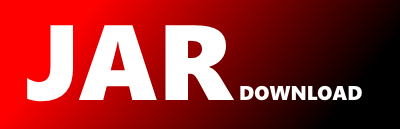
com.pulumi.kubernetes.admissionregistration.v1alpha1.kotlin.inputs.MutatingAdmissionPolicyBindingSpecPatchArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-kubernetes-kotlin Show documentation
Show all versions of pulumi-kubernetes-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.kubernetes.admissionregistration.v1alpha1.kotlin.inputs
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import com.pulumi.kubernetes.admissionregistration.v1alpha1.inputs.MutatingAdmissionPolicyBindingSpecPatchArgs.builder
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* MutatingAdmissionPolicyBindingSpec is the specification of the MutatingAdmissionPolicyBinding.
* @property matchResources matchResources limits what resources match this binding and may be mutated by it. Note that if matchResources matches a resource, the resource must also match a policy's matchConstraints and matchConditions before the resource may be mutated. When matchResources is unset, it does not constrain resource matching, and only the policy's matchConstraints and matchConditions must match for the resource to be mutated. Additionally, matchResources.resourceRules are optional and do not constraint matching when unset. Note that this is differs from MutatingAdmissionPolicy matchConstraints, where resourceRules are required. The CREATE, UPDATE and CONNECT operations are allowed. The DELETE operation may not be matched. '*' matches CREATE, UPDATE and CONNECT.
* @property paramRef paramRef specifies the parameter resource used to configure the admission control policy. It should point to a resource of the type specified in spec.ParamKind of the bound MutatingAdmissionPolicy. If the policy specifies a ParamKind and the resource referred to by ParamRef does not exist, this binding is considered mis-configured and the FailurePolicy of the MutatingAdmissionPolicy applied. If the policy does not specify a ParamKind then this field is ignored, and the rules are evaluated without a param.
* @property policyName policyName references a MutatingAdmissionPolicy name which the MutatingAdmissionPolicyBinding binds to. If the referenced resource does not exist, this binding is considered invalid and will be ignored Required.
*/
public data class MutatingAdmissionPolicyBindingSpecPatchArgs(
public val matchResources: Output? = null,
public val paramRef: Output? = null,
public val policyName: Output? = null,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.kubernetes.admissionregistration.v1alpha1.inputs.MutatingAdmissionPolicyBindingSpecPatchArgs =
com.pulumi.kubernetes.admissionregistration.v1alpha1.inputs.MutatingAdmissionPolicyBindingSpecPatchArgs.builder()
.matchResources(matchResources?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.paramRef(paramRef?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.policyName(policyName?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [MutatingAdmissionPolicyBindingSpecPatchArgs].
*/
@PulumiTagMarker
public class MutatingAdmissionPolicyBindingSpecPatchArgsBuilder internal constructor() {
private var matchResources: Output? = null
private var paramRef: Output? = null
private var policyName: Output? = null
/**
* @param value matchResources limits what resources match this binding and may be mutated by it. Note that if matchResources matches a resource, the resource must also match a policy's matchConstraints and matchConditions before the resource may be mutated. When matchResources is unset, it does not constrain resource matching, and only the policy's matchConstraints and matchConditions must match for the resource to be mutated. Additionally, matchResources.resourceRules are optional and do not constraint matching when unset. Note that this is differs from MutatingAdmissionPolicy matchConstraints, where resourceRules are required. The CREATE, UPDATE and CONNECT operations are allowed. The DELETE operation may not be matched. '*' matches CREATE, UPDATE and CONNECT.
*/
@JvmName("kswqfpbchnjybvcj")
public suspend fun matchResources(`value`: Output) {
this.matchResources = value
}
/**
* @param value paramRef specifies the parameter resource used to configure the admission control policy. It should point to a resource of the type specified in spec.ParamKind of the bound MutatingAdmissionPolicy. If the policy specifies a ParamKind and the resource referred to by ParamRef does not exist, this binding is considered mis-configured and the FailurePolicy of the MutatingAdmissionPolicy applied. If the policy does not specify a ParamKind then this field is ignored, and the rules are evaluated without a param.
*/
@JvmName("ridixmufbcaiuyhi")
public suspend fun paramRef(`value`: Output) {
this.paramRef = value
}
/**
* @param value policyName references a MutatingAdmissionPolicy name which the MutatingAdmissionPolicyBinding binds to. If the referenced resource does not exist, this binding is considered invalid and will be ignored Required.
*/
@JvmName("vqphbfayuquykeyv")
public suspend fun policyName(`value`: Output) {
this.policyName = value
}
/**
* @param value matchResources limits what resources match this binding and may be mutated by it. Note that if matchResources matches a resource, the resource must also match a policy's matchConstraints and matchConditions before the resource may be mutated. When matchResources is unset, it does not constrain resource matching, and only the policy's matchConstraints and matchConditions must match for the resource to be mutated. Additionally, matchResources.resourceRules are optional and do not constraint matching when unset. Note that this is differs from MutatingAdmissionPolicy matchConstraints, where resourceRules are required. The CREATE, UPDATE and CONNECT operations are allowed. The DELETE operation may not be matched. '*' matches CREATE, UPDATE and CONNECT.
*/
@JvmName("hnkwxounlcicahjw")
public suspend fun matchResources(`value`: MatchResourcesPatchArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.matchResources = mapped
}
/**
* @param argument matchResources limits what resources match this binding and may be mutated by it. Note that if matchResources matches a resource, the resource must also match a policy's matchConstraints and matchConditions before the resource may be mutated. When matchResources is unset, it does not constrain resource matching, and only the policy's matchConstraints and matchConditions must match for the resource to be mutated. Additionally, matchResources.resourceRules are optional and do not constraint matching when unset. Note that this is differs from MutatingAdmissionPolicy matchConstraints, where resourceRules are required. The CREATE, UPDATE and CONNECT operations are allowed. The DELETE operation may not be matched. '*' matches CREATE, UPDATE and CONNECT.
*/
@JvmName("kmxclfnfeerxnnpb")
public suspend fun matchResources(argument: suspend MatchResourcesPatchArgsBuilder.() -> Unit) {
val toBeMapped = MatchResourcesPatchArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.matchResources = mapped
}
/**
* @param value paramRef specifies the parameter resource used to configure the admission control policy. It should point to a resource of the type specified in spec.ParamKind of the bound MutatingAdmissionPolicy. If the policy specifies a ParamKind and the resource referred to by ParamRef does not exist, this binding is considered mis-configured and the FailurePolicy of the MutatingAdmissionPolicy applied. If the policy does not specify a ParamKind then this field is ignored, and the rules are evaluated without a param.
*/
@JvmName("gnhaysbohpflglnt")
public suspend fun paramRef(`value`: ParamRefPatchArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.paramRef = mapped
}
/**
* @param argument paramRef specifies the parameter resource used to configure the admission control policy. It should point to a resource of the type specified in spec.ParamKind of the bound MutatingAdmissionPolicy. If the policy specifies a ParamKind and the resource referred to by ParamRef does not exist, this binding is considered mis-configured and the FailurePolicy of the MutatingAdmissionPolicy applied. If the policy does not specify a ParamKind then this field is ignored, and the rules are evaluated without a param.
*/
@JvmName("sxneserfotgbpqey")
public suspend fun paramRef(argument: suspend ParamRefPatchArgsBuilder.() -> Unit) {
val toBeMapped = ParamRefPatchArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.paramRef = mapped
}
/**
* @param value policyName references a MutatingAdmissionPolicy name which the MutatingAdmissionPolicyBinding binds to. If the referenced resource does not exist, this binding is considered invalid and will be ignored Required.
*/
@JvmName("nammetpxhmxkabaj")
public suspend fun policyName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.policyName = mapped
}
internal fun build(): MutatingAdmissionPolicyBindingSpecPatchArgs =
MutatingAdmissionPolicyBindingSpecPatchArgs(
matchResources = matchResources,
paramRef = paramRef,
policyName = policyName,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy