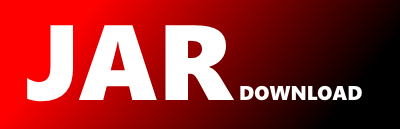
com.pulumi.kubernetes.resource.v1alpha1.kotlin.inputs.AllocationResultArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.resource.v1alpha1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.kubernetes.core.v1.kotlin.inputs.NodeSelectorArgs
import com.pulumi.kubernetes.core.v1.kotlin.inputs.NodeSelectorArgsBuilder
import com.pulumi.kubernetes.resource.v1alpha1.inputs.AllocationResultArgs.builder
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* AllocationResult contains attributed of an allocated resource.
* @property availableOnNodes This field will get set by the resource driver after it has allocated the resource driver to inform the scheduler where it can schedule Pods using the ResourceClaim.
* Setting this field is optional. If null, the resource is available everywhere.
* @property resourceHandle ResourceHandle contains arbitrary data returned by the driver after a successful allocation. This is opaque for Kubernetes. Driver documentation may explain to users how to interpret this data if needed.
* The maximum size of this field is 16KiB. This may get increased in the future, but not reduced.
* @property shareable Shareable determines whether the resource supports more than one consumer at a time.
*/
public data class AllocationResultArgs(
public val availableOnNodes: Output? = null,
public val resourceHandle: Output? = null,
public val shareable: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.resource.v1alpha1.inputs.AllocationResultArgs =
com.pulumi.kubernetes.resource.v1alpha1.inputs.AllocationResultArgs.builder()
.availableOnNodes(availableOnNodes?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.resourceHandle(resourceHandle?.applyValue({ args0 -> args0 }))
.shareable(shareable?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [AllocationResultArgs].
*/
@PulumiTagMarker
public class AllocationResultArgsBuilder internal constructor() {
private var availableOnNodes: Output? = null
private var resourceHandle: Output? = null
private var shareable: Output? = null
/**
* @param value This field will get set by the resource driver after it has allocated the resource driver to inform the scheduler where it can schedule Pods using the ResourceClaim.
* Setting this field is optional. If null, the resource is available everywhere.
*/
@JvmName("yyyimsyhvccfiori")
public suspend fun availableOnNodes(`value`: Output) {
this.availableOnNodes = value
}
/**
* @param value ResourceHandle contains arbitrary data returned by the driver after a successful allocation. This is opaque for Kubernetes. Driver documentation may explain to users how to interpret this data if needed.
* The maximum size of this field is 16KiB. This may get increased in the future, but not reduced.
*/
@JvmName("uynmdlycirlyuftb")
public suspend fun resourceHandle(`value`: Output) {
this.resourceHandle = value
}
/**
* @param value Shareable determines whether the resource supports more than one consumer at a time.
*/
@JvmName("jyoxhjuqxprtfujo")
public suspend fun shareable(`value`: Output) {
this.shareable = value
}
/**
* @param value This field will get set by the resource driver after it has allocated the resource driver to inform the scheduler where it can schedule Pods using the ResourceClaim.
* Setting this field is optional. If null, the resource is available everywhere.
*/
@JvmName("scuhirqoyvcrgwni")
public suspend fun availableOnNodes(`value`: NodeSelectorArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.availableOnNodes = mapped
}
/**
* @param argument This field will get set by the resource driver after it has allocated the resource driver to inform the scheduler where it can schedule Pods using the ResourceClaim.
* Setting this field is optional. If null, the resource is available everywhere.
*/
@JvmName("gvdoyqxbyeubngpl")
public suspend fun availableOnNodes(argument: suspend NodeSelectorArgsBuilder.() -> Unit) {
val toBeMapped = NodeSelectorArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.availableOnNodes = mapped
}
/**
* @param value ResourceHandle contains arbitrary data returned by the driver after a successful allocation. This is opaque for Kubernetes. Driver documentation may explain to users how to interpret this data if needed.
* The maximum size of this field is 16KiB. This may get increased in the future, but not reduced.
*/
@JvmName("lrdbfpsarcwfnugx")
public suspend fun resourceHandle(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceHandle = mapped
}
/**
* @param value Shareable determines whether the resource supports more than one consumer at a time.
*/
@JvmName("tnndeobnarkxnoud")
public suspend fun shareable(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.shareable = mapped
}
internal fun build(): AllocationResultArgs = AllocationResultArgs(
availableOnNodes = availableOnNodes,
resourceHandle = resourceHandle,
shareable = shareable,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy