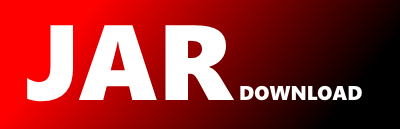
com.pulumi.nomad.kotlin.Job.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.nomad.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.nomad.kotlin.outputs.JobHcl2
import com.pulumi.nomad.kotlin.outputs.JobTaskGroup
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.nomad.kotlin.outputs.JobHcl2.Companion.toKotlin as jobHcl2ToKotlin
import com.pulumi.nomad.kotlin.outputs.JobTaskGroup.Companion.toKotlin as jobTaskGroupToKotlin
/**
* Builder for [Job].
*/
@PulumiTagMarker
public class JobResourceBuilder internal constructor() {
public var name: String? = null
public var args: JobArgs = JobArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend JobArgsBuilder.() -> Unit) {
val builder = JobArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Job {
val builtJavaResource = com.pulumi.nomad.Job(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Job(builtJavaResource)
}
}
public class Job internal constructor(
override val javaResource: com.pulumi.nomad.Job,
) : KotlinCustomResource(javaResource, JobMapper) {
/**
* The IDs for allocations associated with this job.
*/
@Deprecated(
message = """
Retrieving allocation IDs from the job resource is deprecated and will be removed in a future
release. Use the nomad.getAllocations data source instead.
""",
)
public val allocationIds: Output>
get() = javaResource.allocationIds().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* `(string: )` - Consul token used when registering this job.
* Will fallback to the value declared in Nomad provider configuration, if any.
*/
public val consulToken: Output?
get() = javaResource.consulToken().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The target datacenters for the job, as derived from the jobspec.
*/
public val datacenters: Output>
get() = javaResource.datacenters().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* If detach = false, the ID for the deployment associated with the last job create/update, if one exists.
*/
public val deploymentId: Output
get() = javaResource.deploymentId().applyValue({ args0 -> args0 })
/**
* If detach = false, the status for the deployment associated with the last job create/update, if one exists.
*/
public val deploymentStatus: Output
get() = javaResource.deploymentStatus().applyValue({ args0 -> args0 })
/**
* If true, the job will be deregistered on destroy.
*/
public val deregisterOnDestroy: Output?
get() = javaResource.deregisterOnDestroy().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* `(boolean: true)` - Determines if the job will be
* deregistered if the ID of the job in the jobspec changes.
*/
public val deregisterOnIdChange: Output?
get() = javaResource.deregisterOnIdChange().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* `(boolean: true)` - If true, the provider will return immediately
* after creating or updating, instead of monitoring.
*/
public val detach: Output?
get() = javaResource.detach().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* `(boolean: false)` - Set this to `true` to use the previous HCL1
* parser. This option is provided for backwards compatibility only and should
* not be used unless absolutely necessary.
*/
public val hcl1: Output?
get() = javaResource.hcl1().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* `(block: optional)` - Options for the HCL2 jobspec parser.
*/
public val hcl2: Output?
get() = javaResource.hcl2().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
jobHcl2ToKotlin(args0)
})
}).orElse(null)
})
/**
* `(string: )` - The contents of the jobspec to register.
*/
public val jobspec: Output
get() = javaResource.jobspec().applyValue({ args0 -> args0 })
/**
* `(boolean: false)` - Set this to `true` if your jobspec is structured with
* JSON instead of the default HCL.
*/
public val json: Output?
get() = javaResource.json().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Integer that increments for each change. Used to detect any changes between plan and apply.
*/
public val modifyIndex: Output
get() = javaResource.modifyIndex().applyValue({ args0 -> args0 })
/**
* The name of the job, as derived from the jobspec.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The namespace of the job, as derived from the jobspec.
*/
public val namespace: Output
get() = javaResource.namespace().applyValue({ args0 -> args0 })
/**
* `(boolean: false)` - Determines if the job will override any
* soft-mandatory Sentinel policies and register even if they fail.
*/
public val policyOverride: Output?
get() = javaResource.policyOverride().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* `(boolean: false)` - Set this to true if you want the job to
* be purged when the resource is destroyed.
*/
public val purgeOnDestroy: Output?
get() = javaResource.purgeOnDestroy().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
@Deprecated(
message = """
Retrieving allocation IDs from the job resource is deprecated and will be removed in a future
release. Use the nomad.getAllocations data source instead.
""",
)
public val readAllocationIds: Output?
get() = javaResource.readAllocationIds().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The target region for the job, as derived from the jobspec.
*/
public val region: Output
get() = javaResource.region().applyValue({ args0 -> args0 })
/**
* `(boolean: false)` - Set this to true to force the job to run
* again if its status is `dead`.
*/
public val rerunIfDead: Output?
get() = javaResource.rerunIfDead().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The status of the job.
*/
public val status: Output
get() = javaResource.status().applyValue({ args0 -> args0 })
public val taskGroups: Output>
get() = javaResource.taskGroups().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
jobTaskGroupToKotlin(args0)
})
})
})
/**
* The type of the job, as derived from the jobspec.
*/
public val type: Output
get() = javaResource.type().applyValue({ args0 -> args0 })
/**
* `(string: )` - Vault token used when registering this job.
* Will fallback to the value declared in Nomad provider configuration, if any.
*/
public val vaultToken: Output?
get() = javaResource.vaultToken().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
}
public object JobMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.nomad.Job::class == javaResource::class
override fun map(javaResource: Resource): Job = Job(javaResource as com.pulumi.nomad.Job)
}
/**
* @see [Job].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Job].
*/
public suspend fun job(name: String, block: suspend JobResourceBuilder.() -> Unit): Job {
val builder = JobResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Job].
* @param name The _unique_ name of the resulting resource.
*/
public fun job(name: String): Job {
val builder = JobResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy