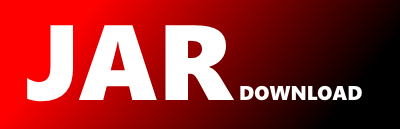
com.pulumi.nomad.kotlin.AclAuthMethod.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-nomad-kotlin Show documentation
Show all versions of pulumi-nomad-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.nomad.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.nomad.kotlin.outputs.AclAuthMethodConfig
import com.pulumi.nomad.kotlin.outputs.AclAuthMethodConfig.Companion.toKotlin
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [AclAuthMethod].
*/
@PulumiTagMarker
public class AclAuthMethodResourceBuilder internal constructor() {
public var name: String? = null
public var args: AclAuthMethodArgs = AclAuthMethodArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend AclAuthMethodArgsBuilder.() -> Unit) {
val builder = AclAuthMethodArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): AclAuthMethod {
val builtJavaResource = com.pulumi.nomad.AclAuthMethod(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return AclAuthMethod(builtJavaResource)
}
}
/**
* Manages an ACL Auth Method in Nomad.
* ## Example Usage
* Creating an ALC Auth Method:
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as nomad from "@pulumi/nomad";
* const myNomadAclAuthMethod = new nomad.AclAuthMethod("my_nomad_acl_auth_method", {
* name: "my-nomad-acl-auth-method",
* type: "OIDC",
* tokenLocality: "global",
* maxTokenTtl: "10m0s",
* tokenNameFormat: `${auth_method_type}-${value.user}`,
* "default": true,
* config: {
* oidcDiscoveryUrl: "https://uk.auth0.com/",
* oidcClientId: "someclientid",
* oidcClientSecret: "someclientsecret-t",
* boundAudiences: ["someclientid"],
* allowedRedirectUris: [
* "http://localhost:4649/oidc/callback",
* "http://localhost:4646/ui/settings/tokens",
* ],
* listClaimMappings: {
* "http://nomad.internal/roles": "roles",
* },
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_nomad as nomad
* my_nomad_acl_auth_method = nomad.AclAuthMethod("my_nomad_acl_auth_method",
* name="my-nomad-acl-auth-method",
* type="OIDC",
* token_locality="global",
* max_token_ttl="10m0s",
* token_name_format="${auth_method_type}-${value.user}",
* default=True,
* config={
* "oidc_discovery_url": "https://uk.auth0.com/",
* "oidc_client_id": "someclientid",
* "oidc_client_secret": "someclientsecret-t",
* "bound_audiences": ["someclientid"],
* "allowed_redirect_uris": [
* "http://localhost:4649/oidc/callback",
* "http://localhost:4646/ui/settings/tokens",
* ],
* "list_claim_mappings": {
* "http___nomad_internal_roles": "roles",
* },
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Nomad = Pulumi.Nomad;
* return await Deployment.RunAsync(() =>
* {
* var myNomadAclAuthMethod = new Nomad.AclAuthMethod("my_nomad_acl_auth_method", new()
* {
* Name = "my-nomad-acl-auth-method",
* Type = "OIDC",
* TokenLocality = "global",
* MaxTokenTtl = "10m0s",
* TokenNameFormat = "${auth_method_type}-${value.user}",
* Default = true,
* Config = new Nomad.Inputs.AclAuthMethodConfigArgs
* {
* OidcDiscoveryUrl = "https://uk.auth0.com/",
* OidcClientId = "someclientid",
* OidcClientSecret = "someclientsecret-t",
* BoundAudiences = new[]
* {
* "someclientid",
* },
* AllowedRedirectUris = new[]
* {
* "http://localhost:4649/oidc/callback",
* "http://localhost:4646/ui/settings/tokens",
* },
* ListClaimMappings =
* {
* { "http://nomad.internal/roles", "roles" },
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "fmt"
* "github.com/pulumi/pulumi-nomad/sdk/v2/go/nomad"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := nomad.NewAclAuthMethod(ctx, "my_nomad_acl_auth_method", &nomad.AclAuthMethodArgs{
* Name: pulumi.String("my-nomad-acl-auth-method"),
* Type: pulumi.String("OIDC"),
* TokenLocality: pulumi.String("global"),
* MaxTokenTtl: pulumi.String("10m0s"),
* TokenNameFormat: pulumi.Sprintf("${auth_method_type}-${value.user}"),
* Default: pulumi.Bool(true),
* Config: &nomad.AclAuthMethodConfigArgs{
* OidcDiscoveryUrl: pulumi.String("https://uk.auth0.com/"),
* OidcClientId: pulumi.String("someclientid"),
* OidcClientSecret: pulumi.String("someclientsecret-t"),
* BoundAudiences: pulumi.StringArray{
* pulumi.String("someclientid"),
* },
* AllowedRedirectUris: pulumi.StringArray{
* pulumi.String("http://localhost:4649/oidc/callback"),
* pulumi.String("http://localhost:4646/ui/settings/tokens"),
* },
* ListClaimMappings: pulumi.StringMap{
* "http://nomad.internal/roles": pulumi.String("roles"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.nomad.AclAuthMethod;
* import com.pulumi.nomad.AclAuthMethodArgs;
* import com.pulumi.nomad.inputs.AclAuthMethodConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var myNomadAclAuthMethod = new AclAuthMethod("myNomadAclAuthMethod", AclAuthMethodArgs.builder()
* .name("my-nomad-acl-auth-method")
* .type("OIDC")
* .tokenLocality("global")
* .maxTokenTtl("10m0s")
* .tokenNameFormat("${auth_method_type}-${value.user}")
* .default_(true)
* .config(AclAuthMethodConfigArgs.builder()
* .oidcDiscoveryUrl("https://uk.auth0.com/")
* .oidcClientId("someclientid")
* .oidcClientSecret("someclientsecret-t")
* .boundAudiences("someclientid")
* .allowedRedirectUris(
* "http://localhost:4649/oidc/callback",
* "http://localhost:4646/ui/settings/tokens")
* .listClaimMappings(Map.of("http://nomad.internal/roles", "roles"))
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* myNomadAclAuthMethod:
* type: nomad:AclAuthMethod
* name: my_nomad_acl_auth_method
* properties:
* name: my-nomad-acl-auth-method
* type: OIDC
* tokenLocality: global
* maxTokenTtl: 10m0s
* tokenNameFormat: ${auth_method_type}-${value.user}
* default: true
* config:
* oidcDiscoveryUrl: https://uk.auth0.com/
* oidcClientId: someclientid
* oidcClientSecret: someclientsecret-t
* boundAudiences:
* - someclientid
* allowedRedirectUris:
* - http://localhost:4649/oidc/callback
* - http://localhost:4646/ui/settings/tokens
* listClaimMappings:
* http://nomad.internal/roles: roles
* ```
*
*/
public class AclAuthMethod internal constructor(
override val javaResource: com.pulumi.nomad.AclAuthMethod,
) : KotlinCustomResource(javaResource, AclAuthMethodMapper) {
/**
* `(block: )` - Configuration specific to the auth method
* provider.
*/
public val config: Output
get() = javaResource.config().applyValue({ args0 -> args0.let({ args0 -> toKotlin(args0) }) })
/**
* `(bool: false)` - Defines whether this ACL Auth Method is to be set
* as default.
*/
public val default: Output?
get() = javaResource.default_().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* `(string: )` - Defines the maximum life of a token
* created by this method and is specified as a time duration such as "15h".
*/
public val maxTokenTtl: Output
get() = javaResource.maxTokenTtl().applyValue({ args0 -> args0 })
/**
* `(string: )` - The identifier of the ACL Auth Method.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* `(string: )` - Defines whether the ACL Auth Method
* creates a local or global token when performing SSO login. This field must be
* set to either `local` or `global`.
*/
public val tokenLocality: Output
get() = javaResource.tokenLocality().applyValue({ args0 -> args0 })
/**
* `(string: "${auth_method_type}-${auth_method_name}")` -
* Defines the token name format for the generated tokens This can be lightly
* templated using HIL '${foo}' syntax.
*/
public val tokenNameFormat: Output?
get() = javaResource.tokenNameFormat().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* `(string: )` - ACL Auth Method SSO workflow type. Valid values,
* are `OIDC` and `JWT`.
*/
public val type: Output
get() = javaResource.type().applyValue({ args0 -> args0 })
}
public object AclAuthMethodMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.nomad.AclAuthMethod::class == javaResource::class
override fun map(javaResource: Resource): AclAuthMethod = AclAuthMethod(
javaResource as
com.pulumi.nomad.AclAuthMethod,
)
}
/**
* @see [AclAuthMethod].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [AclAuthMethod].
*/
public suspend fun aclAuthMethod(
name: String,
block: suspend AclAuthMethodResourceBuilder.() -> Unit,
): AclAuthMethod {
val builder = AclAuthMethodResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [AclAuthMethod].
* @param name The _unique_ name of the resulting resource.
*/
public fun aclAuthMethod(name: String): AclAuthMethod {
val builder = AclAuthMethodResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy