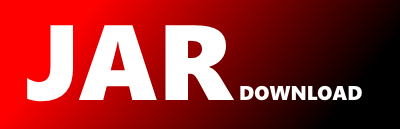
com.pulumi.random.kotlin.RandomShuffle.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.random.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
/**
* Builder for [RandomShuffle].
*/
@PulumiTagMarker
public class RandomShuffleResourceBuilder internal constructor() {
public var name: String? = null
public var args: RandomShuffleArgs = RandomShuffleArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend RandomShuffleArgsBuilder.() -> Unit) {
val builder = RandomShuffleArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.opts(block)
}
internal fun build(): RandomShuffle {
val builtJavaResource = com.pulumi.random.RandomShuffle(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return RandomShuffle(builtJavaResource)
}
}
/**
* The resource `random.RandomShuffle` generates a random permutation of a list of strings given as an argument.
* ## Example Usage
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.random.RandomShuffle;
* import com.pulumi.random.RandomShuffleArgs;
* import com.pulumi.aws.elb.LoadBalancer;
* import com.pulumi.aws.elb.LoadBalancerArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var az = new RandomShuffle("az", RandomShuffleArgs.builder()
* .inputs(
* "us-west-1a",
* "us-west-1c",
* "us-west-1d",
* "us-west-1e")
* .resultCount(2)
* .build());
* var example = new LoadBalancer("example", LoadBalancerArgs.builder()
* .availabilityZones(az.results())
* .build());
* }
* }
* ```
*/
public class RandomShuffle internal constructor(
override val javaResource: com.pulumi.random.RandomShuffle,
) : KotlinCustomResource(javaResource, RandomShuffleMapper) {
/**
* The list of strings to shuffle.
*/
public val inputs: Output>
get() = javaResource.inputs().applyValue({ args0 -> args0.map({ args0 -> args0 }) })
/**
* Arbitrary map of values that, when changed, will trigger recreation of resource. See the main provider documentation for more information.
*/
public val keepers: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy