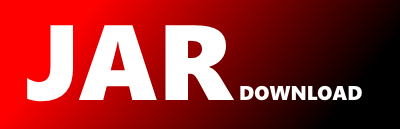
com.pulumi.kubernetes.node.v1beta1.kotlin.RuntimeClassPatch.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.node.v1beta1.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.kubernetes.meta.v1.kotlin.outputs.ObjectMetaPatch
import com.pulumi.kubernetes.node.v1beta1.kotlin.outputs.OverheadPatch
import com.pulumi.kubernetes.node.v1beta1.kotlin.outputs.SchedulingPatch
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.kubernetes.meta.v1.kotlin.outputs.ObjectMetaPatch.Companion.toKotlin as objectMetaPatchToKotlin
import com.pulumi.kubernetes.node.v1beta1.kotlin.outputs.OverheadPatch.Companion.toKotlin as overheadPatchToKotlin
import com.pulumi.kubernetes.node.v1beta1.kotlin.outputs.SchedulingPatch.Companion.toKotlin as schedulingPatchToKotlin
/**
* Builder for [RuntimeClassPatch].
*/
@PulumiTagMarker
public class RuntimeClassPatchResourceBuilder internal constructor() {
public var name: String? = null
public var args: RuntimeClassPatchArgs = RuntimeClassPatchArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend RuntimeClassPatchArgsBuilder.() -> Unit) {
val builder = RuntimeClassPatchArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): RuntimeClassPatch {
val builtJavaResource =
com.pulumi.kubernetes.node.v1beta1.RuntimeClassPatch(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return RuntimeClassPatch(builtJavaResource)
}
}
/**
* Patch resources are used to modify existing Kubernetes resources by using
* Server-Side Apply updates. The name of the resource must be specified, but all other properties are optional. More than
* one patch may be applied to the same resource, and a random FieldManager name will be used for each Patch resource.
* Conflicts will result in an error by default, but can be forced using the "pulumi.com/patchForce" annotation. See the
* [Server-Side Apply Docs](https://www.pulumi.com/registry/packages/kubernetes/how-to-guides/managing-resources-with-server-side-apply/) for
* additional information about using Server-Side Apply to manage Kubernetes resources with Pulumi.
* RuntimeClass defines a class of container runtime supported in the cluster. The RuntimeClass is used to determine which container runtime is used to run all containers in a pod. RuntimeClasses are (currently) manually defined by a user or cluster provisioner, and referenced in the PodSpec. The Kubelet is responsible for resolving the RuntimeClassName reference before running the pod. For more details, see https://git.k8s.io/enhancements/keps/sig-node/runtime-class.md
*/
public class RuntimeClassPatch internal constructor(
override val javaResource: com.pulumi.kubernetes.node.v1beta1.RuntimeClassPatch,
) : KotlinCustomResource(javaResource, RuntimeClassPatchMapper) {
/**
* APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*/
public val apiVersion: Output?
get() = javaResource.apiVersion().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Handler specifies the underlying runtime and configuration that the CRI implementation will use to handle pods of this class. The possible values are specific to the node & CRI configuration. It is assumed that all handlers are available on every node, and handlers of the same name are equivalent on every node. For example, a handler called "runc" might specify that the runc OCI runtime (using native Linux containers) will be used to run the containers in a pod. The Handler must conform to the DNS Label (RFC 1123) requirements, and is immutable.
*/
public val handler: Output?
get() = javaResource.handler().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
public val kind: Output?
get() = javaResource.kind().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#metadata
*/
public val metadata: Output?
get() = javaResource.metadata().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
objectMetaPatchToKotlin(args0)
})
}).orElse(null)
})
/**
* Overhead represents the resource overhead associated with running a pod for a given RuntimeClass. For more details, see https://git.k8s.io/enhancements/keps/sig-node/20190226-pod-overhead.md This field is alpha-level as of Kubernetes v1.15, and is only honored by servers that enable the PodOverhead feature.
*/
public val overhead: Output?
get() = javaResource.overhead().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
overheadPatchToKotlin(args0)
})
}).orElse(null)
})
/**
* Scheduling holds the scheduling constraints to ensure that pods running with this RuntimeClass are scheduled to nodes that support it. If scheduling is nil, this RuntimeClass is assumed to be supported by all nodes.
*/
public val scheduling: Output?
get() = javaResource.scheduling().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
schedulingPatchToKotlin(args0)
})
}).orElse(null)
})
}
public object RuntimeClassPatchMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.kubernetes.node.v1beta1.RuntimeClassPatch::class == javaResource::class
override fun map(javaResource: Resource): RuntimeClassPatch = RuntimeClassPatch(
javaResource as
com.pulumi.kubernetes.node.v1beta1.RuntimeClassPatch,
)
}
/**
* @see [RuntimeClassPatch].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [RuntimeClassPatch].
*/
public suspend fun runtimeClassPatch(
name: String,
block: suspend RuntimeClassPatchResourceBuilder.() -> Unit,
): RuntimeClassPatch {
val builder = RuntimeClassPatchResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [RuntimeClassPatch].
* @param name The _unique_ name of the resulting resource.
*/
public fun runtimeClassPatch(name: String): RuntimeClassPatch {
val builder = RuntimeClassPatchResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy