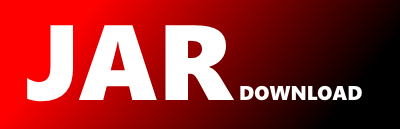
com.pulumi.kubernetes.rbac.v1.kotlin.ClusterRolePatchArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.rbac.v1.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.ObjectMetaPatchArgs
import com.pulumi.kubernetes.meta.v1.kotlin.inputs.ObjectMetaPatchArgsBuilder
import com.pulumi.kubernetes.rbac.v1.ClusterRolePatchArgs.builder
import com.pulumi.kubernetes.rbac.v1.kotlin.inputs.AggregationRulePatchArgs
import com.pulumi.kubernetes.rbac.v1.kotlin.inputs.AggregationRulePatchArgsBuilder
import com.pulumi.kubernetes.rbac.v1.kotlin.inputs.PolicyRulePatchArgs
import com.pulumi.kubernetes.rbac.v1.kotlin.inputs.PolicyRulePatchArgsBuilder
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Patch resources are used to modify existing Kubernetes resources by using
* Server-Side Apply updates. The name of the resource must be specified, but all other properties are optional. More than
* one patch may be applied to the same resource, and a random FieldManager name will be used for each Patch resource.
* Conflicts will result in an error by default, but can be forced using the "pulumi.com/patchForce" annotation. See the
* [Server-Side Apply Docs](https://www.pulumi.com/registry/packages/kubernetes/how-to-guides/managing-resources-with-server-side-apply/) for
* additional information about using Server-Side Apply to manage Kubernetes resources with Pulumi.
* ClusterRole is a cluster level, logical grouping of PolicyRules that can be referenced as a unit by a RoleBinding or ClusterRoleBinding.
* @property aggregationRule AggregationRule is an optional field that describes how to build the Rules for this ClusterRole. If AggregationRule is set, then the Rules are controller managed and direct changes to Rules will be stomped by the controller.
* @property apiVersion APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
* @property kind Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
* @property metadata Standard object's metadata.
* @property rules Rules holds all the PolicyRules for this ClusterRole
*/
public data class ClusterRolePatchArgs(
public val aggregationRule: Output? = null,
public val apiVersion: Output? = null,
public val kind: Output? = null,
public val metadata: Output? = null,
public val rules: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.rbac.v1.ClusterRolePatchArgs =
com.pulumi.kubernetes.rbac.v1.ClusterRolePatchArgs.builder()
.aggregationRule(aggregationRule?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.apiVersion(apiVersion?.applyValue({ args0 -> args0 }))
.kind(kind?.applyValue({ args0 -> args0 }))
.metadata(metadata?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.rules(
rules?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [ClusterRolePatchArgs].
*/
@PulumiTagMarker
public class ClusterRolePatchArgsBuilder internal constructor() {
private var aggregationRule: Output? = null
private var apiVersion: Output? = null
private var kind: Output? = null
private var metadata: Output? = null
private var rules: Output>? = null
/**
* @param value AggregationRule is an optional field that describes how to build the Rules for this ClusterRole. If AggregationRule is set, then the Rules are controller managed and direct changes to Rules will be stomped by the controller.
*/
@JvmName("dtonbkdfvxekkkhm")
public suspend fun aggregationRule(`value`: Output) {
this.aggregationRule = value
}
/**
* @param value APIVersion defines the versioned schema of this representation of an object. Servers should convert recognized schemas to the latest internal value, and may reject unrecognized values. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#resources
*/
@JvmName("gaaddilkdbujiyhe")
public suspend fun apiVersion(`value`: Output) {
this.apiVersion = value
}
/**
* @param value Kind is a string value representing the REST resource this object represents. Servers may infer this from the endpoint the client submits requests to. Cannot be updated. In CamelCase. More info: https://git.k8s.io/community/contributors/devel/sig-architecture/api-conventions.md#types-kinds
*/
@JvmName("feqbmfesaxljpphn")
public suspend fun kind(`value`: Output) {
this.kind = value
}
/**
* @param value Standard object's metadata.
*/
@JvmName("lijejkgkeuphkkfr")
public suspend fun metadata(`value`: Output) {
this.metadata = value
}
/**
* @param value Rules holds all the PolicyRules for this ClusterRole
*/
@JvmName("uwqojavjxccbcmhi")
public suspend fun rules(`value`: Output>) {
this.rules = value
}
@JvmName("vfmkvqwhosrgaepr")
public suspend fun rules(vararg values: Output) {
this.rules = Output.all(values.asList())
}
/**
* @param values Rules holds all the PolicyRules for this ClusterRole
*/
@JvmName("hcwtghpuocxttqiy")
public suspend fun rules(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy