspend fun apiVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiVersion = mapped
}
/**
* @param value FieldsType is the discriminator for the different fields format and version. There is currently only one possible value: "FieldsV1"
*/
@JvmName("cpqisrxiqmntqije")
public suspend fun fieldsType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fieldsType = mapped
}
/**
* @param value FieldsV1 holds the first JSON version format as described in the "FieldsV1" type.
*/
@JvmName("fcdhuvnpwoclyaay")
public suspend fun fieldsV1(`value`: JsonElement?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.fieldsV1 = mapped
}
/**
* @param value Manager is an identifier of the workflow managing these fields.
*/
@JvmName("cxnbngajwiknddoa")
public suspend fun manager(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.manager = mapped
}
/**
* @param value Operation is the type of operation which lead to this ManagedFieldsEntry being created. The only valid values for this field are 'Apply' and 'Update'.
*/
@JvmName("nxgwhmfoxxfbobnu")
public suspend fun operation(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.operation = mapped
}
/**
* @param value Subresource is the name of the subresource used to update that object, or empty string if the object was updated through the main resource. The value of this field is used to distinguish between managers, even if they share the same name. For example, a status update will be distinct from a regular update using the same manager name. Note that the APIVersion field is not related to the Subresource field and it always corresponds to the version of the main resource.
*/
@JvmName("qklfihkodavqatkn")
public suspend fun subresource(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.subresource = mapped
}
/**
* @param value Time is the timestamp of when the ManagedFields entry was added. The timestamp will also be updated if a field is added, the manager changes any of the owned fields value or removes a field. The timestamp does not update when a field is removed from the entry because another manager took it over.
*/
@JvmName("hkxntrhfwyrgjgiq")
public suspend fun time(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.time = mapped
}
internal fun build(): ManagedFieldsEntryPatchArgs = ManagedFieldsEntryPatchArgs(
apiVersion = apiVersion,
fieldsType = fieldsType,
fieldsV1 = fieldsV1,
manager = manager,
operation = operation,
subresource = subresource,
time = time,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy