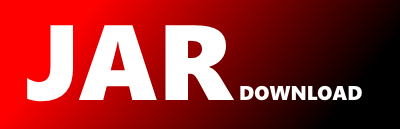
com.pulumi.vault.ad.kotlin.SecretBackend.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.ad.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [SecretBackend].
*/
@PulumiTagMarker
public class SecretBackendResourceBuilder internal constructor() {
public var name: String? = null
public var args: SecretBackendArgs = SecretBackendArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend SecretBackendArgsBuilder.() -> Unit) {
val builder = SecretBackendArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): SecretBackend {
val builtJavaResource = com.pulumi.vault.ad.SecretBackend(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return SecretBackend(builtJavaResource)
}
}
/**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const config = new vault.ad.SecretBackend("config", {
* backend: "ad",
* binddn: "CN=Administrator,CN=Users,DC=corp,DC=example,DC=net",
* bindpass: "SuperSecretPassw0rd",
* url: "ldaps://ad",
* insecureTls: true,
* userdn: "CN=Users,DC=corp,DC=example,DC=net",
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* config = vault.ad.SecretBackend("config",
* backend="ad",
* binddn="CN=Administrator,CN=Users,DC=corp,DC=example,DC=net",
* bindpass="SuperSecretPassw0rd",
* url="ldaps://ad",
* insecure_tls=True,
* userdn="CN=Users,DC=corp,DC=example,DC=net")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var config = new Vault.AD.SecretBackend("config", new()
* {
* Backend = "ad",
* Binddn = "CN=Administrator,CN=Users,DC=corp,DC=example,DC=net",
* Bindpass = "SuperSecretPassw0rd",
* Url = "ldaps://ad",
* InsecureTls = true,
* Userdn = "CN=Users,DC=corp,DC=example,DC=net",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/ad"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := ad.NewSecretBackend(ctx, "config", &ad.SecretBackendArgs{
* Backend: pulumi.String("ad"),
* Binddn: pulumi.String("CN=Administrator,CN=Users,DC=corp,DC=example,DC=net"),
* Bindpass: pulumi.String("SuperSecretPassw0rd"),
* Url: pulumi.String("ldaps://ad"),
* InsecureTls: pulumi.Bool(true),
* Userdn: pulumi.String("CN=Users,DC=corp,DC=example,DC=net"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.ad.SecretBackend;
* import com.pulumi.vault.ad.SecretBackendArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var config = new SecretBackend("config", SecretBackendArgs.builder()
* .backend("ad")
* .binddn("CN=Administrator,CN=Users,DC=corp,DC=example,DC=net")
* .bindpass("SuperSecretPassw0rd")
* .url("ldaps://ad")
* .insecureTls("true")
* .userdn("CN=Users,DC=corp,DC=example,DC=net")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* config:
* type: vault:ad:SecretBackend
* properties:
* backend: ad
* binddn: CN=Administrator,CN=Users,DC=corp,DC=example,DC=net
* bindpass: SuperSecretPassw0rd
* url: ldaps://ad
* insecureTls: 'true'
* userdn: CN=Users,DC=corp,DC=example,DC=net
* ```
*
* ## Import
* AD secret backend can be imported using the `backend`, e.g.
* ```sh
* $ pulumi import vault:ad/secretBackend:SecretBackend ad ad
* ```
*/
public class SecretBackend internal constructor(
override val javaResource: com.pulumi.vault.ad.SecretBackend,
) : KotlinCustomResource(javaResource, SecretBackendMapper) {
/**
* Use anonymous binds when performing LDAP group searches
* (if true the initial credentials will still be used for the initial connection test).
*/
public val anonymousGroupSearch: Output?
get() = javaResource.anonymousGroupSearch().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The unique path this backend should be mounted at. Must
* not begin or end with a `/`. Defaults to `ad`.
*/
public val backend: Output?
get() = javaResource.backend().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Distinguished name of object to bind when performing user and group search.
*/
public val binddn: Output
get() = javaResource.binddn().applyValue({ args0 -> args0 })
/**
* Password to use along with binddn when performing user search.
*/
public val bindpass: Output
get() = javaResource.bindpass().applyValue({ args0 -> args0 })
/**
* If set, user and group names assigned to policies within the
* backend will be case sensitive. Otherwise, names will be normalized to lower case.
*/
public val caseSensitiveNames: Output?
get() = javaResource.caseSensitiveNames().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* CA certificate to use when verifying LDAP server certificate, must be
* x509 PEM encoded.
*/
public val certificate: Output?
get() = javaResource.certificate().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Client certificate to provide to the LDAP server, must be x509 PEM encoded.
*/
public val clientTlsCert: Output?
get() = javaResource.clientTlsCert().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Client certificate key to provide to the LDAP server, must be x509 PEM encoded.
*/
public val clientTlsKey: Output?
get() = javaResource.clientTlsKey().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Default lease duration for secrets in seconds.
*/
public val defaultLeaseTtlSeconds: Output
get() = javaResource.defaultLeaseTtlSeconds().applyValue({ args0 -> args0 })
/**
* Denies an unauthenticated LDAP bind request if the user's password is empty;
* defaults to true.
*/
public val denyNullBind: Output?
get() = javaResource.denyNullBind().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Human-friendly description of the mount for the Active Directory backend.
*/
public val description: Output?
get() = javaResource.description().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* If set, opts out of mount migration on path updates.
* See here for more info on [Mount Migration](https://www.vaultproject.io/docs/concepts/mount-migration)
*/
public val disableRemount: Output?
get() = javaResource.disableRemount().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Use anonymous bind to discover the bind Distinguished Name of a user.
*/
public val discoverdn: Output?
get() = javaResource.discoverdn().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* LDAP attribute to follow on objects returned by in order to enumerate
* user group membership. Examples: `cn` or `memberOf`, etc. Defaults to `cn`.
*/
public val groupattr: Output?
get() = javaResource.groupattr().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* LDAP search base to use for group membership search (eg: ou=Groups,dc=example,dc=org).
*/
public val groupdn: Output?
get() = javaResource.groupdn().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Go template for querying group membership of user (optional) The template can access
* the following context variables: UserDN, Username. Defaults to `(|(memberUid={{.Username}})(member={{.UserDN}})(uniqueMember={{.UserDN}}))`
*/
public val groupfilter: Output?
get() = javaResource.groupfilter().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Skip LDAP server SSL Certificate verification. This is not recommended for production.
* Defaults to `false`.
*/
public val insecureTls: Output?
get() = javaResource.insecureTls().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The number of seconds after a Vault rotation where, if Active Directory
* shows a later rotation, it should be considered out-of-band
*/
public val lastRotationTolerance: Output
get() = javaResource.lastRotationTolerance().applyValue({ args0 -> args0 })
/**
* Mark the secrets engine as local-only. Local engines are not replicated or removed by
* replication.Tolerance duration to use when checking the last rotation time.
*/
public val local: Output?
get() = javaResource.local().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Maximum possible lease duration for secrets in seconds.
*/
public val maxLeaseTtlSeconds: Output
get() = javaResource.maxLeaseTtlSeconds().applyValue({ args0 -> args0 })
/**
* In seconds, the maximum password time-to-live.
*/
public val maxTtl: Output
get() = javaResource.maxTtl().applyValue({ args0 -> args0 })
/**
* The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
public val namespace: Output?
get() = javaResource.namespace().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Name of the password policy to use to generate passwords.
*/
public val passwordPolicy: Output?
get() = javaResource.passwordPolicy().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Timeout, in seconds, for the connection when making requests against the server
* before returning back an error.
*/
public val requestTimeout: Output?
get() = javaResource.requestTimeout().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Issue a StartTLS command after establishing unencrypted connection.
*/
public val starttls: Output
get() = javaResource.starttls().applyValue({ args0 -> args0 })
/**
* Maximum TLS version to use. Accepted values are `tls10`, `tls11`,
* `tls12` or `tls13`. Defaults to `tls12`.
*/
public val tlsMaxVersion: Output
get() = javaResource.tlsMaxVersion().applyValue({ args0 -> args0 })
/**
* Minimum TLS version to use. Accepted values are `tls10`, `tls11`,
* `tls12` or `tls13`. Defaults to `tls12`.
*/
public val tlsMinVersion: Output
get() = javaResource.tlsMinVersion().applyValue({ args0 -> args0 })
/**
* In seconds, the default password time-to-live.
*/
public val ttl: Output
get() = javaResource.ttl().applyValue({ args0 -> args0 })
/**
* Enables userPrincipalDomain login with [username]@UPNDomain.
*/
public val upndomain: Output
get() = javaResource.upndomain().applyValue({ args0 -> args0 })
/**
* LDAP URL to connect to. Multiple URLs can be specified by concatenating
* them with commas; they will be tried in-order. Defaults to `ldap://127.0.0.1`.
*/
public val url: Output?
get() = javaResource.url().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* In Vault 1.1.1 a fix for handling group CN values of
* different cases unfortunately introduced a regression that could cause previously defined groups
* to not be found due to a change in the resulting name. If set true, the pre-1.1.1 behavior for
* matching group CNs will be used. This is only needed in some upgrade scenarios for backwards
* compatibility. It is enabled by default if the config is upgraded but disabled by default on
* new configurations.
*/
public val usePre111GroupCnBehavior: Output
get() = javaResource.usePre111GroupCnBehavior().applyValue({ args0 -> args0 })
/**
* If true, use the Active Directory tokenGroups constructed attribute of the
* user to find the group memberships. This will find all security groups including nested ones.
*/
public val useTokenGroups: Output?
get() = javaResource.useTokenGroups().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Attribute used when searching users. Defaults to `cn`.
*/
public val userattr: Output?
get() = javaResource.userattr().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* LDAP domain to use for users (eg: ou=People,dc=example,dc=org)`.
*/
public val userdn: Output?
get() = javaResource.userdn().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
}
public object SecretBackendMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.vault.ad.SecretBackend::class == javaResource::class
override fun map(javaResource: Resource): SecretBackend = SecretBackend(
javaResource as
com.pulumi.vault.ad.SecretBackend,
)
}
/**
* @see [SecretBackend].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [SecretBackend].
*/
public suspend fun secretBackend(
name: String,
block: suspend SecretBackendResourceBuilder.() -> Unit,
): SecretBackend {
val builder = SecretBackendResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [SecretBackend].
* @param name The _unique_ name of the resulting resource.
*/
public fun secretBackend(name: String): SecretBackend {
val builder = SecretBackendResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy