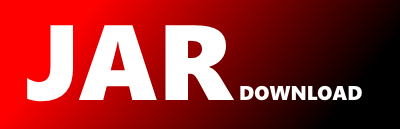
com.pulumi.nomad.kotlin.outputs.GetJobResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-nomad-kotlin Show documentation
Show all versions of pulumi-nomad-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.nomad.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
* A collection of values returned by getJob.
* @property allAtOnce `(boolean)` If the scheduler can make partial placements on oversubscribed nodes.
* @property constraints
* @property createIndex `(integer)` Creation Index.
* @property datacenters `(list of strings)` Datacenters allowed to run the specified job.
* @property id The provider-assigned unique ID for this managed resource.
* @property jobId
* @property jobModifyIndex `(integer)` Job modify index (used for version verification).
* @property modifyIndex `(integer)` Modification Index.
* @property name `(string)` Name of the job.
* @property namespace
* @property parentId `(string)` Job's parent ID.
* @property periodicConfigs `(list of maps)` Job's periodic configuration (time based scheduling).
* @property priority `(integer)` Used for the prioritization of scheduling and resource access.
* @property region `(string)` Region where the Nomad cluster resides.
* @property stable `(boolean)` Job stability status.
* @property status `(string)` Execution status of the specified job.
* @property statusDescription `(string)` Status description of the specified job.
* @property stop `(boolean)` Job enabled status.
* @property submitTime `(integer)` Job submission date.
* @property taskGroups `(list of maps)` A list of of the job's task groups.
* @property type `(string)` Scheduler type used during job creation.
* @property version `(integer)` Version of the specified job.
*/
public data class GetJobResult(
public val allAtOnce: Boolean,
public val constraints: List,
public val createIndex: Int,
public val datacenters: List,
public val id: String,
public val jobId: String,
public val jobModifyIndex: Int,
public val modifyIndex: Int,
public val name: String,
public val namespace: String? = null,
public val parentId: String,
public val periodicConfigs: List,
public val priority: Int,
public val region: String,
public val stable: Boolean,
public val status: String,
public val statusDescription: String,
public val stop: Boolean,
public val submitTime: Int,
public val taskGroups: List,
public val type: String,
public val version: Int,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.nomad.outputs.GetJobResult): GetJobResult =
GetJobResult(
allAtOnce = javaType.allAtOnce(),
constraints = javaType.constraints().map({ args0 ->
args0.let({ args0 ->
com.pulumi.nomad.kotlin.outputs.GetJobConstraint.Companion.toKotlin(args0)
})
}),
createIndex = javaType.createIndex(),
datacenters = javaType.datacenters().map({ args0 -> args0 }),
id = javaType.id(),
jobId = javaType.jobId(),
jobModifyIndex = javaType.jobModifyIndex(),
modifyIndex = javaType.modifyIndex(),
name = javaType.name(),
namespace = javaType.namespace().map({ args0 -> args0 }).orElse(null),
parentId = javaType.parentId(),
periodicConfigs = javaType.periodicConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.nomad.kotlin.outputs.GetJobPeriodicConfig.Companion.toKotlin(args0)
})
}),
priority = javaType.priority(),
region = javaType.region(),
stable = javaType.stable(),
status = javaType.status(),
statusDescription = javaType.statusDescription(),
stop = javaType.stop(),
submitTime = javaType.submitTime(),
taskGroups = javaType.taskGroups().map({ args0 ->
args0.let({ args0 ->
com.pulumi.nomad.kotlin.outputs.GetJobTaskGroup.Companion.toKotlin(args0)
})
}),
type = javaType.type(),
version = javaType.version(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy