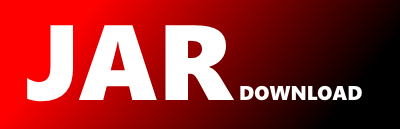
com.pulumi.random.kotlin.RandomIntegerArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.random.kotlin
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.random.RandomIntegerArgs.builder
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* The resource `random.RandomInteger` generates random values from a given range, described by the `min` and `max` attributes of a given resource.
* This resource can be used in conjunction with resources that have the `create_before_destroy` lifecycle flag set, to avoid conflicts with unique names during the brief period where both the old and new resources exist concurrently.
* ## Example Usage
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.random.RandomInteger;
* import com.pulumi.random.RandomIntegerArgs;
* import com.pulumi.aws.alb.ListenerRule;
* import com.pulumi.aws.alb.ListenerRuleArgs;
* import com.pulumi.aws.alb.inputs.ListenerRuleActionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var priority = new RandomInteger("priority", RandomIntegerArgs.builder()
* .min(1)
* .max(50000)
* .keepers(Map.of("listener_arn", var_.listener_arn()))
* .build());
* var main = new ListenerRule("main", ListenerRuleArgs.builder()
* .listenerArn(priority.keepers().applyValue(keepers -> keepers.listenerArn()))
* .priority(priority.result())
* .actions(ListenerRuleActionArgs.builder()
* .type("forward")
* .targetGroupArn(var_.target_group_arn())
* .build())
* .build());
* }
* }
* ```
* ## Import
* Random integers can be imported using the result, min, and max, with an optional seed. This can be used to replace a config value with a value interpolated from the random provider without experiencing diffs. Example (values are separated by a ,)
* ```sh
* $ pulumi import random:index/randomInteger:RandomInteger priority 15390,1,50000
* ```
* @property keepers Arbitrary map of values that, when changed, will trigger recreation of resource. See the main provider documentation for more information.
* @property max The maximum inclusive value of the range.
* @property min The minimum inclusive value of the range.
* @property seed A custom seed to always produce the same value.
*/
public data class RandomIntegerArgs(
public val keepers: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy