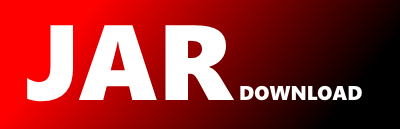
com.pulumi.kubernetes.resource.v1alpha2.kotlin.inputs.NamedResourcesAttributeArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.resource.v1alpha2.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.kubernetes.resource.v1alpha2.inputs.NamedResourcesAttributeArgs.builder
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* NamedResourcesAttribute is a combination of an attribute name and its value.
* @property bool BoolValue is a true/false value.
* @property int IntValue is a 64-bit integer.
* @property intSlice IntSliceValue is an array of 64-bit integers.
* @property name Name is unique identifier among all resource instances managed by the driver on the node. It must be a DNS subdomain.
* @property quantity QuantityValue is a quantity.
* @property string StringValue is a string.
* @property stringSlice StringSliceValue is an array of strings.
* @property version VersionValue is a semantic version according to semver.org spec 2.0.0.
*/
public data class NamedResourcesAttributeArgs(
public val bool: Output? = null,
public val int: Output? = null,
public val intSlice: Output? = null,
public val name: Output,
public val quantity: Output? = null,
public val string: Output? = null,
public val stringSlice: Output? = null,
public val version: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.resource.v1alpha2.inputs.NamedResourcesAttributeArgs = com.pulumi.kubernetes.resource.v1alpha2.inputs.NamedResourcesAttributeArgs.builder()
.bool(bool?.applyValue({ args0 -> args0 }))
.int_(int?.applyValue({ args0 -> args0 }))
.intSlice(intSlice?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.name(name.applyValue({ args0 -> args0 }))
.quantity(quantity?.applyValue({ args0 -> args0 }))
.string(string?.applyValue({ args0 -> args0 }))
.stringSlice(stringSlice?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.version(version?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [NamedResourcesAttributeArgs].
*/
@PulumiTagMarker
public class NamedResourcesAttributeArgsBuilder internal constructor() {
private var bool: Output? = null
private var int: Output? = null
private var intSlice: Output? = null
private var name: Output? = null
private var quantity: Output? = null
private var string: Output? = null
private var stringSlice: Output? = null
private var version: Output? = null
/**
* @param value BoolValue is a true/false value.
*/
@JvmName("doeokyqthcolsryo")
public suspend fun bool(`value`: Output) {
this.bool = value
}
/**
* @param value IntValue is a 64-bit integer.
*/
@JvmName("icikiqpluhrtvcpu")
public suspend fun int(`value`: Output) {
this.int = value
}
/**
* @param value IntSliceValue is an array of 64-bit integers.
*/
@JvmName("jsdlahdtswurpgli")
public suspend fun intSlice(`value`: Output) {
this.intSlice = value
}
/**
* @param value Name is unique identifier among all resource instances managed by the driver on the node. It must be a DNS subdomain.
*/
@JvmName("rkxhlvjpgtqigulm")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value QuantityValue is a quantity.
*/
@JvmName("gyktecdggilatvlf")
public suspend fun quantity(`value`: Output) {
this.quantity = value
}
/**
* @param value StringValue is a string.
*/
@JvmName("agfevhbhixnmwpti")
public suspend fun string(`value`: Output) {
this.string = value
}
/**
* @param value StringSliceValue is an array of strings.
*/
@JvmName("huufluarxnvtmgxa")
public suspend fun stringSlice(`value`: Output) {
this.stringSlice = value
}
/**
* @param value VersionValue is a semantic version according to semver.org spec 2.0.0.
*/
@JvmName("hcuxquhjcraqsnhj")
public suspend fun version(`value`: Output) {
this.version = value
}
/**
* @param value BoolValue is a true/false value.
*/
@JvmName("qpngqxcpgeawesfo")
public suspend fun bool(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.bool = mapped
}
/**
* @param value IntValue is a 64-bit integer.
*/
@JvmName("uxybnfvpuawhejxw")
public suspend fun int(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.int = mapped
}
/**
* @param value IntSliceValue is an array of 64-bit integers.
*/
@JvmName("bttufonmmbnmimri")
public suspend fun intSlice(`value`: NamedResourcesIntSliceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.intSlice = mapped
}
/**
* @param argument IntSliceValue is an array of 64-bit integers.
*/
@JvmName("ikdosagsdpgnwcas")
public suspend fun intSlice(argument: suspend NamedResourcesIntSliceArgsBuilder.() -> Unit) {
val toBeMapped = NamedResourcesIntSliceArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.intSlice = mapped
}
/**
* @param value Name is unique identifier among all resource instances managed by the driver on the node. It must be a DNS subdomain.
*/
@JvmName("bkjmhxqvtxwuhpyq")
public suspend fun name(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value QuantityValue is a quantity.
*/
@JvmName("haiioqeeuagawchc")
public suspend fun quantity(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.quantity = mapped
}
/**
* @param value StringValue is a string.
*/
@JvmName("tihggmgowwmgbhnu")
public suspend fun string(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.string = mapped
}
/**
* @param value StringSliceValue is an array of strings.
*/
@JvmName("fnaeyuifcwunvylg")
public suspend fun stringSlice(`value`: NamedResourcesStringSliceArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.stringSlice = mapped
}
/**
* @param argument StringSliceValue is an array of strings.
*/
@JvmName("mdityshvfqrwccbm")
public suspend fun stringSlice(argument: suspend NamedResourcesStringSliceArgsBuilder.() -> Unit) {
val toBeMapped = NamedResourcesStringSliceArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.stringSlice = mapped
}
/**
* @param value VersionValue is a semantic version according to semver.org spec 2.0.0.
*/
@JvmName("dtvimglhrimhoonn")
public suspend fun version(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.version = mapped
}
internal fun build(): NamedResourcesAttributeArgs = NamedResourcesAttributeArgs(
bool = bool,
int = int,
intSlice = intSlice,
name = name ?: throw PulumiNullFieldException("name"),
quantity = quantity,
string = string,
stringSlice = stringSlice,
version = version,
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy