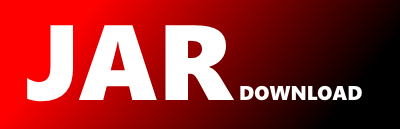
com.pulumi.kubernetes.admissionregistration.v1alpha1.kotlin.inputs.JSONPatchArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.admissionregistration.v1alpha1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kubernetes.admissionregistration.v1alpha1.inputs.JSONPatchArgs.builder
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* JSONPatch defines a JSON Patch.
* @property expression expression will be evaluated by CEL to create a [JSON patch](https://jsonpatch.com/). ref: https://github.com/google/cel-spec
* expression must return an array of JSONPatch values.
* For example, this CEL expression returns a JSON patch to conditionally modify a value:
* [
* JSONPatch{op: "test", path: "/spec/example", value: "Red"},
* JSONPatch{op: "replace", path: "/spec/example", value: "Green"}
* ]
* To define an object for the patch value, use Object types. For example:
* [
* JSONPatch{
* op: "add",
* path: "/spec/selector",
* value: Object.spec.selector{matchLabels: {"environment": "test"}}
* }
* ]
* To use strings containing '/' and '~' as JSONPatch path keys, use "jsonpatch.escapeKey". For example:
* [
* JSONPatch{
* op: "add",
* path: "/metadata/labels/" + jsonpatch.escapeKey("example.com/environment"),
* value: "test"
* },
* ]
* CEL expressions have access to the types needed to create JSON patches and objects:
* - 'JSONPatch' - CEL type of JSON Patch operations. JSONPatch has the fields 'op', 'from', 'path' and 'value'.
* See [JSON patch](https://jsonpatch.com/) for more details. The 'value' field may be set to any of: string,
* integer, array, map or object. If set, the 'path' and 'from' fields must be set to a
* [JSON pointer](https://datatracker.ietf.org/doc/html/rfc6901/) string, where the 'jsonpatch.escapeKey()' CEL
* function may be used to escape path keys containing '/' and '~'.
* - 'Object' - CEL type of the resource object. - 'Object.' - CEL type of object field (such as 'Object.spec') - 'Object.....` - CEL type of nested field (such as 'Object.spec.containers')
* CEL expressions have access to the contents of the API request, organized into CEL variables as well as some other useful variables:
* - 'object' - The object from the incoming request. The value is null for DELETE requests. - 'oldObject' - The existing object. The value is null for CREATE requests. - 'request' - Attributes of the API request([ref](/pkg/apis/admission/types.go#AdmissionRequest)). - 'params' - Parameter resource referred to by the policy binding being evaluated. Only populated if the policy has a ParamKind. - 'namespaceObject' - The namespace object that the incoming object belongs to. The value is null for cluster-scoped resources. - 'variables' - Map of composited variables, from its name to its lazily evaluated value.
* For example, a variable named 'foo' can be accessed as 'variables.foo'.
* - 'authorizer' - A CEL Authorizer. May be used to perform authorization checks for the principal (user or service account) of the request.
* See https://pkg.go.dev/k8s.io/apiserver/pkg/cel/library#Authz
* - 'authorizer.requestResource' - A CEL ResourceCheck constructed from the 'authorizer' and configured with the
* request resource.
* CEL expressions have access to [Kubernetes CEL function libraries](https://kubernetes.io/docs/reference/using-api/cel/#cel-options-language-features-and-libraries) as well as:
* - 'jsonpatch.escapeKey' - Performs JSONPatch key escaping. '~' and '/' are escaped as '~0' and `~1' respectively).
* Only property names of the form `[a-zA-Z_.-/][a-zA-Z0-9_.-/]*` are accessible. Required.
*/
public data class JSONPatchArgs(
public val expression: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.admissionregistration.v1alpha1.inputs.JSONPatchArgs =
com.pulumi.kubernetes.admissionregistration.v1alpha1.inputs.JSONPatchArgs.builder()
.expression(expression?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [JSONPatchArgs].
*/
@PulumiTagMarker
public class JSONPatchArgsBuilder internal constructor() {
private var expression: Output? = null
/**
* @param value expression will be evaluated by CEL to create a [JSON patch](https://jsonpatch.com/). ref: https://github.com/google/cel-spec
* expression must return an array of JSONPatch values.
* For example, this CEL expression returns a JSON patch to conditionally modify a value:
* [
* JSONPatch{op: "test", path: "/spec/example", value: "Red"},
* JSONPatch{op: "replace", path: "/spec/example", value: "Green"}
* ]
* To define an object for the patch value, use Object types. For example:
* [
* JSONPatch{
* op: "add",
* path: "/spec/selector",
* value: Object.spec.selector{matchLabels: {"environment": "test"}}
* }
* ]
* To use strings containing '/' and '~' as JSONPatch path keys, use "jsonpatch.escapeKey". For example:
* [
* JSONPatch{
* op: "add",
* path: "/metadata/labels/" + jsonpatch.escapeKey("example.com/environment"),
* value: "test"
* },
* ]
* CEL expressions have access to the types needed to create JSON patches and objects:
* - 'JSONPatch' - CEL type of JSON Patch operations. JSONPatch has the fields 'op', 'from', 'path' and 'value'.
* See [JSON patch](https://jsonpatch.com/) for more details. The 'value' field may be set to any of: string,
* integer, array, map or object. If set, the 'path' and 'from' fields must be set to a
* [JSON pointer](https://datatracker.ietf.org/doc/html/rfc6901/) string, where the 'jsonpatch.escapeKey()' CEL
* function may be used to escape path keys containing '/' and '~'.
* - 'Object' - CEL type of the resource object. - 'Object.' - CEL type of object field (such as 'Object.spec') - 'Object.....` - CEL type of nested field (such as 'Object.spec.containers')
* CEL expressions have access to the contents of the API request, organized into CEL variables as well as some other useful variables:
* - 'object' - The object from the incoming request. The value is null for DELETE requests. - 'oldObject' - The existing object. The value is null for CREATE requests. - 'request' - Attributes of the API request([ref](/pkg/apis/admission/types.go#AdmissionRequest)). - 'params' - Parameter resource referred to by the policy binding being evaluated. Only populated if the policy has a ParamKind. - 'namespaceObject' - The namespace object that the incoming object belongs to. The value is null for cluster-scoped resources. - 'variables' - Map of composited variables, from its name to its lazily evaluated value.
* For example, a variable named 'foo' can be accessed as 'variables.foo'.
* - 'authorizer' - A CEL Authorizer. May be used to perform authorization checks for the principal (user or service account) of the request.
* See https://pkg.go.dev/k8s.io/apiserver/pkg/cel/library#Authz
* - 'authorizer.requestResource' - A CEL ResourceCheck constructed from the 'authorizer' and configured with the
* request resource.
* CEL expressions have access to [Kubernetes CEL function libraries](https://kubernetes.io/docs/reference/using-api/cel/#cel-options-language-features-and-libraries) as well as:
* - 'jsonpatch.escapeKey' - Performs JSONPatch key escaping. '~' and '/' are escaped as '~0' and `~1' respectively).
* Only property names of the form `[a-zA-Z_.-/][a-zA-Z0-9_.-/]*` are accessible. Required.
*/
@JvmName("oldvsngnkvpmrcjw")
public suspend fun expression(`value`: Output) {
this.expression = value
}
/**
* @param value expression will be evaluated by CEL to create a [JSON patch](https://jsonpatch.com/). ref: https://github.com/google/cel-spec
* expression must return an array of JSONPatch values.
* For example, this CEL expression returns a JSON patch to conditionally modify a value:
* [
* JSONPatch{op: "test", path: "/spec/example", value: "Red"},
* JSONPatch{op: "replace", path: "/spec/example", value: "Green"}
* ]
* To define an object for the patch value, use Object types. For example:
* [
* JSONPatch{
* op: "add",
* path: "/spec/selector",
* value: Object.spec.selector{matchLabels: {"environment": "test"}}
* }
* ]
* To use strings containing '/' and '~' as JSONPatch path keys, use "jsonpatch.escapeKey". For example:
* [
* JSONPatch{
* op: "add",
* path: "/metadata/labels/" + jsonpatch.escapeKey("example.com/environment"),
* value: "test"
* },
* ]
* CEL expressions have access to the types needed to create JSON patches and objects:
* - 'JSONPatch' - CEL type of JSON Patch operations. JSONPatch has the fields 'op', 'from', 'path' and 'value'.
* See [JSON patch](https://jsonpatch.com/) for more details. The 'value' field may be set to any of: string,
* integer, array, map or object. If set, the 'path' and 'from' fields must be set to a
* [JSON pointer](https://datatracker.ietf.org/doc/html/rfc6901/) string, where the 'jsonpatch.escapeKey()' CEL
* function may be used to escape path keys containing '/' and '~'.
* - 'Object' - CEL type of the resource object. - 'Object.' - CEL type of object field (such as 'Object.spec') - 'Object.....` - CEL type of nested field (such as 'Object.spec.containers')
* CEL expressions have access to the contents of the API request, organized into CEL variables as well as some other useful variables:
* - 'object' - The object from the incoming request. The value is null for DELETE requests. - 'oldObject' - The existing object. The value is null for CREATE requests. - 'request' - Attributes of the API request([ref](/pkg/apis/admission/types.go#AdmissionRequest)). - 'params' - Parameter resource referred to by the policy binding being evaluated. Only populated if the policy has a ParamKind. - 'namespaceObject' - The namespace object that the incoming object belongs to. The value is null for cluster-scoped resources. - 'variables' - Map of composited variables, from its name to its lazily evaluated value.
* For example, a variable named 'foo' can be accessed as 'variables.foo'.
* - 'authorizer' - A CEL Authorizer. May be used to perform authorization checks for the principal (user or service account) of the request.
* See https://pkg.go.dev/k8s.io/apiserver/pkg/cel/library#Authz
* - 'authorizer.requestResource' - A CEL ResourceCheck constructed from the 'authorizer' and configured with the
* request resource.
* CEL expressions have access to [Kubernetes CEL function libraries](https://kubernetes.io/docs/reference/using-api/cel/#cel-options-language-features-and-libraries) as well as:
* - 'jsonpatch.escapeKey' - Performs JSONPatch key escaping. '~' and '/' are escaped as '~0' and `~1' respectively).
* Only property names of the form `[a-zA-Z_.-/][a-zA-Z0-9_.-/]*` are accessible. Required.
*/
@JvmName("ioiwdebgjyfrglyd")
public suspend fun expression(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.expression = mapped
}
internal fun build(): JSONPatchArgs = JSONPatchArgs(
expression = expression,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy