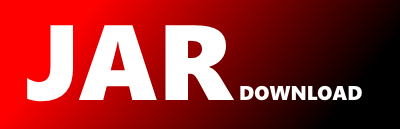
com.pulumi.kubernetes.resource.v1alpha3.kotlin.inputs.DeviceAllocationResultArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.resource.v1alpha3.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.kubernetes.resource.v1alpha3.inputs.DeviceAllocationResultArgs.builder
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* DeviceAllocationResult is the result of allocating devices.
* @property config This field is a combination of all the claim and class configuration parameters. Drivers can distinguish between those based on a flag.
* This includes configuration parameters for drivers which have no allocated devices in the result because it is up to the drivers which configuration parameters they support. They can silently ignore unknown configuration parameters.
* @property results Results lists all allocated devices.
*/
public data class DeviceAllocationResultArgs(
public val config: Output>? = null,
public val results: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.resource.v1alpha3.inputs.DeviceAllocationResultArgs =
com.pulumi.kubernetes.resource.v1alpha3.inputs.DeviceAllocationResultArgs.builder()
.config(config?.applyValue({ args0 -> args0.map({ args0 -> args0.let({ args0 -> args0.toJava() }) }) }))
.results(
results?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [DeviceAllocationResultArgs].
*/
@PulumiTagMarker
public class DeviceAllocationResultArgsBuilder internal constructor() {
private var config: Output>? = null
private var results: Output>? = null
/**
* @param value This field is a combination of all the claim and class configuration parameters. Drivers can distinguish between those based on a flag.
* This includes configuration parameters for drivers which have no allocated devices in the result because it is up to the drivers which configuration parameters they support. They can silently ignore unknown configuration parameters.
*/
@JvmName("tosynfrwkgyqgsno")
public suspend fun config(`value`: Output>) {
this.config = value
}
@JvmName("skpgoshuhtmrnawe")
public suspend fun config(vararg values: Output) {
this.config = Output.all(values.asList())
}
/**
* @param values This field is a combination of all the claim and class configuration parameters. Drivers can distinguish between those based on a flag.
* This includes configuration parameters for drivers which have no allocated devices in the result because it is up to the drivers which configuration parameters they support. They can silently ignore unknown configuration parameters.
*/
@JvmName("hirocikqcmcgqxfa")
public suspend fun config(values: List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy