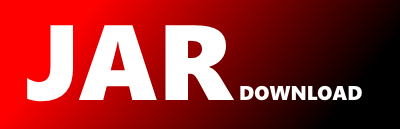
com.pulumi.vault.aws.kotlin.AuthBackendRole.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-vault-kotlin Show documentation
Show all versions of pulumi-vault-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.vault.aws.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
/**
* Builder for [AuthBackendRole].
*/
@PulumiTagMarker
public class AuthBackendRoleResourceBuilder internal constructor() {
public var name: String? = null
public var args: AuthBackendRoleArgs = AuthBackendRoleArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend AuthBackendRoleArgsBuilder.() -> Unit) {
val builder = AuthBackendRoleArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): AuthBackendRole {
val builtJavaResource = com.pulumi.vault.aws.AuthBackendRole(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return AuthBackendRole(builtJavaResource)
}
}
/**
* Manages an AWS auth backend role in a Vault server. Roles constrain the
* instances or principals that can perform the login operation against the
* backend. See the [Vault
* documentation](https://www.vaultproject.io/docs/auth/aws.html) for more
* information.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as vault from "@pulumi/vault";
* const aws = new vault.AuthBackend("aws", {type: "aws"});
* const example = new vault.aws.AuthBackendRole("example", {
* backend: aws.path,
* role: "test-role",
* authType: "iam",
* boundAmiIds: ["ami-8c1be5f6"],
* boundAccountIds: ["123456789012"],
* boundVpcIds: ["vpc-b61106d4"],
* boundSubnetIds: ["vpc-133128f1"],
* boundIamRoleArns: ["arn:aws:iam::123456789012:role/MyRole"],
* boundIamInstanceProfileArns: ["arn:aws:iam::123456789012:instance-profile/MyProfile"],
* inferredEntityType: "ec2_instance",
* inferredAwsRegion: "us-east-1",
* tokenTtl: 60,
* tokenMaxTtl: 120,
* tokenPolicies: [
* "default",
* "dev",
* "prod",
* ],
* });
* ```
* ```python
* import pulumi
* import pulumi_vault as vault
* aws = vault.AuthBackend("aws", type="aws")
* example = vault.aws.AuthBackendRole("example",
* backend=aws.path,
* role="test-role",
* auth_type="iam",
* bound_ami_ids=["ami-8c1be5f6"],
* bound_account_ids=["123456789012"],
* bound_vpc_ids=["vpc-b61106d4"],
* bound_subnet_ids=["vpc-133128f1"],
* bound_iam_role_arns=["arn:aws:iam::123456789012:role/MyRole"],
* bound_iam_instance_profile_arns=["arn:aws:iam::123456789012:instance-profile/MyProfile"],
* inferred_entity_type="ec2_instance",
* inferred_aws_region="us-east-1",
* token_ttl=60,
* token_max_ttl=120,
* token_policies=[
* "default",
* "dev",
* "prod",
* ])
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Vault = Pulumi.Vault;
* return await Deployment.RunAsync(() =>
* {
* var aws = new Vault.AuthBackend("aws", new()
* {
* Type = "aws",
* });
* var example = new Vault.Aws.AuthBackendRole("example", new()
* {
* Backend = aws.Path,
* Role = "test-role",
* AuthType = "iam",
* BoundAmiIds = new[]
* {
* "ami-8c1be5f6",
* },
* BoundAccountIds = new[]
* {
* "123456789012",
* },
* BoundVpcIds = new[]
* {
* "vpc-b61106d4",
* },
* BoundSubnetIds = new[]
* {
* "vpc-133128f1",
* },
* BoundIamRoleArns = new[]
* {
* "arn:aws:iam::123456789012:role/MyRole",
* },
* BoundIamInstanceProfileArns = new[]
* {
* "arn:aws:iam::123456789012:instance-profile/MyProfile",
* },
* InferredEntityType = "ec2_instance",
* InferredAwsRegion = "us-east-1",
* TokenTtl = 60,
* TokenMaxTtl = 120,
* TokenPolicies = new[]
* {
* "default",
* "dev",
* "prod",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault"
* "github.com/pulumi/pulumi-vault/sdk/v6/go/vault/aws"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* aws, err := vault.NewAuthBackend(ctx, "aws", &vault.AuthBackendArgs{
* Type: pulumi.String("aws"),
* })
* if err != nil {
* return err
* }
* _, err = aws.NewAuthBackendRole(ctx, "example", &aws.AuthBackendRoleArgs{
* Backend: aws.Path,
* Role: pulumi.String("test-role"),
* AuthType: pulumi.String("iam"),
* BoundAmiIds: pulumi.StringArray{
* pulumi.String("ami-8c1be5f6"),
* },
* BoundAccountIds: pulumi.StringArray{
* pulumi.String("123456789012"),
* },
* BoundVpcIds: pulumi.StringArray{
* pulumi.String("vpc-b61106d4"),
* },
* BoundSubnetIds: pulumi.StringArray{
* pulumi.String("vpc-133128f1"),
* },
* BoundIamRoleArns: pulumi.StringArray{
* pulumi.String("arn:aws:iam::123456789012:role/MyRole"),
* },
* BoundIamInstanceProfileArns: pulumi.StringArray{
* pulumi.String("arn:aws:iam::123456789012:instance-profile/MyProfile"),
* },
* InferredEntityType: pulumi.String("ec2_instance"),
* InferredAwsRegion: pulumi.String("us-east-1"),
* TokenTtl: pulumi.Int(60),
* TokenMaxTtl: pulumi.Int(120),
* TokenPolicies: pulumi.StringArray{
* pulumi.String("default"),
* pulumi.String("dev"),
* pulumi.String("prod"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.vault.AuthBackend;
* import com.pulumi.vault.AuthBackendArgs;
* import com.pulumi.vault.aws.AuthBackendRole;
* import com.pulumi.vault.aws.AuthBackendRoleArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var aws = new AuthBackend("aws", AuthBackendArgs.builder()
* .type("aws")
* .build());
* var example = new AuthBackendRole("example", AuthBackendRoleArgs.builder()
* .backend(aws.path())
* .role("test-role")
* .authType("iam")
* .boundAmiIds("ami-8c1be5f6")
* .boundAccountIds("123456789012")
* .boundVpcIds("vpc-b61106d4")
* .boundSubnetIds("vpc-133128f1")
* .boundIamRoleArns("arn:aws:iam::123456789012:role/MyRole")
* .boundIamInstanceProfileArns("arn:aws:iam::123456789012:instance-profile/MyProfile")
* .inferredEntityType("ec2_instance")
* .inferredAwsRegion("us-east-1")
* .tokenTtl(60)
* .tokenMaxTtl(120)
* .tokenPolicies(
* "default",
* "dev",
* "prod")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* aws:
* type: vault:AuthBackend
* properties:
* type: aws
* example:
* type: vault:aws:AuthBackendRole
* properties:
* backend: ${aws.path}
* role: test-role
* authType: iam
* boundAmiIds:
* - ami-8c1be5f6
* boundAccountIds:
* - '123456789012'
* boundVpcIds:
* - vpc-b61106d4
* boundSubnetIds:
* - vpc-133128f1
* boundIamRoleArns:
* - arn:aws:iam::123456789012:role/MyRole
* boundIamInstanceProfileArns:
* - arn:aws:iam::123456789012:instance-profile/MyProfile
* inferredEntityType: ec2_instance
* inferredAwsRegion: us-east-1
* tokenTtl: 60
* tokenMaxTtl: 120
* tokenPolicies:
* - default
* - dev
* - prod
* ```
*
* ## Import
* AWS auth backend roles can be imported using `auth/`, the `backend` path, `/role/`, and the `role` name e.g.
* ```sh
* $ pulumi import vault:aws/authBackendRole:AuthBackendRole example auth/aws/role/test-role
* ```
*/
public class AuthBackendRole internal constructor(
override val javaResource: com.pulumi.vault.aws.AuthBackendRole,
) : KotlinCustomResource(javaResource, AuthBackendRoleMapper) {
/**
* If set to `true`, allows migration of
* the underlying instance where the client resides.
*/
public val allowInstanceMigration: Output?
get() = javaResource.allowInstanceMigration().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The auth type permitted for this role. Valid choices
* are `ec2` and `iam`. Defaults to `iam`.
*/
public val authType: Output?
get() = javaResource.authType().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Path to the mounted aws auth backend.
*/
public val backend: Output?
get() = javaResource.backend().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* If set, defines a constraint on the EC2
* instances that can perform the login operation that they should be using the
* account ID specified by this field. `auth_type` must be set to `ec2` or
* `inferred_entity_type` must be set to `ec2_instance` to use this constraint.
*/
public val boundAccountIds: Output>?
get() = javaResource.boundAccountIds().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* If set, defines a constraint on the EC2 instances
* that can perform the login operation that they should be using the AMI ID
* specified by this field. `auth_type` must be set to `ec2` or
* `inferred_entity_type` must be set to `ec2_instance` to use this constraint.
*/
public val boundAmiIds: Output>?
get() = javaResource.boundAmiIds().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0
})
}).orElse(null)
})
/**
* Only EC2 instances that match this instance ID will be permitted to log in.
*/
public val boundEc2InstanceIds: Output>?
get() = javaResource.boundEc2InstanceIds().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* If set, defines a constraint on
* the EC2 instances that can perform the login operation that they must be
* associated with an IAM instance profile ARN which has a prefix that matches
* the value specified by this field. The value is prefix-matched as though it
* were a glob ending in `*`. `auth_type` must be set to `ec2` or
* `inferred_entity_type` must be set to `ec2_instance` to use this constraint.
*/
public val boundIamInstanceProfileArns: Output>?
get() = javaResource.boundIamInstanceProfileArns().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* If set, defines the IAM principal that
* must be authenticated when `auth_type` is set to `iam`. Wildcards are
* supported at the end of the ARN.
*/
public val boundIamPrincipalArns: Output>?
get() = javaResource.boundIamPrincipalArns().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* If set, defines a constraint on the EC2
* instances that can perform the login operation that they must match the IAM
* role ARN specified by this field. `auth_type` must be set to `ec2` or
* `inferred_entity_type` must be set to `ec2_instance` to use this constraint.
*/
public val boundIamRoleArns: Output>?
get() = javaResource.boundIamRoleArns().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* If set, defines a constraint on the EC2 instances
* that can perform the login operation that the region in their identity
* document must match the one specified by this field. `auth_type` must be set
* to `ec2` or `inferred_entity_type` must be set to `ec2_instance` to use this
* constraint.
*/
public val boundRegions: Output>?
get() = javaResource.boundRegions().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0
})
}).orElse(null)
})
/**
* If set, defines a constraint on the EC2
* instances that can perform the login operation that they be associated with
* the subnet ID that matches the value specified by this field. `auth_type`
* must be set to `ec2` or `inferred_entity_type` must be set to `ec2_instance`
* to use this constraint.
*/
public val boundSubnetIds: Output>?
get() = javaResource.boundSubnetIds().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* If set, defines a constraint on the EC2 instances
* that can perform the login operation that they be associated with the VPC ID
* that matches the value specified by this field. `auth_type` must be set to
* `ec2` or `inferred_entity_type` must be set to `ec2_instance` to use this
* constraint.
*/
public val boundVpcIds: Output>?
get() = javaResource.boundVpcIds().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0
})
}).orElse(null)
})
/**
* IF set to `true`, only allows a
* single token to be granted per instance ID. This can only be set when
* `auth_type` is set to `ec2`.
*/
public val disallowReauthentication: Output?
get() = javaResource.disallowReauthentication().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* When `inferred_entity_type` is set, this
* is the region to search for the inferred entities. Required if
* `inferred_entity_type` is set. This only applies when `auth_type` is set to
* `iam`.
*/
public val inferredAwsRegion: Output?
get() = javaResource.inferredAwsRegion().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* If set, instructs Vault to turn on
* inferencing. The only valid value is `ec2_instance`, which instructs Vault to
* infer that the role comes from an EC2 instance in an IAM instance profile.
* This only applies when `auth_type` is set to `iam`.
*/
public val inferredEntityType: Output?
get() = javaResource.inferredEntityType().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The namespace to provision the resource in.
* The value should not contain leading or trailing forward slashes.
* The `namespace` is always relative to the provider's configured [namespace](https://www.terraform.io/docs/providers/vault/index.html#namespace).
* *Available only for Vault Enterprise*.
*/
public val namespace: Output?
get() = javaResource.namespace().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Only valid when
* `auth_type` is `iam`. If set to `true`, the `bound_iam_principal_arns` are
* resolved to [AWS Unique
* IDs](http://docs.aws.amazon.com/IAM/latest/UserGuide/reference_identifiers.html#identifiers-unique-ids)
* for the bound principal ARN. This field is ignored when a
* `bound_iam_principal_arn` ends in a wildcard. Resolving to unique IDs more
* closely mimics the behavior of AWS services in that if an IAM user or role is
* deleted and a new one is recreated with the same name, those new users or
* roles won't get access to roles in Vault that were permissioned to the prior
* principals of the same name. Defaults to `true`.
* Once set to `true`, this cannot be changed to `false` without recreating the role.
*/
public val resolveAwsUniqueIds: Output?
get() = javaResource.resolveAwsUniqueIds().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The name of the role.
*/
public val role: Output
get() = javaResource.role().applyValue({ args0 -> args0 })
/**
* The Vault generated role ID.
*/
public val roleId: Output
get() = javaResource.roleId().applyValue({ args0 -> args0 })
/**
* If set, enable role tags for this role. The value set
* for this field should be the key of the tag on the EC2 instance. `auth_type`
* must be set to `ec2` or `inferred_entity_type` must be set to `ec2_instance`
* to use this constraint.
*/
public val roleTag: Output?
get() = javaResource.roleTag().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Specifies the blocks of IP addresses which are allowed to use the generated token
*/
public val tokenBoundCidrs: Output>?
get() = javaResource.tokenBoundCidrs().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* Generated Token's Explicit Maximum TTL in seconds
*/
public val tokenExplicitMaxTtl: Output?
get() = javaResource.tokenExplicitMaxTtl().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The maximum lifetime of the generated token
*/
public val tokenMaxTtl: Output?
get() = javaResource.tokenMaxTtl().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* If true, the 'default' policy will not automatically be added to generated tokens
*/
public val tokenNoDefaultPolicy: Output?
get() = javaResource.tokenNoDefaultPolicy().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The maximum number of times a token may be used, a value of zero means unlimited
*/
public val tokenNumUses: Output?
get() = javaResource.tokenNumUses().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Generated Token's Period
*/
public val tokenPeriod: Output?
get() = javaResource.tokenPeriod().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* Generated Token's Policies
*/
public val tokenPolicies: Output>?
get() = javaResource.tokenPolicies().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* The initial ttl of the token to generate in seconds
*/
public val tokenTtl: Output?
get() = javaResource.tokenTtl().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The type of token to generate, service or batch
*/
public val tokenType: Output?
get() = javaResource.tokenType().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
}
public object AuthBackendRoleMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.vault.aws.AuthBackendRole::class == javaResource::class
override fun map(javaResource: Resource): AuthBackendRole = AuthBackendRole(
javaResource as
com.pulumi.vault.aws.AuthBackendRole,
)
}
/**
* @see [AuthBackendRole].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [AuthBackendRole].
*/
public suspend fun authBackendRole(
name: String,
block: suspend AuthBackendRoleResourceBuilder.() -> Unit,
): AuthBackendRole {
val builder = AuthBackendRoleResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [AuthBackendRole].
* @param name The _unique_ name of the resulting resource.
*/
public fun authBackendRole(name: String): AuthBackendRole {
val builder = AuthBackendRoleResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy