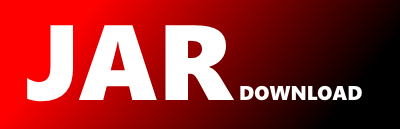
com.pulumi.random.kotlin.RandomId.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.random.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
/**
* Builder for [RandomId].
*/
@PulumiTagMarker
public class RandomIdResourceBuilder internal constructor() {
public var name: String? = null
public var args: RandomIdArgs = RandomIdArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend RandomIdArgsBuilder.() -> Unit) {
val builder = RandomIdArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.opts(block)
}
internal fun build(): RandomId {
val builtJavaResource = com.pulumi.random.RandomId(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return RandomId(builtJavaResource)
}
}
/**
* The resource `random.RandomId` generates random numbers that are intended to be
* used as unique identifiers for other resources. If the output is considered
* sensitive, and should not be displayed in the CLI, use `random.RandomBytes`
* instead.
* This resource *does* use a cryptographic random number generator in order
* to minimize the chance of collisions, making the results of this resource
* when a 16-byte identifier is requested of equivalent uniqueness to a
* type-4 UUID.
* This resource can be used in conjunction with resources that have
* the `create_before_destroy` lifecycle flag set to avoid conflicts with
* unique names during the brief period where both the old and new resources
* exist concurrently.
* ## Example Usage
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.random.RandomId;
* import com.pulumi.random.RandomIdArgs;
* import com.pulumi.aws.ec2.Instance;
* import com.pulumi.aws.ec2.InstanceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var serverRandomId = new RandomId("serverRandomId", RandomIdArgs.builder()
* .keepers(Map.of("ami_id", var_.ami_id()))
* .byteLength(8)
* .build());
* var serverInstance = new Instance("serverInstance", InstanceArgs.builder()
* .tags(Map.of("Name", serverRandomId.hex().applyValue(hex -> String.format("web-server %s", hex))))
* .ami(serverRandomId.keepers().applyValue(keepers -> keepers.amiId()))
* .build());
* }
* }
* ```
* ## Import
* Random IDs can be imported using the b64_url with an optional prefix. This can be used to replace a config value with a value interpolated from the random provider without experiencing diffs. Example with no prefix
* ```sh
* $ pulumi import random:index/randomId:RandomId server p-9hUg
* ```
* Example with prefix (prefix is separated by a ,)
* ```sh
* $ pulumi import random:index/randomId:RandomId server my-prefix-,p-9hUg
* ```
*
*/
public class RandomId internal constructor(
override val javaResource: com.pulumi.random.RandomId,
) : KotlinCustomResource(javaResource, RandomIdMapper) {
/**
* The generated id presented in base64 without additional transformations.
*/
public val b64Std: Output
get() = javaResource.b64Std().applyValue({ args0 -> args0 })
/**
* The generated id presented in base64, using the URL-friendly character set: case-sensitive letters, digits and the characters `_` and `-`.
*/
public val b64Url: Output
get() = javaResource.b64Url().applyValue({ args0 -> args0 })
/**
* The number of random bytes to produce. The minimum value is 1, which produces eight bits of randomness.
*/
public val byteLength: Output
get() = javaResource.byteLength().applyValue({ args0 -> args0 })
/**
* The generated id presented in non-padded decimal digits.
*/
public val dec: Output
get() = javaResource.dec().applyValue({ args0 -> args0 })
/**
* The generated id presented in padded hexadecimal digits. This result will always be twice as long as the requested byte length.
*/
public val hex: Output
get() = javaResource.hex().applyValue({ args0 -> args0 })
/**
* Arbitrary map of values that, when changed, will trigger recreation of resource. See the main provider documentation for more information.
*/
public val keepers: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy